Lab Exercise 4
1.?(簡答題)
Students are required to submit your solutions?before 0:00am on November 14th. You should only upload two files (do not zip), your?notebook ".ipynb" file?and?PDF file generated after notebook execution.?
1. Add random noises to an image
1) Use skimage.util.random_noise (or other equivalent method functions) to add random noises?

?to any image, and display the resulting image.
2) Add random noises to the original image each time to generate a noisy image, repeat M times, accumulate all M noisy?images, and then do average. which is
Show average pictures for M=50, 100, 150, 200 respectively.
3) What conclusions can you draw from the results?
2. A ring-enhancing lesion is an abnormal radiologic sign on MRI or CT scans obtained using radiocontrast. On the image, there is an area of decreased density (see radiodensity) surrounded by a bright rim from concentration of the enhancing contrast dye. This enhancement may represent breakdown of the blood-brain barrier and the development of an inflammatory capsule. This can be a finding in numerous disease states. In the brain, it can occur with an early brain abscess as well as in Nocardia infections associated with lung cavitary lesions. In patients with HIV, the major differential is between CNS lymphoma and CNS toxoplasmosis, with CT imaging being the appropriate next step to differentiate between the two conditions.
??
The following is a head CT scan of a patient with a ring-enhanced lesion:
1) Design and implement a pseudocolor shading method to colorize head CT scans (grayscale images). The results can be similar to those shown in the example or other better methods (which can highlight the lesion area best).
2) Explain your pseudocolor shading method.
3) If you are required to segment the lesion area, what problems do you think you will encounter? Discuss how you would approach this problem? (Competent students may try to implement your idea, not asking for a perfect answer)
提供的圖片:
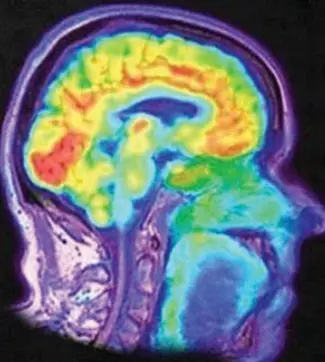
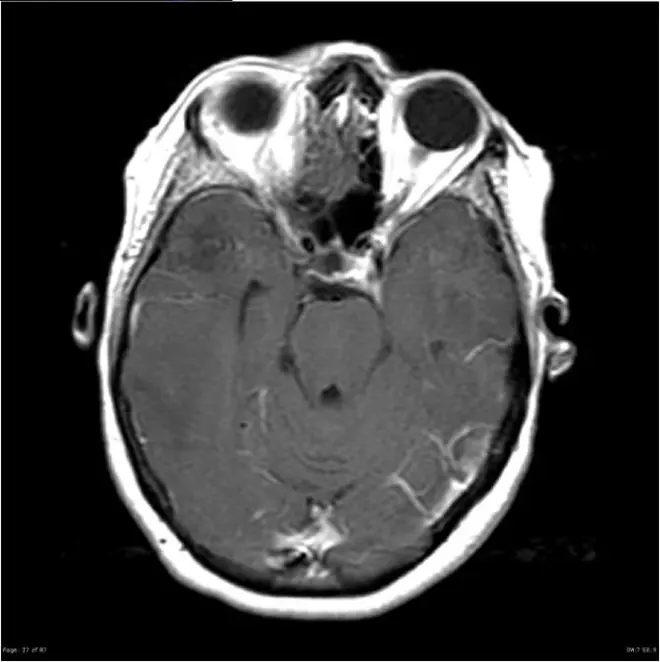
作答:
import skimage?
from skimage import util
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image
import CV2
import random
img = Image.open('image/original.png')
img = np.array(img)
noise_gs_img = util.random_noise(img,mode='gaussian')
plt.subplot(1,2,1)
plt.title('original')
plt.imshow(img)
plt.subplot(1,2,2)
plt.title('gaussian')
plt.imshow(noise_gs_img)
plt.show()
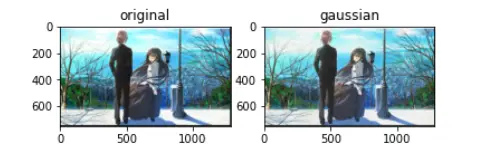
Add random noises to the original image each time to generate a noisy image, repeat M times, accumulate all M noisy images, and then do average.
#展示圖片函數(shù)
def show(img):
? ? if img.ndim==2:
? ? ? ? plt.imshow(img,cmap='gray')
? ? else:
? ? ? ? plt.imshow(CV2.cvtColor(img,CV2.COLOR_BGR2RGB))
def gaussian_noise(image, count,mean=0.1, sigma=0.3):
? ? """
? ? 添加高斯噪聲
? ? :param image:原圖
? ? :param mean:均值
? ? :param sigma:標(biāo)準(zhǔn)差 值越大,噪聲越多
? ? :return:噪聲處理后的圖片
? ? """
? ? sum=0.000
? ? image = np.asarray(image / 255, dtype=np.float32)? # 圖片灰度標(biāo)準(zhǔn)化
? ? for i in range(count):
? ? ? ? noise = np.random.normal(mean, sigma, image.shape).astype(dtype=np.float32)? # 產(chǎn)生高斯噪聲
? ? ? ? sum=sum+noise
#? ? ?print(sum)
? ? ? ??
