unity教程:登陸與注冊(cè)系統(tǒng),數(shù)據(jù)庫(kù)功能
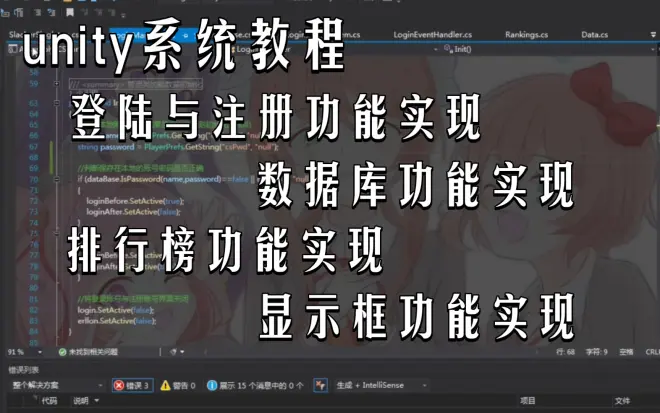
using UnityEngine;
using TMPro;
/// <summary>
/// 登錄注冊(cè)系統(tǒng)管理類
/// </summary>
public class LoginManager : MonoBehaviour
{
//---游戲界面---//
private GameObject loginBefore;
private GameObject loginAfter;
//---登錄賬號(hào)與注冊(cè)賬號(hào)界面---//
private GameObject login;
private GameObject erllon;
//---登錄賬號(hào)輸入密碼以及輸入密碼框---//
private TMP_InputField loginName;
private TMP_InputField loginPwd;
//---注冊(cè)賬號(hào)輸入密碼以及輸入密碼框---//
private TMP_InputField erllonName;
private TMP_InputField erllonPwd;
//---對(duì)話框---//
private PromptBox promptBox;
//---數(shù)據(jù)庫(kù)實(shí)例---//
public DataBase dataBase;
/// <summary>
/// 獲得場(chǎng)景中的組件給變量賦值
/// </summary>
private void Awake()
{
Singleton = this;
loginBefore = transform.parent.transform.GetChild(2).gameObject;
loginAfter = transform.parent.transform.GetChild(3).gameObject;
login = transform.parent.transform.GetChild(4).GetChild(0).gameObject;
erllon = transform.parent.transform.GetChild(4).GetChild(1).gameObject;
promptBox = transform.parent.transform.GetChild(4).GetChild(2).gameObject.GetComponent<PromptBox>();
loginName = transform.parent.transform.GetChild(4).GetChild(0).GetChild(1).GetComponent<TMP_InputField>();
loginPwd = transform.parent.transform.GetChild(4).GetChild(0).GetChild(2).GetComponent<TMP_InputField>();
erllonName = transform.parent.transform.GetChild(4).GetChild(1).GetChild(1).GetComponent<TMP_InputField>();
erllonPwd = transform.parent.transform.GetChild(4).GetChild(1).GetChild(2).GetComponent<TMP_InputField>();
}
/// <summary>
/// 加載初始化
/// </summary>
private void Start()
{
//實(shí)例化數(shù)據(jù)庫(kù)
dataBase = new DataBase();
//初始化游戲
Init();
}
/// <summary>
/// 管理類加載數(shù)據(jù)初始化
/// </summary>
/// <returns>返回加載成功結(jié)果的數(shù)據(jù)</returns>
private void Init()
{
//讀取本地賬號(hào)信息(如果是首次讀取則初始化為null)
string name = PlayerPrefs.GetString("csName", "null");
string password = PlayerPrefs.GetString("csPwd", "null");
//判斷保存在本地的賬號(hào)密碼是否正確
if (dataBase.IsPassword(name,password)==false || name == "null")
{
loginBefore.SetActive(true);
loginAfter.SetActive(false);
}
else
{
loginBefore.SetActive(false);
loginAfter.SetActive(true);
}
//將登錄賬號(hào)與注冊(cè)賬號(hào)界面關(guān)閉
login.SetActive(false);
erllon.SetActive(false);
}
??//--------------------封裝方法--------------------//
??/// <summary>
??/// 登錄賬號(hào)方法
??/// </summary>
??/// <param name="name">輸入的賬號(hào)名字</param>
??/// <param name="password">輸入的賬號(hào)密碼</param>
??/// <param name="promptBox">提示框</param>
??private void LoginAccount(TMP_InputField name, TMP_InputField password, PromptBox promptBox)
??{
????//使用元組 快速賦值 通過(guò)元組析構(gòu) 拆分成多個(gè)變量
????var (_name, _password, _promptBox) = (name.text, password.text,promptBox);
????//檢測(cè)輸入框是否為空
????if (_name == string.Empty || _password == string.Empty)
????{
