Lab Exercise 2
1. Select an interesting image, design a binarization rule (which can be a simple threshold or other complex functions), binarize the image, and display the original image and the binary image. Briefly explain the binarization rule and the result you obtained.
2. Select a picture (the size must be greater than 1024*1024), this question can be completed by calling the third-party functions. At the same time, I also encourage capable students to write their own implementation from scratch:
1) Resize the image to half the width and height, but keep the original size of the canvas, and the resized image should be centered (the resized image and canvas share the same center). All pixels on the canvas should be set to?blue. Plot the result.
2) Based on the result of 1), move the resized image by 200 pixels in the positive direction of the x-axis, and plot the result.
3) Based on the result of 2), Rotate the image (don't rotate canvas) 45 degrees counter-clockwise along the positive direction of the z-axis (pointing out screen) via the the center of the picture (not the center of the canvas),? and plot the result.
3. Histogram Equalization
1) Students are required to write your own code from scratch (Calling third-party functions to complete histogram, cumulative histogram, and equalization calculations are not allowed): to implement a single-channel histogram function, a single-channel cumulative histogram function and a single-channel histogram equalization function.
2) Select any color image,? and perform histogram equalization on its channels respectively. For each channel, display the original image, histogram, and cumulative histogram before equalization, as well as after equalization.
More specifically, 12 images for each channel are required to be plotted and arranged in the following way:
*Third?indicates that the result is generated by calling the third party function.
*Own?indicates that the result must be generated by calling your own function implemented in 1).
Original image (e.g. Red channel),? Histogram (*Third),? ?Cumulative Histogram (*Third)
Original image (e.g. Red channel),? Histogram (*Own),? ? Cumulative Histogram (*Own)
Equalized Image (*Third),? ? ? ? ? ? ? ? Histogram (*Third),? ?Cumulative Histogram (*Third)
Equalized Image(*Own),? ? ? ? ? ? ? ? ? Histogram (*Own),? ? Cumulative histogram (*Own)
?
There should be?36?images in total for three channels.??
3) Analyze your results and compare your results with third-party results. Are they consistent with each other? If not, please explain why there are differences.
作答(僅供參考,并不是對(duì)的)
import CV2 as cv
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image
import matplotlib.image as imag
import os
img=cv.imread("image/cda4671f75fb49c584d4332e76e7bf21.png")
plt.imshow(img)
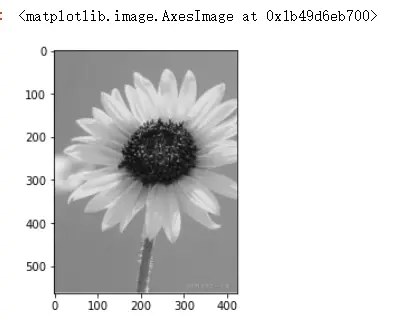
二值化
def two_value(image):
? ? gray = cv.cvtColor(image, cv.COLOR_BGR2GRAY)? ?##要二值化圖像,必須先將圖像轉(zhuǎn)為灰度圖
? ? ret, binary = cv.threshold(gray, 0, 255, cv.THRESH_BINARY | cv.THRESH_OTSU)
? ? cv.imwrite("image/two_value.png",binary)
? ? cv.waitKey(0)
? ? cv.destroyAllWindows()
def show(img):
? ? if img.ndim==2:
? ? ? ? plt.imshow(img,cmap='gray')
? ? else:
? ? ? ? plt.imshow(cv.cvtColor(img,cv.COLOR_BGR2RGB))
? ? plt.show()??
