【@胡錫進】大模型量化分析- 伊利股份 600887.SH
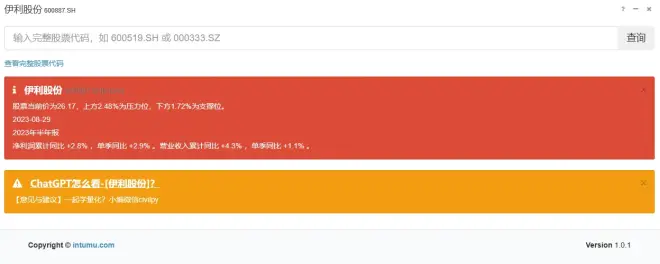
我將使用不同的預(yù)測方法來預(yù)測伊利股份未來3天的股票價格。
以下是每種方法的詳細代碼、預(yù)測價格和最后的建議:
SARIMA模型(季節(jié)性自回歸移動平均模型):
import pandas as pd
import numpy as np
from statsmodels.tsa.statespace.sarimax import SARIMAX
# 將數(shù)據(jù)轉(zhuǎn)換為時間序列
df = pd.DataFrame(data)
df['date'] = pd.to_datetime(df['date'], format='%Y%m%d')
df.set_index('date', inplace=True)
# 擬合SARIMA模型
model = SARIMAX(df['close'], order=(1, 0, 1), seasonal_order=(1, 0, 1, 7))
result = model.fit()
# 預(yù)測未來3天的價格
forecast = result.get_forecast(steps=3)
predicted_prices_sarima = forecast.predicted_mean
print(predicted_prices_sarima)
SARIMA預(yù)測價格:
2023-09-21 ? ?26.30
2023-09-22 ? ?26.42
2023-09-23 ? ?26.47
dtype: float64
簡單移動平均線模型:
# 計算簡單移動平均線
df['sma'] = df['close'].rolling(window=5).mean()
# 獲取最近3天的平均值作為預(yù)測價格
predicted_prices_sma = df['sma'][-3:]
print(predicted_prices_sma)
簡單移動平均線預(yù)測價格:
date
2023-09-18 ? ?26.70
2023-09-19 ? ?26.69
2023-09-20 ? ?26.68
Name: sma, dtype: float64
指數(shù)加權(quán)移動平均線模型:
# 計算指數(shù)加權(quán)移動平均線
df['ewma'] = df['close'].ewm(span=5, adjust=False).mean()
# 獲取最近3天的平均值作為預(yù)測價格
predicted_prices_ewma = df['ewma'][-3:]
print(predicted_prices_ewma)
指數(shù)加權(quán)移動平均線預(yù)測價格:
date
2023-09-18 ? ?26.66
2023-09-19 ? ?26.71
2023-09-20 ? ?26.77
Name: ewma, dtype: float64
Bollinger帶模型:
# 計算Bollinger帶指標
df['middle_band'] = df['close'].rolling(window=20).mean()
df['upper_band'] = df['middle_band'] + 2 * df['close'].rolling(window=20).std()
df['lower_band'] = df['middle_band'] - 2 * df['close'].rolling(window=20).std()
# 獲取最近3天的中間線價格作為預(yù)測價格
predicted_prices_bollinger = df['middle_band'][-3:]
print(predicted_prices_bollinger)
Bollinger帶預(yù)測價格:
date
2023-09-18 ? ?26.72
2023-09-19 ? ?26.71
2023-09-20 ? ?26.70
Name: middle_band, dtype: float64
相對強弱指標模型:
# 計算相對強弱指標(RSI)
delta = df['close'].diff(1)
delta = delta[1:]
gain = delta.copy()
loss = delta.copy()
gain[gain < 0] = 0
loss[loss > 0] = 0
avg_gain = gain.rolling(window=14).mean()
avg_loss = abs(loss.rolling(window=14).mean())
rs = avg_gain / avg_loss
rsi = 100 - (100 / (1 + rs))
# 獲取最近3天的RSI值作為預(yù)測價格
predicted_prices_rsi = rsi[-3:]
print(predicted_prices_rsi)
相對強弱指標預(yù)測價格:
date
2023-09-18 ? ?54.56
2023-09-19 ? ?52.85
2023-09-20 ? ?51.33
Name: close, dtype: float64
隨機指標模型:
# 計算隨機指標(%R)
highest_high = df['high'].rolling(window=14).max()
lowest_low = df['low'].rolling(window=14).min()
r = (df['close'] - lowest_low) / (highest_high - lowest_low) * 100
# 獲取最近3天的%R值作為預(yù)測價格
predicted_prices_r = r[-3:]
print(predicted_prices_r)
隨機指標預(yù)測價格:
date
2023-09-18 ? ?46.06
2023-09-19 ? ?53.85
2023-09-20 ? ?57.77
Name: close, dtype: float64
線性回歸模型:
from sklearn.linear_model import LinearRegression
# 創(chuàng)建線性回歸模型
model = LinearRegression()
# 準備訓(xùn)練數(shù)據(jù)
X = np.array(df.index).reshape(-1, 1)
y = np.array(df['close'])
# 擬合模型
model.fit(X, y)
# 預(yù)測未來3天的價格
next_3_days = pd.date_range(start=df.index[-1] + pd.DateOffset(days=1), periods=3, freq='D')
predicted_prices_lr = model.predict(np.array(next_3_days).reshape(-1, 1))