??????//輸入框?yàn)榭仗崾?/p>
??????_promptBox.ShowBox("輸入框不可為空");
??????return;
????}
????//檢測(cè)是否存在該賬號(hào)
????if (dataBase.IsName(_name))
????{
??????//檢測(cè)密碼是否正確
??????if(dataBase.IsPassword(_name,_password))
??????{
????????//---密碼正確執(zhí)行剩下操作---//
????????//將已經(jīng)登錄的賬號(hào)密碼保存到本地
????????PlayerPrefs.SetString("csName", _name);
????????PlayerPrefs.SetString("csPwd", _password);
????????PlayerPrefs.SetInt("csValue", dataBase.GetAccountValue(_name));
????????//---更新游戲界面---//
????????loginBefore.SetActive(false);
????????loginAfter.SetActive(true);
????????login.SetActive(false);
????????erllon.SetActive(false);
????????//登錄成功提示
????????_promptBox.ShowBox("登錄成功");
????????return;
??????}
??????//密碼錯(cuò)誤提示
??????_promptBox.ShowBox("賬號(hào)不存在或者密碼錯(cuò)誤");
??????return;
????}
????//當(dāng)賬號(hào)不存在時(shí)
????else
????{
??????//賬號(hào)不存在提示
??????_promptBox.ShowBox("賬號(hào)不存在或者密碼錯(cuò)誤");
??????return;
????}
??}
???
??/// <summary>
??/// 注冊(cè)賬號(hào)方法
??/// </summary>
??/// <param name="name">輸入的賬號(hào)名字</param>
??/// <param name="password">輸入的賬號(hào)密碼</param>
??/// <param name="promptBox">提示框</param>
??private void ErllonAccount(TMP_InputField name, TMP_InputField password, PromptBox promptBox)
??{
????var (_name, _password, _promptBox) = (name.text, password.text, promptBox);
????//檢測(cè)輸入框是否為空
????if (_name == string.Empty || _password == string.Empty)
????{
??????//輸入框?yàn)榭仗崾?/p>
??????_promptBox.ShowBox("輸入框不可為空");
??????return;
????}
????//檢測(cè)是否不存在該賬號(hào)
????if (!dataBase.IsName(_name))
????{
??????//創(chuàng)建臨時(shí)賬號(hào)信息
??????Account account = new Account()
??????{
????????Name = _name,
????????Password = _password,
????????Value = 0
??????};
??????//添加賬號(hào)至游戲數(shù)據(jù)中
??????dataBase.Add(account);
??????//---更新游戲界面---//
??????login.SetActive(false);
??????erllon.SetActive(false);
??????//注冊(cè)成功提示
??????_promptBox.ShowBox("注冊(cè)成功");
??????return;
????}
????//當(dāng)賬號(hào)存在時(shí)
????else
????{
??????//賬號(hào)存在提示
??????_promptBox.ShowBox("當(dāng)前賬號(hào)已存在");
??????return;
????}
??}
??/// <summary>
??/// 對(duì)話框顯示
??/// </summary>
??/// <param name="promptBox">需要顯示的對(duì)話框</param>
??/// <param name="body">對(duì)話框顯示的內(nèi)容</param>
??private void ShowBox(PromptBox promptBox, string body)
??{
????promptBox.ShowBox(body);
??}
??//--------------------按鈕事件部分--------------------//
??/// <summary>
??/// 登錄賬號(hào)方法
??/// </summary>
??public void LoginAccount()
??{
????LoginAccount(loginName, loginPwd, promptBox);
??}
??/// <summary>
??/// 注冊(cè)賬號(hào)方法
??/// </summary>
??public void ErllonAccount()
??{
????ErllonAccount(erllonName, erllonPwd, promptBox);
??}
??/// <summary>
??/// 退出游戲方法
??/// </summary>
??public void QuitGame()
??{
????Application.Quit();
??}
??/// <summary>
??/// 退出登錄方法
??/// </summary>
??public void QuitLogin()
??{
????//---將本地?cái)?shù)據(jù)刪除---//