? ? noise=sum/(count*1.00)
#? ? ?print(noise)
? ? output = image + noise? # 將噪聲和圖片疊加
? ? output = np.clip(output, 0, 1)
? ? output = np.uint8(output * 255)
? ? return output
#50
image = CV2.imread("image/original.png")
out1 = gaussian_noise(image.copy(),50)
show(out1)
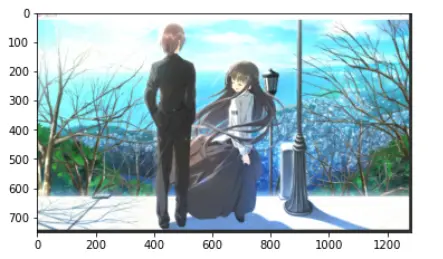
#100
out2= gaussian_noise(image.copy(),100)
show(out2)
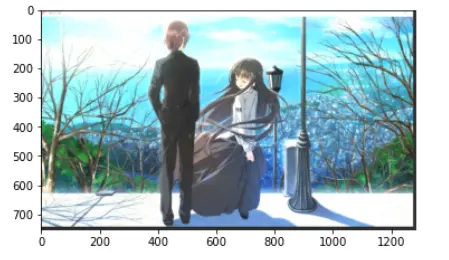
#150
out3= gaussian_noise(image.copy(),150)
show(out3)
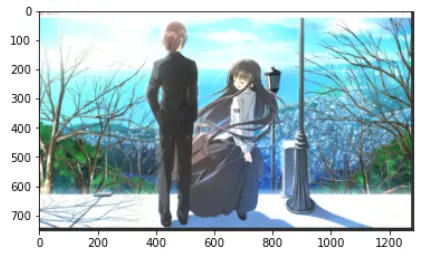
#200
out4= gaussian_noise(image.copy(),200)
show(out4)
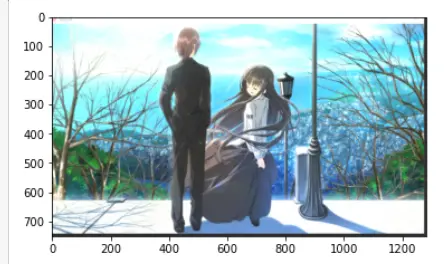
1) Design and implement a pseudocolor shading method to colorize head CT scans (grayscale images). The results can be similar to those shown in the example or other better methods (which can highlight the lesion area best)
im_gray = CV2.imread("image/1.png", CV2.IMREAD_GRAYSCALE)
im_color = CV2.applyColorMap(im_gray, CV2.COLORMAP_JET)
show(im_color)
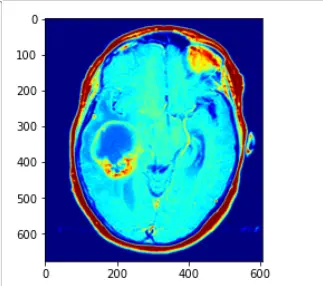
im_gray = CV2.imread("image/2.png", CV2.IMREAD_GRAYSCALE)
im_color = CV2.applyColorMap(im_gray, CV2.COLORMAP_JET)
show(im_color)
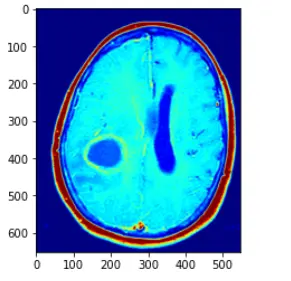
im_gray = CV2.imread("image/3.png", CV2.IMREAD_GRAYSCALE)
im_color = CV2.applyColorMap(im_gray, CV2.COLORMAP_JET)
show(im_color)
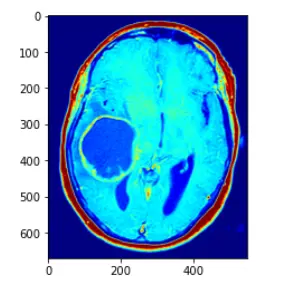
im_gray = CV2.imread("image/10.png", CV2.IMREAD_GRAYSCALE)
im_color = CV2.applyColorMap(im_gray, CV2.COLORMAP_JET)
show(im_color)
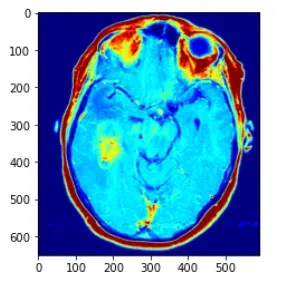
2) Explain your pseudocolor shading method.
解釋如下:直接使用CV2.applyColorMap 方法,applyColorMap為偽彩色函數(shù),建立在灰度圖的前提下使用,CV2.COLORMAP_JET采用染色模式,灰度值越高則表現(xiàn)的顏色越為紅色,灰度值越低的話,變現(xiàn)為藍(lán)色
在此中間的灰度值漸變?yōu)樗{(lán)紅之間的黃,綠等
而opencv中的applyColor函數(shù)其實現(xiàn)方法為對于遍歷灰度圖像獲取每個像素點的灰度值,然后對灰度值進行層級劃分,例如0~63 藍(lán)色,64~127 紫色,128~191黃色,192~255紅色
進行一個劃分,然后對層級進行一個人,r,g,b的轉(zhuǎn)化,設(shè)置相應(yīng)的三通道值即可,轉(zhuǎn)化為三通道圖像,即可得到偽彩色圖像處理的方法
3) If you are required to segment the lesion area, what problems do you think you will encounter? Discuss how you would approach this problem? (Competent students may try to implement your idea, not asking for a perfect answer)
我認(rèn)為首先確定好哪里是受損傷的部位是一個問題,然后如何對受傷部位進行分割也是一個問題,我的話,在已知損傷部位的情況下,對ct圖使用較深顏色筆進行邊緣大致的畫線圈畫
然后用圈畫之后,畫出大致的輪廓,在偽彩色處理之后,損傷部位被清晰標(biāo)注圈出來,這樣子不就已經(jīng)劃分出來損傷和被損傷的部位了
(有些代碼是百度的,csdn中的,畢竟不是造輪子,相互看看改改就能實現(xiàn),但是如果想要真的了解學(xué)懂,還是慢慢看著來)