【中英對(duì)譯】MCEdit 2 Documentation(1)
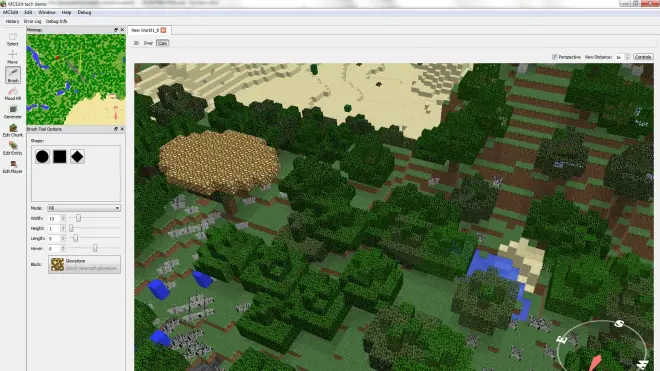
MCEdit 2 Plugin Development
MCEdit 2插件開(kāi)發(fā)
A plugin for MCEdit 2 is a Python module that defines one or more Plugin classes and then registers them with MCEdit. These classes are instantiated once for each Editor Session and called on by MCEdit in response to various user actions.
mcedit 2的插件是一個(gè)python模塊,它定義一個(gè)或多個(gè)插件類,然后將它們注冊(cè)到mcedit。這些類為每個(gè)編輯器會(huì)話實(shí)例化一次,并由mcedit響應(yīng)各種用戶操作調(diào)用。
·Plugin basics?插件基礎(chǔ)
··Plugin Structure?插件結(jié)構(gòu)
··Undo History?撤消歷史記錄
··Registering Plugin Classes?正在注冊(cè)插件類
·Plugin Tasks?插件任務(wù)
··World Dimensions?世界維度
··Editing Blocks?編輯塊
··Editing Entities?編輯實(shí)體
··Creating entities?創(chuàng)建實(shí)體
·Types of plugins?插件類型
··Command Plugins?命令插件
··Simple Commands?簡(jiǎn)單命令
···Simple Command Inputs?簡(jiǎn)單命令輸入
····Input Types?輸入類型
··Tool Plugins?工具插件
···Mouse Handlers?鼠標(biāo)處理程序
··Brush Shape Plugins?畫(huà)筆形狀插件
···As a boolean array?作為布爾數(shù)組
···As a Selection object?作為選擇對(duì)象
·Plugin Examples?插件示例
··Simple Options?簡(jiǎn)單選項(xiàng)
··World Editing?世界編輯

Plugin basics?插件基礎(chǔ)
Plugin Structure?插件結(jié)構(gòu)
A plugin is defined to be a Python module. A module can take several forms. The simplest is a single python file, such as?my_plugin.py?that contains plugin class definitions.
插件被定義為Python模塊。一個(gè)模塊可以有多種形式。最簡(jiǎn)單的是一個(gè)單獨(dú)的python文件,比如包含插件類定義的my_plugin.py。
For larger plugins, or plugins that include other support files, you may choose to create a Python package, which is a folder that contains (at the very least) an?__init__.py?file. For example:
對(duì)于更大的插件或包含其他支持文件的插件,您可以選擇創(chuàng)建一個(gè)Python包,該包是一個(gè)文件夾,其中至少包含一個(gè)__init__.py文件。例如:
my_plugin/
????__init__.py
????helpers.py
????header_image.png
????footer_image.png
TBD: In the future, it will be possible to package your plugin as a zip file for easy installation.
TBD:將來(lái),可以將您的插件打包為zip文件,以便安裝。
Undo History?撤消歷史記錄
NOTE: The following mainly applies to the full-featured?CommandPlugin. Plugins derived?from?SimpleCommandPlugin?or?BrushMode?will automatically manage the undo history for you.