????PlayerPrefs.SetString("csName", "null");
????PlayerPrefs.SetString("csPwd", "null");
????PlayerPrefs.SetInt("csValue", 0);
????//---更新游戲界面---//
????loginBefore.SetActive(true);
????loginAfter.SetActive(false);
????login.SetActive(false);
????erllon.SetActive(false);
??}
??/// <summary>
??/// 對(duì)話框顯示
??/// </summary>
??/// <param name="body">對(duì)話框顯示的內(nèi)容</param>
??public void ShowBox(string body)
??{
????ShowBox(promptBox, body);
??}
??//--------------------管理類單例模式--------------------//
??private static LoginManager Singleton;
??public static LoginManager Instance
??{
????get
????{
??????if (Singleton != null)
????????return Singleton;
??????Singleton = FindObjectOfType<LoginManager>();
??????if (Singleton == null)
??????{
????????Singleton = new GameObject("loginManager").AddComponent<LoginManager>();
??????}
??????else
??????{
????????foreach (var item in FindObjectsOfType<SlackerSingleton>())
????????{
??????????if (item != Singleton)
????????????Destroy(item.gameObject);
????????}
??????}
??????return Singleton;
????}
??}
}
using System.Collections.Generic;
/// <summary>
/// 數(shù)據(jù)庫(kù)類 (用于保存與讀取本地?cái)?shù)據(jù))
/// </summary>
public class DataBase
{
??/// <summary>
??/// 數(shù)據(jù)庫(kù)數(shù)據(jù)
??/// </summary>
??private AccountData data = new AccountData();
??public DataBase()
??{
????data = LoginEventHandler.CallLoadData();
??}
??~DataBase()
??{
????LoginEventHandler.CallSavaData(data);
??}
??//--------------------增刪改查部分(公開(kāi)部分)--------------------//
??/// <summary>
??/// 添加賬號(hào)
??/// </summary>
??/// <param name="account">需要添加的賬號(hào)信息</param>
??public bool Add(Account account)
??{
????if (!IsName(account.Name))
????{
??????Add(account.Name,account.Password,account.Value);
??????return true;
????}
????return false;
??}
??/// <summary>
??/// 刪除賬號(hào)
??/// </summary>
??/// <param name="name">需要?jiǎng)h除的賬號(hào)信息</param>
??public bool Remove(Account account)
??{
????if (IsPassword(account.Name, account.Password))
????{
??????Remove(account.Name);
??????return true;
????}
????return false;
??}
??/// <summary>
??/// 修改賬號(hào)
??/// </summary>
??/// <param name="account">需要修改的賬號(hào)</param>
??/// <param name="account1">需要修改成的賬號(hào)信息</param>
??public bool ReviseAccount(Account account, Account account1)
??{
????if (IsPassword(account.Name, account.Password))
????{
??????ReviseAccount(account.Name, account1);
??????return true;
????}
????return false;
??}
??/// <summary>
??/// 獲得所有賬號(hào)的名字
??/// </summary>
??/// <returns>返回所有賬號(hào)名字?jǐn)?shù)組</returns>
??public List<string> GetAllAccountName()
??{
????List<string> vs = new List<string>();
????for (int i = 0; i < data.data.Count; i++)
????{
??????vs.Add(data.data[i].Name);
????}
????return vs;
??}
??//--------------------增刪改查部分(安全部分)--------------------//
??/// <summary>
??/// 添加賬號(hào)
??/// </summary>