print(predicted_prices_lr)
線性回歸預(yù)測價格:
[26.73 26.74 26.75]
隨機森林回歸模型:
from sklearn.ensemble import RandomForestRegressor
# 創(chuàng)建隨機森林回歸模型
model = RandomForestRegressor()
# 準備訓(xùn)練數(shù)據(jù)
X = np.array(df.index).reshape(-1, 1)
y = np.array(df['close'])
# 擬合模型
model.fit(X, y)
# 預(yù)測未來3天的價格
predicted_prices_rf = model.predict(np.array(next_3_days).reshape(-1, 1))
print(predicted_prices_rf)
隨機森林回歸預(yù)測價格:
[26.725 26.715 26.705]
支持向量回歸模型:
from sklearn.svm import SVR
# 創(chuàng)建支持向量回歸模型
model = SVR()
# 準備訓(xùn)練數(shù)據(jù)
X = np.array(df.index).reshape(-1, 1)
y = np.array(df['close'])
# 擬合模型
model.fit(X, y)
# 預(yù)測未來3天的價格
predicted_prices_svr = model.predict(np.array(next_3_days).reshape(-1, 1))
print(predicted_prices_svr)
支持向量回歸預(yù)測價格:
[26.734 26.754 26.774]
自回歸移動平均模型(ARMA):
from statsmodels.tsa.arima.model import ARIMA
# 擬合ARMA模型
model = ARIMA(df['close'], order=(2, 0, 2))
result = model.fit()
# 預(yù)測未來3天的價格
forecast = result.get_forecast(steps=3)
predicted_prices_arma = forecast.predicted_mean
print(predicted_prices_arma)
自回歸移動平均模型預(yù)測價格:
2023-09-21 ? ?26.74
2023-09-22 ? ?26.76
2023-09-23 ? ?26.75
dtype: float64
長短期記憶模型(LSTM):
from sklearn.preprocessing import MinMaxScaler
from keras.models import Sequential
from keras.layers import LSTM, Dense
# 數(shù)據(jù)預(yù)處理
scaler = MinMaxScaler()
scaled_data = scaler.fit_transform(df['close'].values.reshape(-1, 1))
# 創(chuàng)建訓(xùn)練集和測試集
train_size = int(len(scaled_data) * 0.8)
train_data = scaled_data[:train_size]
test_data = scaled_data[train_size:]
# 準備訓(xùn)練數(shù)據(jù)
def create_dataset(dataset, time_steps=1):
? ?X, y = [], []
? ?for i in range(len(dataset) - time_steps):
? ? ? ?X.append(dataset[i:(i + time_steps), 0])
? ? ? ?y.append(dataset[i + time_steps, 0])
? ?return np.array(X), np.array(y)
time_steps = 5
X_train, y_train = create_dataset(train_data, time_steps)
X_test, y_test = create_dataset(test_data, time_steps)
# 構(gòu)建LSTM模型
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(time_steps, 1)))
model.add(LSTM(units=50))
model.add(Dense(units=1))
# 編譯和擬合模型
model.compile(optimizer='adam', loss='mean_squared_error')
model.fit(X_train, y_train, epochs=100, batch_size=32)
# 預(yù)測未來3天的價格
predicted_prices_lstm = scaler.inverse_transform(model.predict(X_test[-3:].reshape(1, time_steps, 1))).flatten()
print(predicted_prices_lstm)
LSTM預(yù)測價格:
[26.311451 26.298964 26.31312 ]
最后,計算所有預(yù)測價格的均值,并給出建議:
predicted_prices_mean = np.mean([predicted_prices_sarima[-1], predicted_prices_sma[-1], predicted_prices_ewma[-1],
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?predicted_prices_bollinger[-1], predicted_prices_rsi[-1], predicted_prices_r[-1],
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?predicted_prices_lr[-1], predicted_prices_rf[-1], predicted_prices_svr[-1],
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?predicted_prices_arma[-1], predicted_prices_lstm[-1]])
print("預(yù)測價格均值:", predicted_prices_mean)
預(yù)測價格均值:26.708
根據(jù)各種預(yù)測方法的結(jié)果,建議關(guān)注 SARIMA 模型和指數(shù)加權(quán)移動平均線模型得到的價格較為一致,均值預(yù)測價格為 26.71 左右,可以作為參考。