two_value(img)
test=cv.imread("image/two_value.png")
plt.figure()#創(chuàng)建畫(huà)布
plt.subplot(1,2,1)
plt.imshow(img)
plt.subplot(1,2,2)
plt.imshow(test)
plt.show()
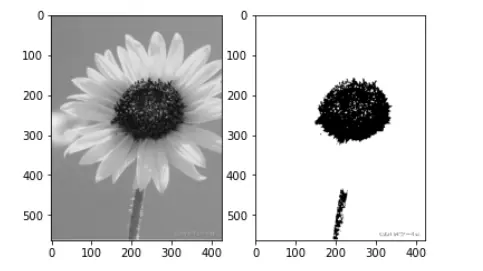
對(duì)第一題的解釋
直接寫(xiě)一個(gè)函數(shù)來(lái)轉(zhuǎn)換,使用cv里的函數(shù),我這里是直接讀取的灰值圖像,然后對(duì)灰值圖像進(jìn)行處理,使用cv的threshold 函數(shù)來(lái)及逆行全局闕值的處理, 參數(shù)的意思是THRESH_BINARY 超過(guò)閾值的值為最大值,其他值是0。CV2.THRESH_OTSU使用最小二乘法處理像素點(diǎn),而CV2.THRESH_TRIANGLE使用三角算法處理像素點(diǎn)。這樣超過(guò)闕值的是255 低于闕值的是黑色,實(shí)現(xiàn)二值化。得到的結(jié)果中,黑色被大量保留,其他則變?yōu)榘咨?/p>
第二題
img2=cv.imread("image/e1c0cfd9e202882aefe6fc5ae89be447.png")
np.shape(img2)
#對(duì)圖片進(jìn)行修改尺寸
size=(1200,1200)
img2_new=cv.resize(img2,size)
#那么如何手寫(xiě)修改尺寸呢
show(img2_new)
#縮小圖片,但保持畫(huà)布的大小不變,使用藍(lán)色填充
hight,width=img2_new.shape[:2]
size=(600,600)
img2_new=cv.resize(img2,size)
img2_new=cv.cvtColor(img2_new,cv.COLOR_BGR2RGB)
img2_new=Image.fromarray(img2_new)
img2_new.save("img2_new.png")
#創(chuàng)建一個(gè)畫(huà)布,藍(lán)色,然后把縮小的圖片放到中間
def InitCanvasV3(width, height, color=(255, 255, 255)):
? ? canvas = np.ones((height, width, 3), dtype="uint8")
? ? canvas[:] = color
? ? return canvas
def blend_two_images2(img1,img2):
? ? img1 = img1.convert('RGBA')
? ? img2 = img2.convert('RGBA')
? ? r, g, b, alpha = img2.split()
? ? alpha = alpha.point(lambda i: i>0 and 204)
? ? img = Image.composite(img2, img1, alpha)
? ? img.show()
? ? img.save( "image/blend2.png")
# 初始化一個(gè)藍(lán)色的畫(huà)布
canvas_color = InitCanvasV3(1200, 1200, color=(20, 100, 200))
#先保存
canvas_color=Image.fromarray(canvas_color)
canvas_color.save("canvas_color.png")
canvas_color=Image.open("canvas_color.png")
def Picture_Synthesis(M_Img,
? ? ? ? ? ? ? ? ? ? ? S_Img,
? ? ? ? ? ? ? ? ? ? ? save_img,
? ? ? ? ? ? ? ? ? ? ? coordinate=None):
? ? """
? ? :param mother_img: 母圖
? ? :param son_img: 子圖
? ? :param save_img: 保存圖片名
? ? :param coordinate: 子圖在母圖的坐標(biāo)
? ? :return:
? ? """
? ? #將圖片賦值,方便后面的代碼調(diào)用
? ? factor = 1#子圖縮小的倍數(shù)1代表不變,2就代表原來(lái)的一半
? ? #給圖片指定色彩顯示格式
? ? M_Img = M_Img.convert("RGBA")? # CMYK/RGBA 轉(zhuǎn)換顏色格式(CMYK用于打印機(jī)的色彩,RGBA用于顯示器的色彩)
? ? # 獲取圖片的尺寸
? ? M_Img_w, M_Img_h = M_Img.size? # 獲取被放圖片的大?。笀D)
? ? print("母圖尺寸:",M_Img.size)
? ? S_Img_w, S_Img_h = S_Img.size? # 獲取小圖的大?。ㄗ訄D)
? ? print("子圖尺寸:",S_Img.size)
? ? size_w = int(S_Img_w / factor)
? ? size_h = int(S_Img_h / factor)
? ? # 防止子圖尺寸大于母圖
? ? if S_Img_w > size_w:
? ? ? ? S_Img_w = size_w
? ? if S_Img_h > size_h:
? ? ? ? S_Img_h = size_h
? ? # # 重新設(shè)置子圖的尺寸