注:以下主要應(yīng)用于功能齊全的CommandPlugin。從SimpleCommandPlugin或BrushMode派生的插件將自動(dòng)為您管理撤消歷史記錄。
Plugins that edit the world must make it possible for these edits to be undone. This is done by enclosing your editing commands within a call to?editorSession.beginSimpleCommand. This creates a new entry in the undo history and tells the editorSession to begin recording undo information. If this function is not called, the world will be in read-only mode and editing will not be possible. For example:
編輯世界的插件必須使這些編輯得以撤銷。這是通過(guò)將編輯命令封閉在對(duì)editorSession.beginSimpleCommand的調(diào)用中來(lái)完成的。這將在撤消歷史記錄中創(chuàng)建一個(gè)新條目,并通知editorSession開(kāi)始記錄撤消信息。如果不調(diào)用此函數(shù),則世界將處于只讀模式,無(wú)法進(jìn)行編輯。例如:
def?changeOneBlock():
????with?editorSession.beginSimpleCommand("Change One Block"):
????????editorSession.currentDimension.setBlock(1, 2, 3, "minecraft:dirt")
Registering Plugin Classes?正在注冊(cè)插件類
When defining a plugin class, you must also call a function to register it with MCEdit’s plugin handling system. This is usually as simple as placing a decorator such as?@registerCommandPlugin?before the class definition. See the examples for each of the?Types of plugins.
定義一個(gè)插件類時(shí),還必須調(diào)用一個(gè)函數(shù),以便在MCEdit的插件處理系統(tǒng)中注冊(cè)它。這通常與將諸如@registerCommandPlugin之類的修飾符放在類定義之前一樣簡(jiǎn)單。請(qǐng)參閱每個(gè)插件類型的示例。

Plugin Tasks?插件任務(wù)
A Minecraft world contains several different kinds of data. The blocks in the world are fundamentally different from the monsters and animals that wander through it, and some blocks contain data that is more complex than a single block ID. Blocks and entities are organized into chunks, and there is additional data that applies to each entire chunk, and there is also metadata that describes the world dimension that contains these chunks, and that describes the save file as a whole. These tasks will guide you in editing each of these different aspects of the save file.
一個(gè)Minecraft世界包含幾種不同的數(shù)據(jù)。世界里的方塊與在其中漫游的怪物和動(dòng)物有著根本的不同,有些方塊包含的數(shù)據(jù)比單個(gè)方塊ID更復(fù)雜。方塊和實(shí)體被組織成區(qū)塊,并且有附加的數(shù)據(jù)應(yīng)用于每個(gè)區(qū)塊,還有描述世界維度的包含這些區(qū)塊并將保存文件描述為一個(gè)整體的元數(shù)據(jù)。這些任務(wù)將指導(dǎo)您編輯保存文件的這些不同方面。
World Dimensions?世界維度
A Minecraft save file contains several world dimensions, which include the Overworld, The Nether, The End, and any other dimensions added by game mods. MCEdit only allows the user to view and edit one of the world’s dimensions at any time, so plugins will often be given a?dimension?object that refers to the world dimension that the user is currently editing. All of the following tasks will refer to this?dimension?object.
一個(gè)Minecraft保存文件包含多個(gè)世界維度,其中包括超世界、下界、末地以及游戲mod添加的任何其他維度。MCEdit只允許用戶在任何時(shí)候查看和編輯世界維度之一,因此插件通常會(huì)被賦予一個(gè)維度對(duì)象,該對(duì)象引用用戶當(dāng)前正在編輯的世界維度。以下所有任務(wù)都將引用此維度對(duì)象。
Editing Blocks?編輯方塊
Blocks are the defining element of a Minecraft dimension and are the simplest to deal with. Blocks are stored in a three dimensional grid extending from the bottom of the world to the build height - in other words, from?Y=0?to?Y=255. Every position in the grid will have a block. If a block appears to be empty, it will really contain the block?air.
