Linux驅(qū)動(dòng)讀取當(dāng)前內(nèi)核中代碼段數(shù)據(jù)(超詳細(xì))
直接上代碼,思路就是得到代碼段的起始地址和結(jié)束地址,然后先將數(shù)據(jù)存在一個(gè)buffer中,最后寫(xiě)入文件。
kallsym.c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kallsyms.h>
static char *path = NULL;
module_param(path, charp, S_IRUGO);
static int __write_to_file(char *buffer, int size)
{
struct file *filep = NULL;
loff_t pos = 0;
int ret = 0;
filep = filp_open(path, O_RDWR | O_CREAT, 0);
if(filep == NULL) {
printk("%s: Open file %s error\n", __func__, path);
return 0;
}
ret = kernel_write(filep, buffer, size, &pos);
if(ret < size) {
printk("%s: Write file %s error\n", __func__, path);
return 0;
}
filp_close(filep, NULL);
return ret;
}
static int __init kallsyms_init(void)
{
unsigned long stext, etext, size = 0;
char *ptr = NULL;
char *buffer = NULL;
int i = 0;
stext = kallsyms_lookup_name("_stext");
etext = kallsyms_lookup_name("_etext");
printk("%s: the _stext address is %lx\n", __func__, stext);
printk("%s: the _stext address is %lx\n", __func__, etext);
if(!stext || !etext) {
printk("%s: Get text start end failed\n", __func__);
return -EINVAL;
}
size = etext - stext;
ptr = (char *)stext;
buffer = vzalloc(size + 1024);
if(buffer == NULL) {
printk("%s: Alloc temp buffer memory failed\n", __func__);
return 0;
}
for(i = 0; i < size; i++) {
buffer[i] = ptr[i];
}
__write_to_file(buffer, size);
vfree(buffer);
return 0;
}
static void __exit kallsyms_exit(void)
{
printk("%s: good bye\n", __func__);
}
module_init(kallsyms_init);
module_exit(kallsyms_exit);
MODULE_LICENSE("GPL");
【文章福利】小編推薦自己的Linux內(nèi)核技術(shù)交流群:【891587639】整理了一些個(gè)人覺(jué)得比較好的學(xué)習(xí)書(shū)籍、視頻資料共享在群文件里面,有需要的可以自行添加哦?。?!前100名進(jìn)群領(lǐng)取,額外贈(zèng)送一份價(jià)值699的內(nèi)核資料包(含視頻教程、電子書(shū)、實(shí)戰(zhàn)項(xiàng)目及代碼)??
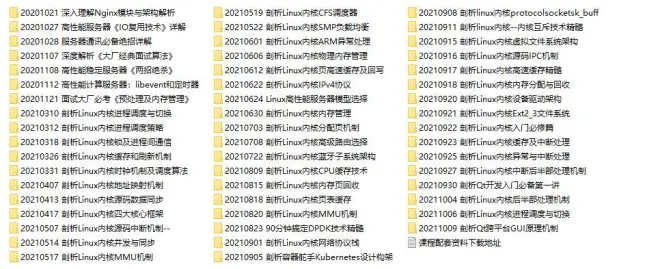
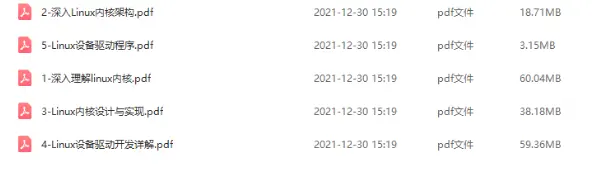
?
Makefile文件
obj-m += kallsym.o?
KBUILD_CFLAGS += -g?
all:?
make -C /lib/modules/$(shell uname -r)/build/ M=$(PWD) modules?
clean:?