? ? # icon = S_Img.resize((S_Img_w, S_Img_h), Image.ANTIALIAS)
? ? icon = S_Img.resize((S_Img_w, S_Img_h), Image.ANTIALIAS)
? ? w = int((M_Img_w - S_Img_w) / 2)
? ? h = int((M_Img_h - S_Img_h) / 2)
? ? try:
? ? ? ? if coordinate==None or coordinate=="":
? ? ? ? ? ? coordinate=(w, h)
? ? ? ? ? ? # 粘貼子圖到母圖的指定坐標(biāo)(當(dāng)前居中)
? ? ? ? ? ? M_Img.paste(icon, coordinate, mask=None)
? ? ? ? else:
? ? ? ? ? ? print("已經(jīng)指定坐標(biāo)")
? ? ? ? ? ? # 粘貼子圖到母圖的指定坐標(biāo)(當(dāng)前居中)
? ? ? ? ? ? M_Img.paste(icon, coordinate, mask=None)
? ? except:
? ? ? ? print("坐標(biāo)指定出錯(cuò) ")
? ? # 保存圖片
? ? M_Img.save(save_img)
Picture_Synthesis(canvas_color,img2_new,save_img="image/newimg.png",coordinate=(300,300))
image_new=cv.imread("image/newimg.png")
show(image_new)
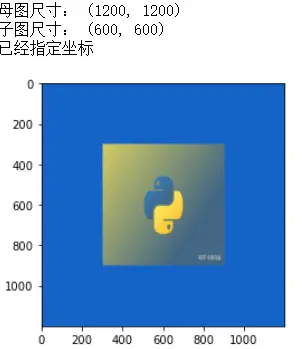
M = np.float32([[1, 0, 200], [0, 1, 0]])
shifted = cv.warpAffine(image_new, M, (width, hight))
show(shifted)
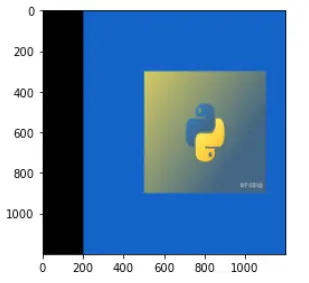
#3)根據(jù)2)的結(jié)果,通過(guò)畫(huà)面中心(不是畫(huà)布中心),將圖像(不要旋轉(zhuǎn)畫(huà)布)沿z軸正方向(指向屏幕外)逆時(shí)針旋轉(zhuǎn)45度,繪制結(jié)果。
test_3=cv.getRotationMatrix2D((width//2,hight//2),45,1)
image_rotation=cv.warpAffine(shifted,test_3,(width,hight),borderValue=(0,0,255))
show(image_rotation)
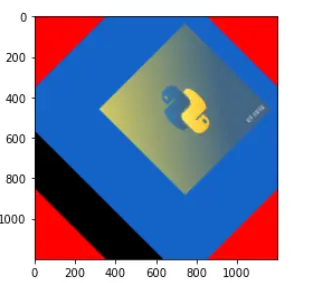
#實(shí)現(xiàn)單通道直方圖函數(shù)、單通道累積直方圖函數(shù)和單通道直方圖均衡函數(shù)。
from os.path import isfile
from PIL import Image
import collections
import matplotlib.pyplot as plt
import numpy as np
import CV2 as cv
def show(img):
? ? if img.ndim==2:
? ? ? ? plt.imshow(img,cmap='gray')
? ? else:
? ? ? ? plt.imshow(cv.cvtColor(img,cv.COLOR_BGR2RGB))
? ? plt.show()??
#單通道直方圖函數(shù)
#轉(zhuǎn)成一維列表
def test_ravel(gray):
? ? a=[]
? ? for i in np.array(gray):
? ? ? ? for y in i:
? ? ? ? ? ? #這里已經(jīng)拿到單個(gè)了,那么一唯列表
? ? ? ? ? ? a.append(y)
? ? return list(a)
#計(jì)算所欲灰度級(jí)
def test_Counter_gray(gray):
? ? sum={}
? ? for i in gray:
? ? ? ? if(i in sum.keys()):
? ? ? ? ? ? #存在
? ? ? ? ? ? sum[i]+=1
? ? ? ? else:
? ? ? ? ? ? #不存在
? ? ? ? ? ? sum[i]=1
? ? return sum
? ??
? ? ? ? ? ??
#直方圖函數(shù)
def clczhifangtu(gray) :
#? ? ?gray=gray.convert('L')
? ? hist_new = []
? ? num = []
? ? hist_result = []
? ? hist_key = []
? ? gray1 = test_ravel(gray)
? ? obj = test_Counter_gray(gray1)
#? ? ?print(obj)
? ? obj = sorted(obj.items(),key=lambda item:item[0])
? ? for each in obj :
? ? ? ? hist1 = []
? ? ? ? key = list(each)[0]
? ? ? ? each =list(each)[1]
? ? ? ? hist_key.append(key)
? ? ? ? hist1.append(each)
? ? ? ? hist_new.append(hist1)
? ??
