python使用matplotlib繪制簡單圖形
1,繪制一個(gè)圓
import matplotlib.pyplot as plt
def creat_circle():
? ? circle=plt.Circle((0,0),radius=0.5,fc='y',ec='r')#圓心坐標(biāo),半徑,內(nèi)部及邊緣填充顏色
? ? return circle
def show_shape(path):
? ? ax=plt.gca() #獲取當(dāng)前對象
? ? ax.add_patch(path) #給對象傳遞添加的塊
? ? ax.set_aspect('equal') #設(shè)置圖形的寬高比,equal為1:1
? ? plt.axis('scaled') #設(shè)置自動(dòng)調(diào)節(jié)數(shù)軸范圍
? ? plt.show()
c=creat_circle()
show_shape(c)
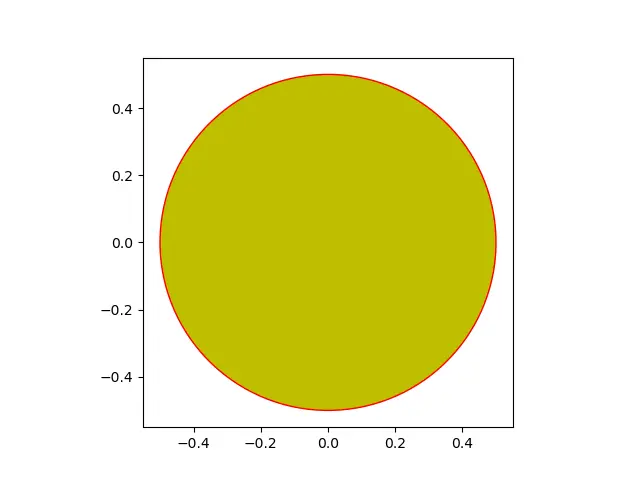
2,繪制動(dòng)圖
from matplotlib import pyplot as plt
from matplotlib import animation
def creat_circle():
? ? circle=plt.Circle((0,0),radius=0.05,fc='y',ec='r')#圓心坐標(biāo),半徑,內(nèi)部及邊緣填充顏色
? ? return circle
def update_radius(i,circle):#調(diào)用時(shí)自動(dòng)傳遞的幀編號,每一幀需要的對象;返回也是一個(gè)幀對象
? ? circle.radius=i*0.5
? ? return circle
def creat_animation():
? ? fig=plt.gcf() #獲取當(dāng)前Figure對象的引用
? ? ax=plt.axes()#創(chuàng)建坐標(biāo)系并設(shè)定取值范圍
? ? plt.xlim(-10,10),plt.ylim(-10,10)
? ? ax.set_aspect('equal')
? ? circle=creat_circle()
? ? ax.add_patch(circle) #將圓添加到當(dāng)前坐標(biāo)系下
? ? anim=animation.FuncAnimation(
? ? ? ? fig,update_radius,fargs=(circle,),frames=30,interval=50)#傳給FunAnimatin繪制動(dòng)圖的參數(shù)
? ? #依次為:當(dāng)前Figure對象,繪制每幀的函數(shù),給前面的函數(shù)通過元組傳遞參數(shù)(幀數(shù)不用傳遞)
? ? #幀數(shù)(這里選30幀),兩幀之間的時(shí)間間隔(這里是50毫秒)
? ?