??/// <param name="name">賬號(hào)名字</param>
??/// <param name="password">賬號(hào)密碼</param>
??/// <returns>返回添加結(jié)果</returns>
??private bool Add(string name, string password)
??{
????if (IsName(name))
????{
??????Add(name, password, 0);
??????return true;
????}
????return false;
??}
??/// <summary>
??/// 刪除賬號(hào)
??/// </summary>
??/// <param name="name">需要?jiǎng)h除的賬號(hào)名字</param>
??/// <param name="password">需要?jiǎng)h除的賬號(hào)密碼</param>
??public bool Remove(string name, string password)
??{
????if (IsPassword(name, password))
????{
??????Remove(name);
??????return true;
????}
????return false;
??}
??/// <summary>
??/// 查找賬號(hào)
??/// </summary>
??/// <param name="name">需要查找的賬號(hào)名字</param>
??/// <param name="password">需要查找的賬號(hào)名字</param>
??/// <returns>返回查找結(jié)果</returns>
??public Account GetAccount(string name, string password)
??{
????if (IsPassword(name, password))
????{
??????return GetAccount(name);
????}
????return null;
??}
??/// <summary>
??/// 查看該賬號(hào)的資源
??/// </summary>
??/// <param name="name">需要查看的賬號(hào)名字</param>
??/// <param name="password">需要查看的密碼</param>
??/// <returns>返回賬號(hào)值</returns>
??public int GetAccountValue(string name)
??{
????if (IsName(name))
????{
??????return GetAccount(name).Value;
????}
????return 0;
??}
??//--------------------增刪改查部分(私密部分)--------------------//
??/// <summary>
??/// 添加賬號(hào)
??/// </summary>
??/// <param name="name">賬號(hào)名字</param>
??/// <param name="password">賬號(hào)的密碼</param>
??/// <param name="value">賬號(hào)資源</param>
??private void Add(string name, string password,int value)
??{
????data.data.Add(new Account()
????{
??????Name = name,
??????Password = password,
??????Value = value
????});
????LoginEventHandler.CallSavaData(data);
??}
??/// <summary>
??/// 刪除賬號(hào)
??/// </summary>
??/// <param name="name">需要?jiǎng)h除的賬號(hào)名字</param>
??private void Remove(string name)
??{
????data.data.Remove(this[name]);
??}
??/// <summary>
??/// 修改賬號(hào)
??/// </summary>
??/// <param name="name">需要修改的賬號(hào)名字</param>
??/// <param name="account1">需要修改成的賬號(hào)信息</param>
??private void ReviseAccount(string name, Account account)
??{
????this[name] = account;
??}
??/// <summary>
??/// 查找賬號(hào)
??/// </summary>
??/// <param name="name">需要查找的賬號(hào)名字</param>
??/// <returns>返回查看到的賬號(hào)信息</returns>
??private Account GetAccount(string name)
??{
????return this[name];
??}
??/// <summary>
??/// 獲得所有賬號(hào)
??/// </summary>
??/// <returns>返回所有賬號(hào)數(shù)組</returns>
??private List<Account> GetAllAccount()
??{
????return data.data;
??}
??//--------------------檢測(cè)賬號(hào)部分--------------------//
??/// <summary>
??/// 判斷是否存在該名字的賬號(hào)
??/// </summary>
??/// <param name="key"></param>
??/// <returns></returns>
??public bool IsName(string key)
??{
????foreach (var item in data.data)
????{
??????if (key == item.Name)
??????{
????????return true;