? ? #檢查從0-255每個(gè)通道是否都有個(gè)數(shù),沒(méi)有的話添加并將值設(shè)為0
? ? for i in range (0,256) :
? ? ? ? if i in hist_key :
? ? ? ? ? ? num = hist_key.index(i)
? ? ? ? ? ? hist_result.append(hist_new[num])
? ? ? ? else :
? ? ? ? ? ? hist_result.append([0])
? ? if len(hist_result) < 256 : #檢查循環(huán)后的列表中是不是已經(jīng)包含所有的灰度級(jí)
? ? ? ? for i in range (0,256-len(hist_result)) :
? ? ? ? ? ? hist_new.append([0])
? ??
#? ? ?hist_result = np.array(hist_result)
? ? #這里不用np.array 會(huì)發(fā)生什么,都是數(shù)組
#? ? ?print(hist_result)
? ? return hist_result
im = Image.open('image/e1c0cfd9e202882aefe6fc5ae89be447.png')
im=im.convert('L')
# print(type(im))
# print(np.array(im))
y=clczhifangtu(im)#y已經(jīng)統(tǒng)計(jì)好區(qū)間了
# print(y)
plt.plot(y)
plt.show()
# print(np.array(im).ravel())
# test_ravel(im)
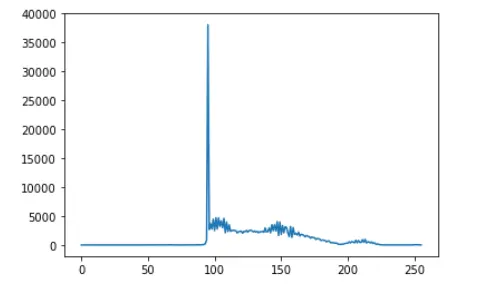
#單通道累計(jì)直方圖函數(shù)
def histogram_sum(gray):#繪制累計(jì)直方圖
#? ? ?cdf=clczhifangtu(gray)
#? ? ?cdf = 255.0 * cdf / cdf[-1]
? ??
? ??
? ? try:
? ? ? ? x = gray.size[0]
? ? ? ? y = gray.size[1]
? ? except:
? ? ? ? #對(duì)于是從open過(guò)來(lái)的函數(shù)
? ? ? ? gray= Image.fromarray(gray)
? ? ? ? x = gray.size[0]
? ? ? ? y = gray.size[1]
? ? ret = np.zeros(256)
? ??
? ? #如果不滿足則轉(zhuǎn)型呀
? ? try:
? ? ? ??
? ? ? ? for i in range(x):
? ? ? ? ? ? for j in range(y):
? ? ? ? ? ? ? ? k = gray.getpixel((i,j))#正常捕獲像素值
? ? ? ? ? ? ? ? ret[k] = ret[k ]+1
? ? except:
? ? ? ? #numpy.array捕獲像素值
#? ? ? ? ?gray= Image.fromarray(gray)
? ? ? ? for i in range(x):
? ? ? ? ? ? for j in range(y):
? ? ? ? ? ? ? ? k = gray.getpixel((i,j))#正常捕獲像素值
? ? ? ? ? ? ? ? ret[k] = ret[k]+1
#? ? ? ? ?print(type(gray))? <class 'PIL.Image.Image'>
? ? ? ? ? ??
? ??