方塊是Minecraft維度的定義元素,是處理起來(lái)最簡(jiǎn)單的。方塊存儲(chǔ)在從世界底部延伸到建造高度的三維網(wǎng)格中——換句話說(shuō),從y=0到y=255。網(wǎng)格中的每個(gè)位置都會(huì)有一個(gè)方塊。如果一個(gè)方塊似乎是空的,它將真正包含空氣方塊。
The position of a block is given by its X, Y, and Z coordinates. The type of the block at that position is given by its identifier, which is a short string of text such as?minecraft:air,?minecraft:stone, or?minecraft:stone[variant=diorite]. Internally, this identifier is stored as a pair of integers. When editing the world, you may use either the string of text or the pair of integers to specify a block type, but it is recommended to use the string of text, for both readability and for compatibility.
方塊的位置由其X、Y和Z坐標(biāo)給出。在該位置的方塊的類型由其標(biāo)識(shí)符給出,該標(biāo)識(shí)符是一個(gè)簡(jiǎn)短的文本字符串,如minecraft:air、minecraft:stone或minecraft:stone[variant=diorite]。在內(nèi)部,這個(gè)標(biāo)識(shí)符存儲(chǔ)為一對(duì)整數(shù)。在編輯世界時(shí),您可以使用文本字符串或整數(shù)對(duì)來(lái)指定方塊類型,但建議使用文本字符串,以提高可讀性和兼容性。
NOTE: When using block types added by mods, you?must?use the text identifier, because the integer IDs will vary from world to world.
注意:當(dāng)使用由mod添加的方塊類型時(shí),您必須使用文本標(biāo)識(shí)符,因?yàn)檎麛?shù)ID將因世界而異。
Alternately, you may pass a BlockType instance. A BlockType instance may be obtained by presenting a block type input to the user (e.g. using an option with?type="blocktype"in a?SimpleCommandPlugin?or by constructing a?BlockTypeButton?yourself). BlockTypes may also be found by looking up a textual or numeric ID in the dimension’s?blocktypes. To wit:
或者,您可以傳遞一個(gè)BlockType實(shí)例。可以通過(guò)向用戶提供方塊類型輸入(例如,在SimpleCommandPlugin中使用帶有type=“blocktype”的選項(xiàng)或自己構(gòu)造BlockTypeButton)來(lái)獲取一個(gè)BlockType實(shí)例。BlockType也可以通過(guò)在維度的blocktypes中查找文本或數(shù)字ID來(lái)找到。也就是說(shuō):
stone =?dimension.blocktypes['minecraft:stone']
granite =?dimension.blocktypes['minecraft:stone[variant=granite]']
cobblestone =?dimension.blocktypes[1] ?# don't do this!
podzol =?dimension.blocktypes[3, 2] ???# don't do this either!
?
dimension.setBlock(0, 0, 0, granite) ??# etc.
To discover which block is at a given position, call?dimension.getBlock, which will return a?BlockType?instance.
要發(fā)現(xiàn)哪個(gè)方塊位于給定位置,請(qǐng)調(diào)用dimension.getBlock,它將返回一個(gè)BlockType實(shí)例。
WorldEditorDimension.getBlock(x,?y,?z)[source]
Returns the block at the given position as an instance of BlockType. This instance will have?id,?meta, and?internalName?attributes that uniquely identify the block’s type, and will have further attributes describing the block’s properties. See?BlockType?for a full description.
以BlockType實(shí)例的形式返回給定位置的方塊。此實(shí)例將具有唯一標(biāo)識(shí)方塊類型的id、meta和internalName屬性,并將具有描述方塊屬性的更多屬性。有關(guān)完整描述,請(qǐng)參見(jiàn)BlockType。
If the given position is outside the generated area of the world, the?minecraft:air?BlockType will be returned.
如果給定位置在世界生成區(qū)域之外,則返回minecraft:air?BlockType。
Parameters:
·x?(int) –
·y?(int) –
·z?(int) –
Returns:
block
Return type:
BlockType
To change the block at a given position, call?dimension.setBlock?