? ? plt.title('Dynamic circle')#設(shè)置標(biāo)題
? ? plt.show() #顯示動(dòng)圖
? ? anim.save('circle.gif',writer='imagemagick')#在當(dāng)前目錄下保存動(dòng)圖
creat_animation()
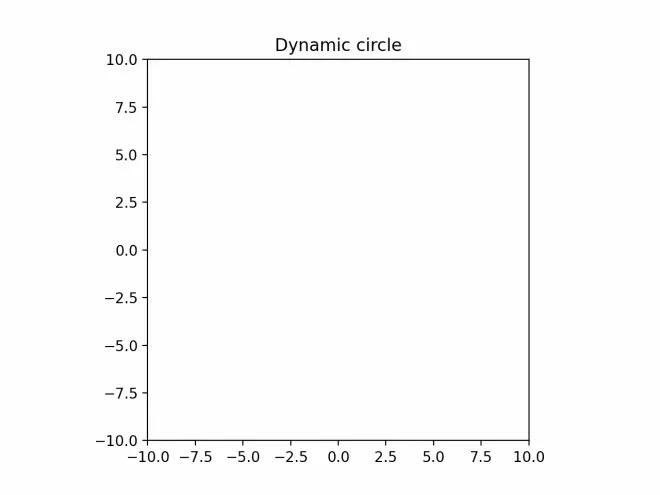
3,繪制拋物線動(dòng)圖:
from matplotlib import pyplot as plt
from matplotlib import animation
import math
g=9.8
def get_intervals(u,theta): #u為初合速度,theta為合速度與地面夾角
? ? t_flight=2*u*math.sin(theta)/g
? ? #由拋物線公式得:總飛行時(shí)間為兩倍的豎直方向速度除豎直加速度g
? ? intervals=[]
? ? start=0
? ? interval=0.005
? ? #以0.5為間隔創(chuàng)建列表,以便于后續(xù)幀與幀之間代表物體運(yùn)動(dòng)的時(shí)間間隔為0.5
? ? while start<t_flight:
? ? ? ? intervals.append(start)
? ? ? ? start+=interval
? ? return intervals
def update_position(i,circle,intervals,u,theta):#更新每一幀坐標(biāo)
? ? t=intervals[i] #傳遞每一幀對應(yīng)的物體實(shí)際運(yùn)動(dòng)時(shí)間
? ? x=u*math.cos(theta)*t
? ? y=u*math.sin(theta)*t-0.5*g*t*t
? ? #根據(jù)拋物線公式算出確定時(shí)間下對應(yīng)的位置坐標(biāo)
? ? circle.center=x,y #傳入球心坐標(biāo)
? ? return circle
def create_animation(u,theta):
? ? intervals=get_intervals(u,theta)
? ? xmin,ymin=0,0
? ? xmax=u*math.cos(theta)*intervals[-1]
? ? #以最大時(shí)間值算出x坐標(biāo)最大值
? ? tmax=u*math.sin(theta)/g
? ? #飛到最高點(diǎn)所用時(shí)間
? ? ymax=u*math.sin(theta)*tmax-0.5*g*tmax**2
? ? #最高點(diǎn)縱坐標(biāo)
? ? fig=plt.gcf()
? ? ax=plt.axes()
? ? plt.xlim(xmin,xmax),plt.ylim(ymin,ymax)
? ? circle=plt.Circle((xmin,ymin),0.1) #從起點(diǎn)開始畫圓
? ? ax.add_patch(circle)#添加圓在坐標(biāo)系下
? ? ax.set_aspect('equal')
? ? anim=animation.FuncAnimation(fig,update_position,fargs=\
? ? (circle,intervals,u,theta),frames=len(intervals),interval=1)
? ? #這里可以參加參數(shù)repeat=False讓動(dòng)圖不要無限重復(fù)
? ? plt.title('parabolic movement')
? ? plt.xlabel('X')
? ? plt.ylabel('Y')
? ? plt.show()
? ? anim.save('move.gif',writer='imagemagick')
try:
? ? u=float(input('enter the initial velocity:'))
? ? theta=float(input('enter the angle of projection:'))
except: #避免錯(cuò)誤輸入
? ? print('wrong input')
else:
? ? theta=math.radians(theta)#轉(zhuǎn)為弧度制
? ? create_animation(u,theta)
當(dāng)我輸入初速度為10,角度為45
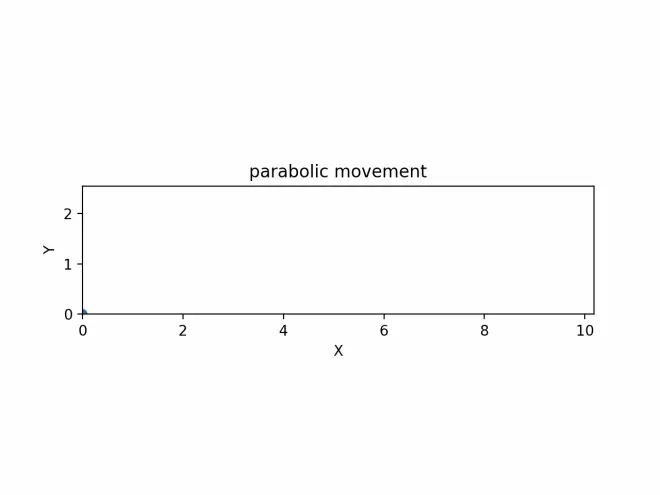
4,繪制分形:
'''
繪制分形:
其實(shí)就是由一些簡單的圖形組合而成
這些獨(dú)立的圖形需要提供初始位置和遞推關(guān)系式(類似于數(shù)列的感覺)
下面舉一個(gè)隨機(jī)游走的例子,即下一個(gè)點(diǎn)的坐標(biāo),橫坐標(biāo)加一,縱坐標(biāo)可能加一或者減一
''' ?