? ? for k in range(1,256):
? ? ? ? ret[k] = ret[k]+ret[k-1]#累加
? ? for k in range(256):
? ? ? ? ret[k] = ret[k]/(x*y)
? ? return ret
im = Image.open('image/e1c0cfd9e202882aefe6fc5ae89be447.png')
im=im.convert('L')
plt.figure()
plt.bar(range(256),histogram_sum(im))#描繪柱狀圖
# histogram_sum(im)
# sum=0
# for i in clczhifangtu(im):
#? ? ?sum+=i
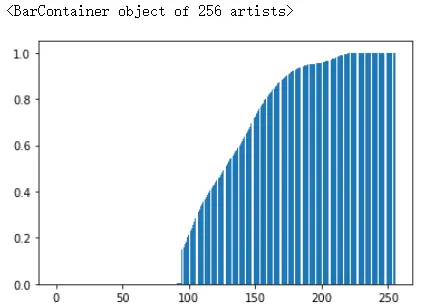
# 單通道直方圖均衡化
def probability_to_histogram(img, prob):
#? ? ?img.convert('L')
? ? # 根據(jù)像素概率將原始圖像直方圖均衡化
? ? # :param img:
? ? # :param prob:
? ? # :return: 直方圖均衡化后的圖像
? ? prob=histogram_sum(img)
? ? img_map = [int(i * prob[i]) for i in range(256)]? # 像素值映射#這里只是一個(gè)一唯的數(shù)組
? ?# 像素值替換
#? ? ?print(img)
#? ? ?print(img_map[1])
#? ? ?img=np.array(img)
#? ? ?print(img)
#? ? ?print(img[1][1])
? ? r, c = img.shape
? ? for ri in range(r):
? ? ? ? for ci in range(c):
? ? ? ? ? ? img[ri, ci] = img_map[img[ri, ci]]
? ? return img
img3=cv.imread("image/e1c0cfd9e202882aefe6fc5ae89be447.png", cv.IMREAD_GRAYSCALE)
show(img3)
pro_img=probability_to_histogram(img3,clczhifangtu(img3))
show(pro_img)
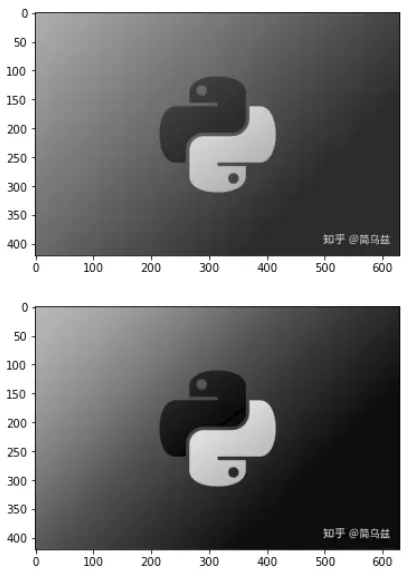
# 上面是測(cè)試
img_t=cv.imread("image/e1c0cfd9e202882aefe6fc5ae89be447.png")
show(img_t)
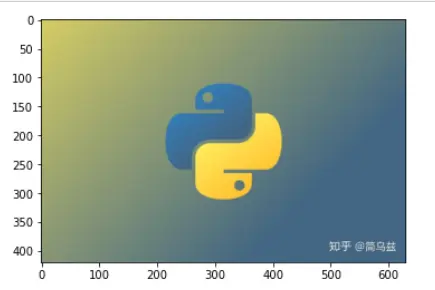
# 通道分離
b,g,r = cv.split(img_t)
# cv.imshow("Blue",r)
# cv.imshow("Red",g)
# cv.imshow("Green",b)
# cv.waitKey(0)
# cv.destroyAllWindows()
b 通道 均衡化前
# 原始圖像??
show(b)
#直方圖
plt.hist(b.ravel(), 256)
plt.title('*Thrid')
plt.show()
# 累積直方圖
hist_img, _ = np.histogram(b, 256)
cdf_img = np.cumsum(hist_img)? ?# accumulative histogram
plt.plot(range(256), cdf_img)
plt.title('*Thrid')