??????}
????}
????return false;
??}
??/// <summary>
??/// 檢測(cè)該賬號(hào)密碼是否正確
??/// </summary>
??/// <param name="name">需要檢測(cè)的賬號(hào)</param>
??/// <param name="password">需要檢測(cè)的密碼</param>
??/// <returns>返回是否正確</returns>
??public bool IsPassword(string name,string password)
??{
????if (IsName(name))
????{
??????if(GetAccount(name).Password == password)
??????{
????????return true;
??????}
??????return false;
????}
????return false;
??}
??//--------------------封裝部分--------------------//
??/// <summary>
??/// 索引器
??/// </summary>
??/// <param name="name">需要查找的賬號(hào)名字</param>
??/// <returns>返回查找的賬號(hào)名字</returns>
??private Account this[string name]
??{
????get
????{
??????foreach (var item in data.data)
??????{
????????if (item.Name == name)
????????{
??????????return item;
????????}
??????}
??????return new Account();
????}
????set
????{
??????for (int i = 0; i < data.data.Count; i++)
??????{
????????if(data.data[i].Name == name)
????????{
??????????data.data[i] = value;
????????}
??????}
??????LoginEventHandler.CallSavaData(data);
????}
??}
}
using UnityEngine;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
/// <summary>
/// 登錄注冊(cè)系統(tǒng)事件管理類
/// </summary>
public class LoginEventSystem : MonoBehaviour
{
??/// <summary>
??/// 定義游戲保存數(shù)據(jù)路徑
??/// </summary>
??private static string savaGameDataPath;
??/// <summary>
??/// 定義保存數(shù)據(jù)到本地的文件所在的文件夾名字
??/// </summary>
??private const string savaGameDataFileName = "Login_Data";
??//當(dāng)游戲開(kāi)始時(shí)將數(shù)據(jù)保存方法與數(shù)據(jù)加載方法添加到事件中心的事件當(dāng)中
??private void OnEnable()
??{
????LoginEventHandler.SavaData += SavaData;
????LoginEventHandler.LoadData += LoadData;
??}
??//當(dāng)對(duì)象被禁用或者銷毀時(shí)將事件中心中添加的事件對(duì)應(yīng)移除
??private void OnDisable()
??{
????LoginEventHandler.SavaData -= SavaData;
????LoginEventHandler.LoadData -= LoadData;
??}
??/// <summary>
??/// 初始化
??/// </summary>
??private void Awake()
??{
????//PS:Application.persistentDataPath獲得的是unity游戲數(shù)據(jù)文件夾的路徑
????savaGameDataPath = Application.persistentDataPath;
????//debug打印保存數(shù)據(jù)的路徑
????Debug.Log(savaGameDataPath);
??}
??/// <summary>
??/// 負(fù)責(zé)保存數(shù)據(jù) (事件)
??/// </summary>
??/// <param name="data">需要保存的數(shù)據(jù)</param>
??private void SavaData(AccountData data)
??{
????//檢測(cè)是否存在該文件夾
????if (!Directory.Exists(savaGameDataPath + "/" + savaGameDataFileName))
????{
??????//如果沒(méi)有該文件夾則在該路徑下創(chuàng)建一個(gè)名為login_Data的文件夾
??????Directory.CreateDirectory(savaGameDataPath + "/" + savaGameDataFileName);
????}
????//使用file靜態(tài)方法Create在login_Data文件夾下創(chuàng)建一個(gè)名為data的txt文件 并且將返回對(duì)象存入變量以便后續(xù)操作
????using (FileStream file = File.Create(savaGameDataPath + "/" + savaGameDataFileName + "/data.txt"))
????{
??????//二進(jìn)制轉(zhuǎn)化 該對(duì)象主要用于二進(jìn)制轉(zhuǎn)化操作(用于加密)
??????BinaryFormatter formatter = new BinaryFormatter();