from matplotlib import pyplot as plt
import random
def trans1(p):#p為儲(chǔ)存x,y坐標(biāo)的元組,先往下看
? ? #縱坐標(biāo)+1
? ? x=p[0]
? ? y=p[1]
? ? return x+1,y+1
def trans2(p):
? ? #縱坐標(biāo)-1
? ? x=p[0]
? ? y=p[1]
? ? return x+1,y-1
def transform(p):
? ? trans=[trans1,trans2] #將函數(shù)作為對象
? ? t=random.choice(trans) #隨機(jī)選擇一個(gè)函數(shù)
? ? x,y=t(p) #對選中的函數(shù)進(jìn)行調(diào)用
? ? return x,y
def update_site(p,n):#n為坐標(biāo)隨機(jī)更新次數(shù)
? ? x=[p[0]]
? ? y=[p[1]]#創(chuàng)建列表先存入初始坐標(biāo)
? ? for _ in range(n):
? ? ? ? p=transform(p) #隨機(jī)調(diào)用函數(shù)更新坐標(biāo)
? ? ? ? x.append(p[0])
? ? ? ? y.append(p[1])#存入更新后的坐標(biāo)值
? ? return x,y #返回完整的坐標(biāo)列表
p=(1,1) #初始位置
n=int(input('enter the number of tries:'))
x,y=update_site(p,n)
plt.plot(x,y) #根據(jù)返回列表繪制
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
比如我輸入10000
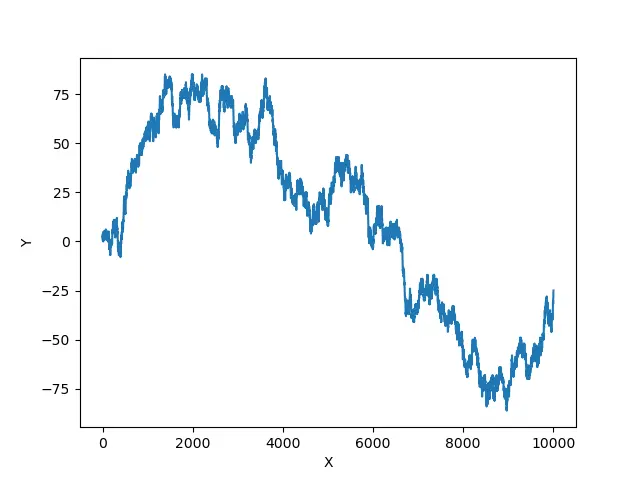
同樣的輸入,每次輸出結(jié)果也不一樣。
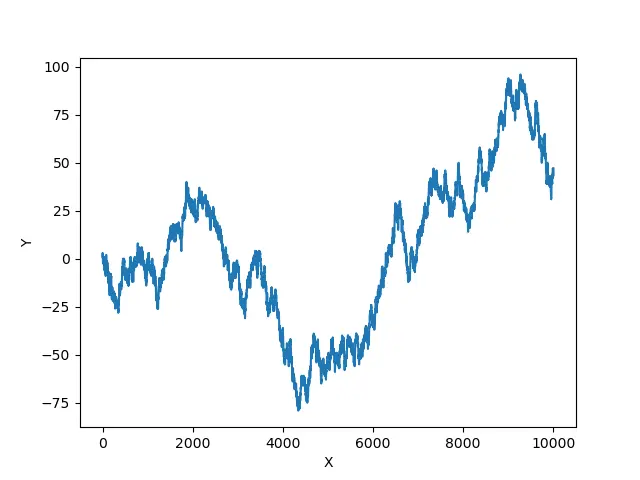
5,更有趣的是,我們可以繪制一些有趣的圖形。
原理:以一個(gè)確定的點(diǎn),但按照實(shí)現(xiàn)分配的概率執(zhí)行變換規(guī)則(依概率執(zhí)行遞推關(guān)系式)
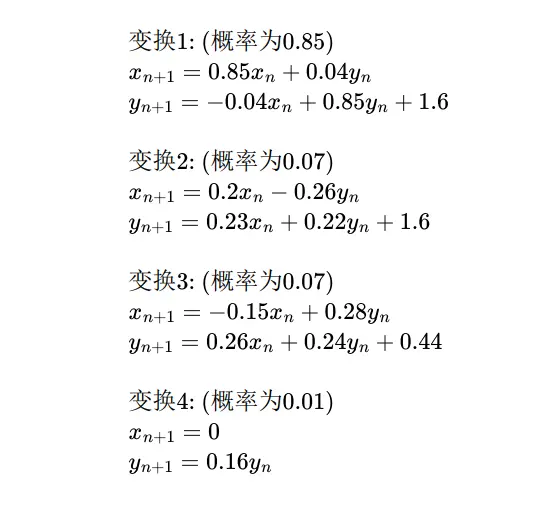
from matplotlib import pyplot as plt
import random
def trans1(p):
? ? x=p[0]
? ? y=p[1]
? ? x1=0.85*x+0.04*y
? ? y1=-0.04*x+0.85*y+1.6
? ? return x1,y1
def trans2(p):
? ? x=p[0]
? ? y=p[1]
? ? x1=0.2*x-0.26*y
? ? y1=0.23*x+0.22*y+1.6
? ? return x1,y1
def trans3(p):
? ? x=p[0]
? ? y=p[1]