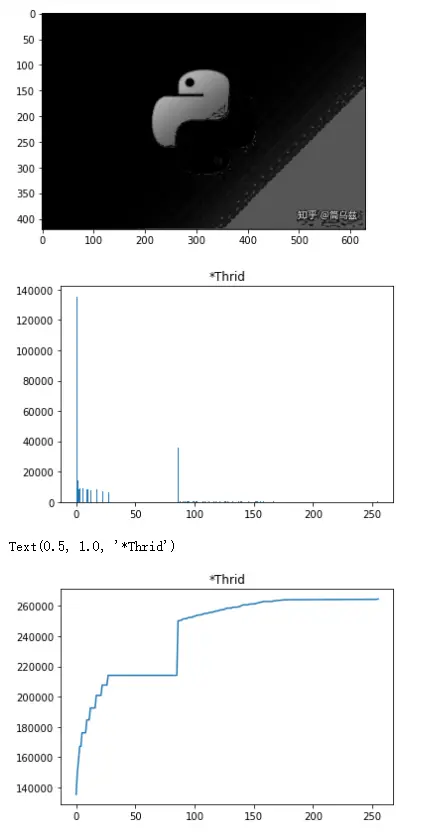
# 原始圖像??
show(b)
# 直方圖
y=clczhifangtu(b)
plt.plot(y)
plt.title("*Own")
plt.show()
#累積直方圖
plt.figure()
plt.title("*Own")
plt.bar(range(256),histogram_sum(b))#描繪柱狀圖
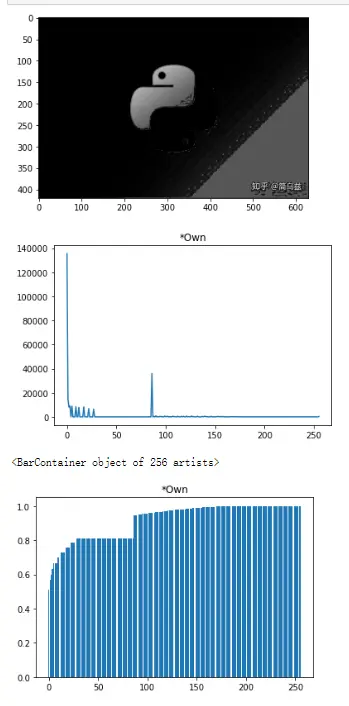
b 通道 均衡化后
# 均衡圖像(*Third),直方圖(*Third),累積直方圖(*Third)
#直方圖均衡化處理
result = cv.equalizeHist(b)
plt.title('*Thrid')
show(result)
#直方圖
plt.hist(result.ravel(), 256)
plt.title('*Thrid')
plt.show()
# 累積直方圖
hist_img, _ = np.histogram(result,256)
cdf_img = np.cumsum(hist_img)? ?# accumulative histogram
plt.plot(range(256), cdf_img)
plt.title('*Thrid')
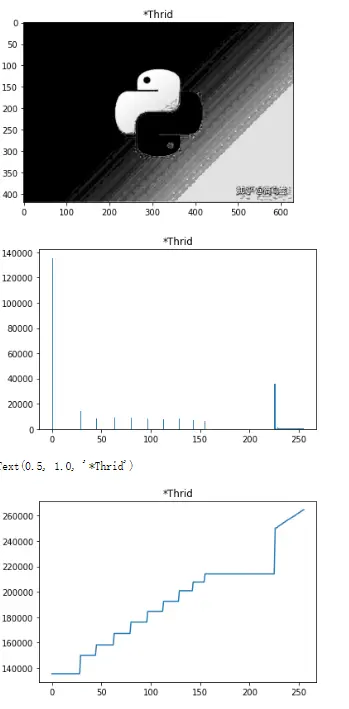
pro_img=probability_to_histogram(b,clczhifangtu(b))
plt.title("*Own")
show(pro_img)
# 直方圖
y=clczhifangtu(pro_img)
plt.plot(y)
plt.title("*Own")
plt.show()
#累積直方圖?
plt.figure()
plt.title("*Own")
plt.bar(range(256),histogram_sum(pro_img))#描繪柱狀圖
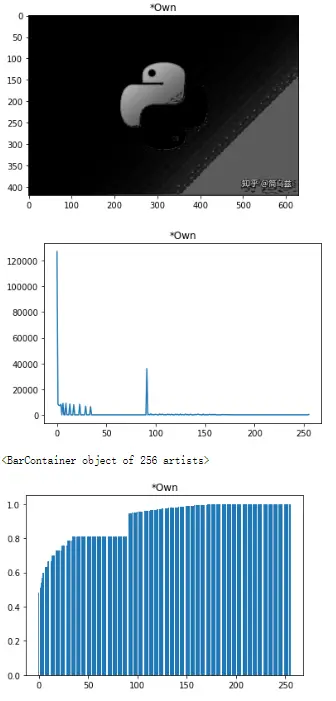
g通道 均衡化前
# 原始圖像??
show(g)
#直方圖
plt.hist(g.ravel(), 256)
plt.title('*Thrid')
plt.show()
# 累積直方圖
hist_img, _ = np.histogram(g, 256)
cdf_img = np.cumsum(hist_img)? ?# accumulative histogram
plt.plot(range(256), cdf_img)
plt.title('*Thrid')