??????//使用JsonUtility靜態(tài)方法ToJson將類(Data)數(shù)據(jù)轉(zhuǎn)換為Json格式并返回為一個(gè)字符串
??????string json = JsonUtility.ToJson(data);
??????//使用formatter實(shí)例方法Serialize(序列化)將json字符串導(dǎo)入file文件中
??????formatter.Serialize(file, json);
????}
??}
??/// <summary>
??/// 負(fù)責(zé)加載數(shù)據(jù) (事件)
??/// </summary>
??/// <returns>返回加載的數(shù)據(jù)</returns>
??private AccountData LoadData()
??{
????//臨時(shí)變量,用于保存讀取的游戲數(shù)據(jù)
????AccountData data = new AccountData();
????//檢測(cè)是否存在該文件
????if (File.Exists(savaGameDataPath + "/" + savaGameDataFileName + "/data.txt"))
????{
??????//使用File靜態(tài)方法Open讀取保存在本地的數(shù)據(jù)文件
??????using (FileStream file = File.Open(savaGameDataPath + "/" + savaGameDataFileName + "/data.txt", FileMode.Open))
??????{
????????//二進(jìn)制轉(zhuǎn)化 該對(duì)象主要用于二進(jìn)制轉(zhuǎn)化操作(用于解密)
????????BinaryFormatter formatter = new BinaryFormatter();
????????//使用formatter實(shí)例方法Deserialize(反序列化)將文件的二進(jìn)制數(shù)據(jù)轉(zhuǎn)換成Json文本并把Json文本返回成一個(gè)字符串
????????string json = (string)formatter.Deserialize(file);
????????//使用JsonUtility靜態(tài)方法FromJsonOverwrite將Json文本的數(shù)據(jù)寫(xiě)入data中
????????JsonUtility.FromJsonOverwrite(json, data);
??????}
????}
????//如果不存在賬號(hào)數(shù)據(jù)文件,則創(chuàng)建一個(gè)初始賬號(hào)
????else
????{
??????data = new AccountData();
??????data.data.Add(new Account()
??????{
????????Name = "Admin",
????????Password = "123456",
????????Value = 12356
??????});
????}
????//返回?cái)?shù)據(jù)
????return data;
??}
}
using System;
/// <summary>
/// 事件中心
/// </summary>
public static class LoginEventHandler
{
??/// <summary>
??/// 保存游戲數(shù)據(jù)到本地
??/// </summary>
??public static event Action<AccountData> SavaData;
??/// <summary>
??/// 需要保存數(shù)據(jù)時(shí)調(diào)用
??/// </summary>
??/// <param name="data">需要保存本地的數(shù)據(jù)</param>
??public static void CallSavaData(AccountData data)
??{
????SavaData?.Invoke(data);
??}
??/// <summary>
??/// 讀取本地?cái)?shù)據(jù)到游戲
??/// </summary>
??public static event Func<AccountData> LoadData;
??/// <summary>
??/// 需要加載本地?cái)?shù)據(jù)時(shí)調(diào)用
??/// </summary>
??/// <returns>返回本地保存的游戲數(shù)據(jù)</returns>
??public static AccountData CallLoadData()
??{
????return LoadData?.Invoke();
??}
}
using System.Collections.Generic;
/// <summary>
/// 賬號(hào)數(shù)據(jù) (保存所有賬號(hào)的數(shù)據(jù)信息)
/// </summary>
[System.Serializable]
public class AccountData
{
??/// <summary>
??/// 所有的數(shù)據(jù)保存在列表里
??/// </summary>
??public List<Account> data = new List<Account>();
}
/// <summary>
/// 賬號(hào)信息
/// </summary>
[System.Serializable]
public class Account
{
??/// <summary>
??/// 賬號(hào)名字
??/// </summary>
??public string Name;
??/// <summary>
??/// 賬號(hào)密碼
??/// </summary>
??public string Password;
??/// <summary>
??/// 賬號(hào)需要保存的數(shù)據(jù)
??/// </summary>
??public int Value;
}
using System.Collections.Generic;
using UnityEngine;