? ? x1=-0.15*x+0.28*y
? ? y1=0.26*x+0.24*y+0.44
? ? return x1,y1
def trans4(p):
? ? x=p[0]
? ? y=p[1]
? ? x1=0
? ? y1=0.16*y
? ? return x1,y1
#非均勻隨機(jī)數(shù)
def unequal_probability_random_number(probability):
? ? #輸出調(diào)用函數(shù)的位置
? ? begin=0
? ? sum_probability=[] #從分布函數(shù)角度來實(shí)現(xiàn)
? ? for p in probability:
? ? ? ? begin+=p
? ? ? ? sum_probability.append(begin) #記錄每個(gè)離散點(diǎn)的分布函數(shù)值
? ? r=random.random() #產(chǎn)生0到1均勻隨機(jī)數(shù)
? ? for index,distribution in enumerate(sum_probability):
? ? ? ? if r<=distribution:
? ? ? ? ? ? return index
? ? return len(probability-1)
def transform(p):
? ? trans=[trans1,trans2,trans3,trans4]
? ? probability=[0.85,0.07,0.07,0.01]#設(shè)置各個(gè)函數(shù)調(diào)用概率
? ? site=unequal_probability_random_number(probability)
? ? t=trans[site] #依概率選擇一個(gè)函數(shù)
? ? x,y=t(p) #對選中的函數(shù)進(jìn)行調(diào)用
? ? return x,y
def update_site(n):
? ? x=[0]
? ? y=[0]#創(chuàng)建列表先存入初始坐標(biāo)
? ? x1,y1=0,0
? ? for _ in range(n):
? ? ? ? x1,y1=transform((x1,y1))
? ? ? ? x.append(x1)
? ? ? ? y.append(y1)#存入更新后的坐標(biāo)值
? ? return x,y #返回完整的坐標(biāo)列表
n=int(input('enter the number of tries:'))
x,y=update_site(n)
plt.plot(x,y,'o') #繪制點(diǎn)
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
比如輸入n=1000
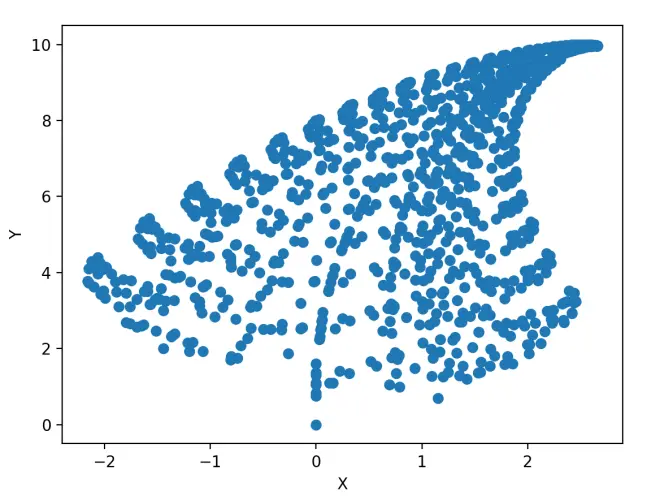
亦或者輸入n=5000
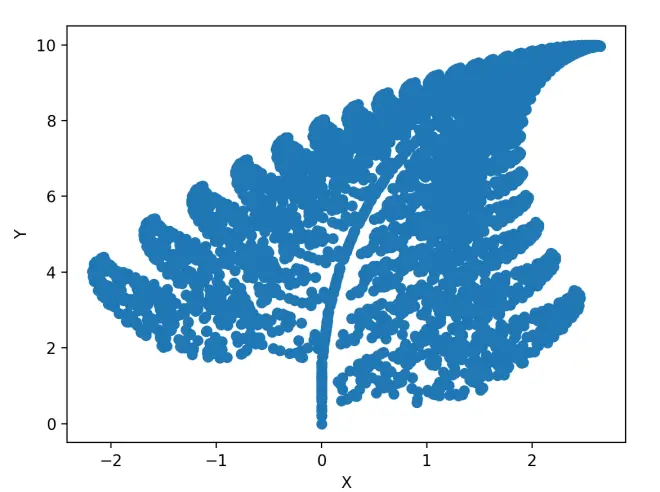
恩,它現(xiàn)在很像一個(gè)蕨類植物了,有趣吧~~~