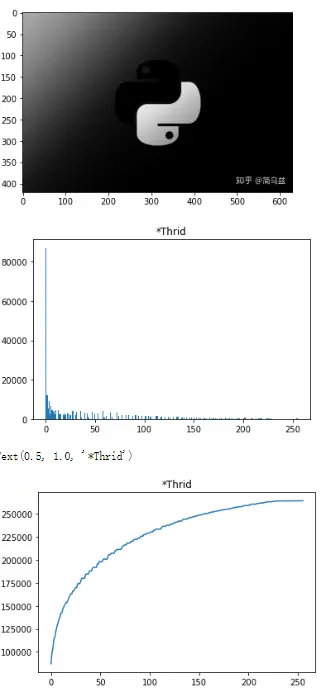
# 原始圖像??
show(g)
# 直方圖
y=clczhifangtu(g)
plt.plot(y)
plt.title("*Own")
plt.show()
#累積直方圖
plt.figure()
plt.title("*Own")
plt.bar(range(256),histogram_sum(g))#描繪柱狀圖
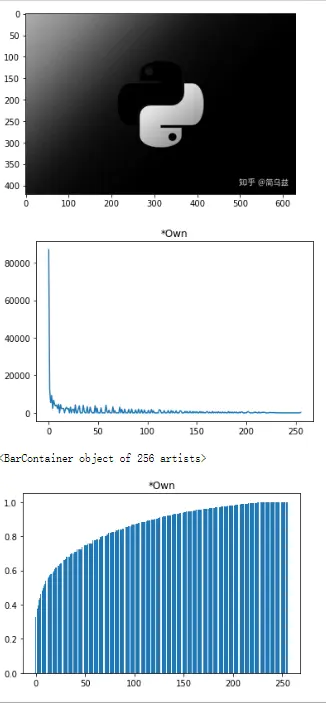
g通道 均衡化后
# 均衡圖像(*Third),直方圖(*Third),累積直方圖(*Third)
#直方圖均衡化處理
result = cv.equalizeHist(g)
plt.title('*Thrid')
show(result)
#直方圖
plt.hist(result.ravel(), 256)
plt.title('*Thrid')
plt.show()
# 累積直方圖
hist_img, _ = np.histogram(result,256)
cdf_img = np.cumsum(hist_img)? ?# accumulative histogram
plt.plot(range(256), cdf_img)
plt.title('*Thrid')
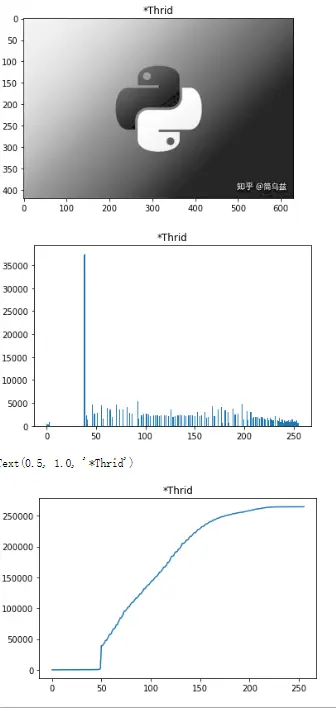
pro_img=probability_to_histogram(g,clczhifangtu(g))
plt.title("*Own")
show(pro_img)
# 直方圖
y=clczhifangtu(pro_img)
plt.plot(y)
plt.title("*Own")
plt.show()
#累積直方圖?
plt.figure()
plt.title("*Own")
plt.bar(range(256),histogram_sum(pro_img))#描繪柱狀圖
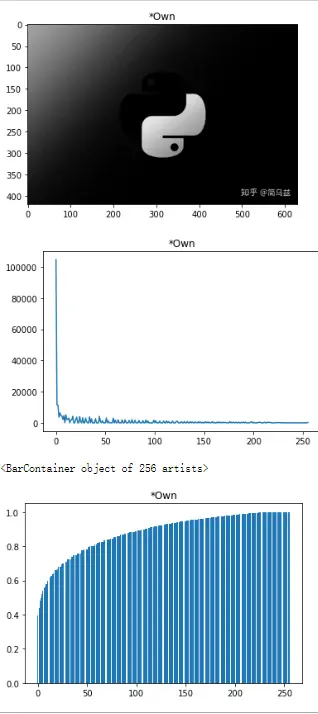
r通道 均衡化前
# 原始圖像??
show(r)
#直方圖
plt.hist(r.ravel(), 256)
plt.title('*Thrid')
plt.show()
# 累積直方圖
hist_img, _ = np.histogram(r, 256)
cdf_img = np.cumsum(hist_img)? ?# accumulative histogram
plt.plot(range(256), cdf_img)
plt.title('*Thrid')