要在給定位置更改方塊,請(qǐng)調(diào)用dimension.setBlock
WorldEditorDimension.setBlock(x,?y,?z,?blocktype)[source]
Changes the block at the given position. The?blocktype?argument may be either a BlockType instance, a textual identifier, a tuple containing a textual identifier and a block metadata value, or a tuple containing a block ID number and a block metadata value.
在給定位置更改方塊。blocktype參數(shù)可以是BlockType實(shí)例、文本標(biāo)識(shí)符、包含文本標(biāo)識(shí)符和方塊元數(shù)據(jù)值的數(shù)組,也可以是包含方塊ID號(hào)和方塊元數(shù)據(jù)值的數(shù)組。
This function will change both the ID value and metadata value at the given position.
此函數(shù)將更改給定位置的ID值和元數(shù)據(jù)值。
It is recommended to pass either a BlockType instance or a textual identifier for readability and compatibility.
為了可讀性和兼容性,建議傳遞BlockType實(shí)例或文本標(biāo)識(shí)符。
Parameters:
x?(int) –
y?(int) –
z?(int) –
blocktype?(BlockType | str |?(str,?int)?|?(int,?int)) –
Returns:
block
Return type:
BlockType
Array-based, high performance variants of these two methods are available. When given parallel arrays of x, y, z coordinates,?dimension.getBlocks?will return an array of the same size containing the block IDs at those coordinates. If requested, it will also return the block metadata values, light values, and/or biome values for those coordinates at the same time.
這兩種方法的基于陣列的高性能變體是可用的。當(dāng)給定x、y、z坐標(biāo)、dimension.getBlocks的并行數(shù)組時(shí),將返回一個(gè)大小相同的數(shù)組,其中包含這些坐標(biāo)處的方塊ID。如果需要,它還將同時(shí)返回這些坐標(biāo)的方塊元數(shù)據(jù)值、燈光值和/或生物群系值。
Likewise,?dimension.setBlocks?may be given parallel arrays of x, y, z coordinates along with arrays of any of the following: block IDs, block metadata values, light values, biome values. To set all coordinates to the same value, you may pass a single value instead of an array.
同樣,可以為dimension.setBlock提供x、y、z坐標(biāo)的并行數(shù)組以及以下任意數(shù)組:方塊ID、方塊元數(shù)據(jù)值、燈光值、生物群系值。要將所有坐標(biāo)設(shè)置為相同的值,可以傳遞單個(gè)值而不是數(shù)組。
WorldEditorDimension.getBlocks(x,?y,?z,?return_Blocks=True,?return_Data=False,?return_BlockLight=False,?return_SkyLight=False,?return_Biomes=False)[source]
WorldEditorDimension.setBlocks(x,?y,?z,?Blocks=None,?Data=None,?BlockLight=None,?SkyLight=None,?Biomes=None,?updateLights=True)[source]
Editing Entities?編輯實(shí)體
Entities are the free-roaming elements of a Minecraft world. They are not bound to the block grid, they may be present at any position in the world, and may even overlap. Animals, monsters, items, and experience orbs are examples of entities.
實(shí)體是Minecraft世界的自由漫游元素。它們沒(méi)有綁定到方塊網(wǎng)格,它們可能存在于世界里的任何位置,甚至可能重疊。動(dòng)物、怪物、物品和經(jīng)驗(yàn)球都是實(shí)體的例子。
Since entities may be at any position, you cannot specify an entity with a single x, y, z coordinate triple. Instead, you may create a BoundingBox (or any other SelectionBox object) and ask MCEdit to find all of the entities within it. You may even ask it to find only the entities which have specific attributes, such as?id="Pig"?to find only Pigs, or?name="Notch"?to find entities named “Notch”. This is all done by calling?dimension.getEntities
由于實(shí)體可能位于任何位置,因此您不能指定具有單一x、y、z三坐標(biāo)的實(shí)體。相反,您可以創(chuàng)建一個(gè)BoundingBox(或任何其他SelectionBox對(duì)象),并要求MCEdit查找其中的所有實(shí)體。您甚至可以要求它僅查找具有特定屬性的實(shí)體,例如id=“pig”僅查找Pigs,或name=“notch”查找名為“Notch”的實(shí)體。這一切都是通過(guò)調(diào)用dimension.getEntities完成的。
WorldEditorDimension.getEntities(selection,?**kw)[source]
Iterate through all entities within the given selection. If any keyword arguments are passed, only yields those entities whose attributes match the given keywords.