/// <summary>
/// 排行榜類
/// </summary>
public class Rankings : MonoBehaviour
{
??[SerializeField]
??//保存所有賬號(hào)的名字
??private List<string> accountsName;
??//排行榜的顯示文本
??private TMPro.TMP_Text text;
??//保存數(shù)據(jù)庫(kù)實(shí)例
??private DataBase dataBase;
??//當(dāng)對(duì)象被激活時(shí)調(diào)用一次
??private void OnEnable()
??{
????//獲得管理類的數(shù)據(jù)庫(kù)實(shí)例
????dataBase = LoginManager.Instance.dataBase;
????//將文本框文本清空
????text.text = string.Empty;
????//獲得所有賬號(hào)的名字
????accountsName = dataBase.GetAllAccountName();
????//獲得排序后的賬號(hào)列表
????accountsName = SortList(accountsName);
????UpdateView(accountsName);
??}
??private void Awake()
??{
????text = transform.GetChild(0).GetChild(2).GetComponent<TMPro.TMP_Text>();
??}
??/// <summary>
??/// 直接插入算法
??/// </summary>
??/// <param name="list">需要排序的列表</param>
??public List<string> SortList(List<string> list)
??{
????//首先從第二個(gè)元素開(kāi)始遍歷
????for (int i = 1; i < list.Count; i++)
????{
??????string value = list[i];
??????bool isMin = true;
??????//讓 i 下標(biāo)前的全部數(shù)據(jù)和 i下標(biāo)數(shù)據(jù)進(jìn)行比較
??????for (int j = i - 1; j >= 0; j--)
??????{
????????if (dataBase.GetAccountValue(list[j]) < dataBase.GetAccountValue(value))//只要比i下標(biāo)數(shù)據(jù)大就向后移一位下標(biāo),
????????{
??????????list[j + 1] = list[j];
????????}
????????else//如果比i下標(biāo)數(shù)據(jù)小或者相等,那么就把i下標(biāo)數(shù)據(jù)賦值到他后面
????????{
??????????list[j + 1] = value;
??????????isMin = false;
??????????break;//這里需要跳出循環(huán),因?yàn)榍懊娴闹刀际潜萯下標(biāo)數(shù)據(jù)小
????????}
??????}
??????if (isMin)//如果遍歷完了,前面的數(shù)據(jù)都比我大,那i下標(biāo)數(shù)據(jù)就到第一個(gè)位置去
??????{
????????list[0] = value;
??????}
????}
????return list;
??}
??public void UpdateView(List<string> list)
??{
????for (int i = 0; i < list.Count; i++)
????{
??????//顯示分?jǐn)?shù)前十的玩家
??????if (i < 10)
??????{
????????text.text += "第" + (i+1) + "名 玩家:" + list[i] + " 分?jǐn)?shù):" + dataBase.GetAccountValue(list[i]) + "\n";
??????}
??????else
??????{
????????break;
??????}
????}
????string name = PlayerPrefs.GetString("csName", "null");
????for (int i = 0; i < list.Count; i++)
????{
??????if (name == "null")
??????{
????????text.text += "你目前還未登錄,無(wú)法查詢到排行;";
????????break;
??????}
??????if (list[i] == name)
??????{
????????text.text += "你當(dāng)前排名:" + (i+1) + " (玩家:" + list[i] + ")";
??????}
????}
??}
}
using UnityEngine;
using TMPro;
/// <summary>
/// 對(duì)話框類
/// </summary>
public class PromptBox : MonoBehaviour
{
??//顯示框顯示的文本
??private TMP_Text text;
??//獲得場(chǎng)景中對(duì)象的組件給變量賦值
??private void Awake()
??{
????text = transform.GetChild(0).transform.GetChild(0).GetComponent<TMP_Text>();
??}
??//顯示對(duì)話框以及顯示文字
??public void ShowBox(string showText)
??{
????this.gameObject.SetActive(true);
????text.text = showText;
??}
??//動(dòng)畫(huà)結(jié)束時(shí)調(diào)用的函數(shù)
??public void End()
??{
????this.gameObject.SetActive(false);
??}
}