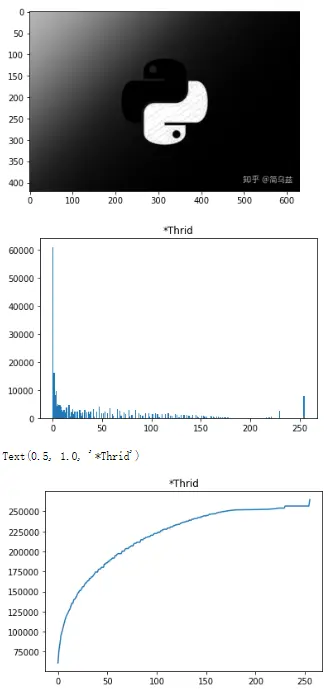
# 原始圖像??
show(r)
# 直方圖
y=clczhifangtu(r)
plt.plot(y)
plt.title("*Own")
plt.show()
#累積直方圖
plt.figure()
plt.title("*Own")
plt.bar(range(256),histogram_sum(r))#描繪柱狀圖
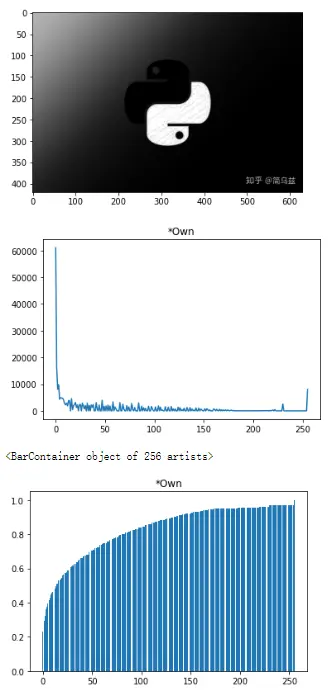
r通道 均衡化后?
# 均衡圖像(*Third),直方圖(*Third),累積直方圖(*Third)
#直方圖均衡化處理
result = cv.equalizeHist(r)
plt.title('*Thrid')
show(result)
#直方圖
plt.hist(result.ravel(), 256)
plt.title('*Thrid')
plt.show()
# 累積直方圖
hist_img, _ = np.histogram(result,256)
cdf_img = np.cumsum(hist_img)? ?# accumulative histogram
plt.plot(range(256), cdf_img)
plt.title('*Thrid')
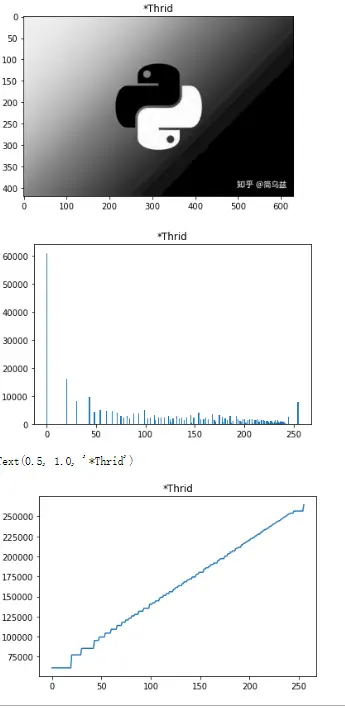
pro_img=probability_to_histogram(r,clczhifangtu(r))
plt.title("*Own")
show(pro_img)
# 直方圖
y=clczhifangtu(pro_img)
plt.plot(y)
plt.title("*Own")
plt.show()
#累積直方圖?
plt.figure()
plt.title("*Own")
plt.bar(range(256),histogram_sum(pro_img))#描繪柱狀圖
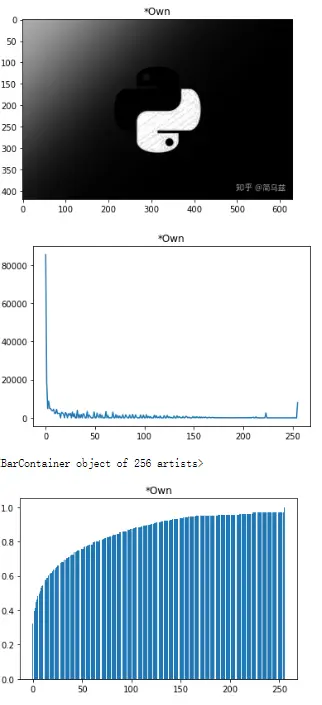
分析結(jié)果并與之比較
直方圖方面自己發(fā)函數(shù)與第三方函數(shù)相比較容易丟失數(shù)據(jù)或者處理不到位,產(chǎn)生偏差,統(tǒng)計(jì)的不到位,在直方圖顯示上,咱自己的函數(shù)大體沒(méi)啥問(wèn)題,主要是有些小灰度上統(tǒng)計(jì)不好,應(yīng)該是把一些算到其他地方去了 累計(jì)直方圖的話,除了表現(xiàn)形式不同,大體的趨勢(shì)和精準(zhǔn)度是差不多的
在均衡化方面,對(duì)于灰度級(jí)0的處理是不到位的,其他數(shù)級(jí)的分配是好的,總體能滿足,但是依然有點(diǎn)瑕疵,應(yīng)該對(duì)第一組數(shù)據(jù)進(jìn)行詳細(xì)在處理,不然達(dá)不到道理