遍歷給定選擇中的所有實(shí)體。如果傳遞了任何關(guān)鍵字參數(shù),則只生成那些屬性與給定關(guān)鍵字匹配的實(shí)體。
For example, to iterate through only the zombies in the selection:
例如,要僅遍歷所選內(nèi)容中的僵尸:
for entity in dimension.getEntities(selection, id=”Zombie”):
# do stuff
Parameters:
selection?(SelectionBox) –
kw?(Entity attributes to match exactly.) –
Returns:
entities
Return type:
Iterator[EntityRef]
It is important to know that this function only returns an iterator over the found entities. If you assign one of these entities to another variable (or put it into a container such as a?list?or?dict) then that entity will keep its containing chunk loaded, which may possibly lead to out-of-memory errors. Thus, it is best to simply modify the entities in-place while iterating through them rather than hold references to them.
重要的是要知道這個(gè)函數(shù)只返回找到實(shí)體的迭代器。如果將這些實(shí)體中的一個(gè)分配給另一個(gè)變量(或?qū)⑵浞湃肴萜?/span>,如list或dict),則該實(shí)體將保持包含它的區(qū)塊被加載,這可能導(dǎo)致“內(nèi)存不足”錯(cuò)誤。因此,最好是在迭代實(shí)體時(shí)簡(jiǎn)單地在適當(dāng)?shù)奈恢眯薷乃鼈?,而不是保留?duì)它們的引用。
The entities are returned as instances of?EntityRef. An?EntityRef?is a wrapper around the underlying NBT Compound Tag that contains the entity’s data. The?EntityRef?allows you to change the values of the entity’s attributes without having to deal with the individual tags and tag types that represent those attributes.
實(shí)體作為EntityRef的實(shí)例返回。EntityRef是包含實(shí)體數(shù)據(jù)的基礎(chǔ)NBT復(fù)合標(biāo)記的包裝器。EntityRef允許您更改實(shí)體屬性的值,而不必處理表示這些屬性的單個(gè)標(biāo)記和標(biāo)記類型。
In other words, instead of writing:
換句話說(shuō),不是寫(xiě):
entity["Name"] =?nbt.TAG_String("codewarrior0")
You only have to write:
你只需要寫(xiě):
entity.Name =?"codewarrior0"
However, it may occasionally be useful to access the entity’s NBT tags directly. This can be done using the?entity.raw_tag?attribute. After modifying the?raw_tag, you must always mark the entity as dirty by doing?entity.dirty?=?True?to ensure your changes are saved:
但是,有時(shí)直接訪問(wèn)實(shí)體的NBT標(biāo)記可能很有用。這可以使用entity.raw_tag屬性來(lái)完成。修改raw_tag后,必須始終通過(guò)執(zhí)行entity.dirty=True將實(shí)體標(biāo)記為 dirty ,以確保更改被保存:
import?nbttag =?entity.raw_tag()tag["Name"] =?nbt.TAG_String("codewarrior0")entity.dirty =?True
Creating entities?創(chuàng)建實(shí)體
TODO: Describe creating entities, either by calling?dimension.worldEditor.EntityRef.create(entityID)?or by crafting the entity’s tag by hand.
TODO:通過(guò)調(diào)用dimension.worldEditor.EntityRef.create(entityID)或手工創(chuàng)建實(shí)體的標(biāo)記來(lái)描述創(chuàng)建實(shí)體。