make -C /lib/modules/$(shell uname -r)/build/ M=$(PWD) clean
以下代碼為dump指定內(nèi)存地址,大小為offset;
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kallsyms.h>
static char *path = NULL;
static unsigned long base_addr = 0;
static unsigned int offset = 0;
module_param(path, charp, S_IRUGO);
module_param(base_addr, ulong, S_IRUGO);
module_param(offset, uint, S_IRUGO);
static int __write_to_file(char *buffer, int size)
{
struct file *filep = NULL;
loff_t pos = 0;
int ret = 0;
filep = filp_open(path, O_RDWR | O_CREAT, 0);
if(filep == NULL) {
printk("%s: Open file %s error\n", __func__, path);
return 0;
}
ret = kernel_write(filep, buffer, size, &pos);
if(ret < size) {
printk("%s: Write file %s error\n", __func__, path);
return 0;
}
filp_close(filep, NULL);
return ret;
}
static int __init kallsyms_init(void)
{
unsigned long stext, etext;
char *ptr = NULL;
char *buffer = NULL;
int i = 0;
stext = base_addr;
etext = base_addr + offset;
printk("%s: the _stext address is %lx\n", __func__, stext);
printk("%s: the _stext address is %lx\n", __func__, etext);
ptr = (char *)stext;
buffer = vzalloc(offset + 100);
if(buffer == NULL) {
printk("%s: Alloc temp buffer memory failed\n", __func__);
return 0;
}
for(i = 0; i < offset; i++) {
buffer[i] = ptr[i];
}
__write_to_file(buffer, offset);
vfree(buffer);
return 0;
}
static void __exit kallsyms_exit(void)
{
printk("%s: good bye\n", __func__);
}
module_init(kallsyms_init);
module_exit(kallsyms_exit);
MODULE_LICENSE("GPL");
linux 查看進(jìn)程數(shù)據(jù)段,如何讀取Linux進(jìn)程中的代碼段和數(shù)據(jù)段
Linux下的程序的文件格式是ELF,里面分了各種段,有代碼段、數(shù)據(jù)段、等。當(dāng)運(yùn)行這個(gè)程序時(shí),系統(tǒng)也會(huì)給這個(gè)進(jìn)程創(chuàng)建虛擬內(nèi)存,然后把ELF中的數(shù)據(jù)分別加載到內(nèi)存中的對(duì)應(yīng)位置。本文整理了用cpp程序讀取內(nèi)存中的代碼段和rodata數(shù)據(jù)段的方法。
Ptrace
Ptrace是一個(gè)Linux系統(tǒng)提供的一個(gè)功能強(qiáng)大的API接口,可以讓一個(gè)進(jìn)程跟蹤或控制另一個(gè)進(jìn)程,調(diào)試程序GDB就是在這個(gè)系統(tǒng)調(diào)用的基礎(chǔ)上開(kāi)發(fā)的。
long ptrace(enum ptrace_request request,pid_t pid,void addr, void *data);
參數(shù)request 控制ptrace函數(shù)的行為,定義在sys/ptrace.h中。
參數(shù)pid 指定trace的進(jìn)程號(hào)。
以上兩個(gè)參數(shù)是必須的,之后兩個(gè)參數(shù)分別為地址和數(shù)據(jù),其含義由參數(shù)request控制。
/proc/pid/mem
mem是內(nèi)核創(chuàng)建的虛擬文件,是Linux的”一切皆文件”在進(jìn)程上的體現(xiàn),但是這個(gè)文件無(wú)法直接進(jìn)行讀取,需要先利用ptrace進(jìn)行綁定操作。
用ptrace綁定之后就可以用read來(lái)讀取這個(gè)“文件”了,但是要注意輸入讀取的地址不對(duì),也讀不出數(shù)據(jù)來(lái)。
/proc/pid/maps
下圖是Linux的進(jìn)程內(nèi)存布局,這是系統(tǒng)給進(jìn)程虛擬出的一個(gè)內(nèi)存空間,并不是實(shí)際的物理內(nèi)存,maps文件中就記錄了虛擬內(nèi)存的的每段地址分別對(duì)應(yīng)什么數(shù)據(jù)。
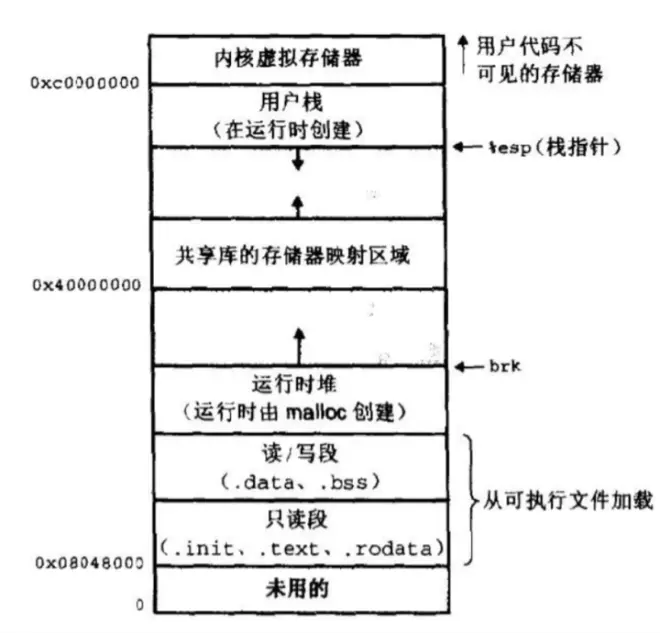
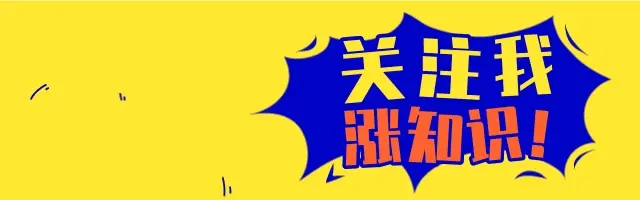