2023.3.30-Java-接口和抽象類
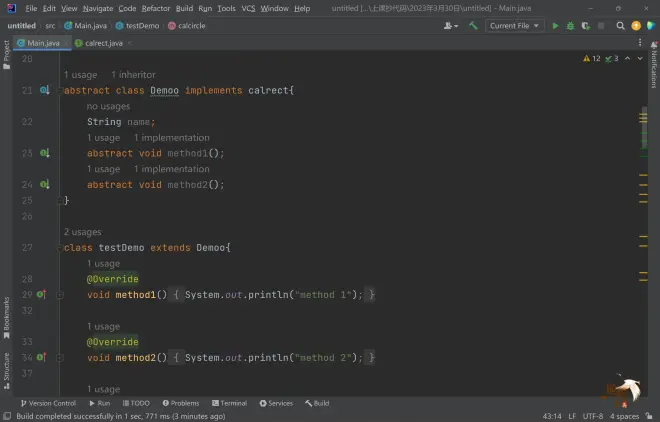
Main.java
public class Main {
? ?public static void main(String[] args) {
? ? ? ?demo sample1 = new demo();
? ? ? ?sample1.list();
? ? ? ?sample1.print();
? ? ? ?testDemo sample2 = new testDemo();
? ? ? ?sample2.method1();
? ? ? ?sample2.method2();
? ? ? ?System.out.println(sample2.sqrt(3.14)+sample2.calcircle()+sample2.calarea());
? ? ? ?Crocodile sample3 = new Crocodile();
? ? ? ?sample3.waterDo();
? ? ? ?Outer outer = new Outer();
? ? ? ?outer.print();
? ?}
}
abstract class Demoo implements calrect{
? ?String name;
? ?abstract void method1();
? ?abstract void method2();
}
class testDemo extends Demoo{
? ?@Override
? ?void method1() {
? ? ? ?System.out.println("method 1");
? ?}
? ?@Override
? ?void method2() {
? ? ? ?System.out.println("method 2");
? ?}
? ?@Override
? ?public int calarea() {
? ? ? ?return 0;
? ?}
? ?@Override
? ?public double calcircle() {
? ? ? ?return 0;
? ?}
}
interface OnEarthFunc{
? ?void earthDo();
}
interface InWaterFunc{
? ?void waterDo();
}
interface OnAirFunc{
? ?void airDo();
}
class Vulture implements OnEarthFunc, OnAirFunc{
? ?public void earthDo(){}
? ?public void airDo(){}
}
class Crocodile implements OnEarthFunc, InWaterFunc{
? ?public void earthDo(){System.out.println("Crocodile on earth!");}
? ?public void waterDo(){System.out.println("Crocodile in water!");}
}
interface func{
? ?void print();
}
interface show extends func{
? ?void list();
}
class demo implements show{
? ?public void print(){
? ? ? ?System.out.println("print");
? ?}
? ?public void list(){
? ? ? ?System.out.println("list");
? ?}
}
class Outer{
? ?private int index=10;
? ?class Inner{
? ? ? ?private int index=20;
? ? ? ?void print() {
? ? ? ? ? ?int index = 30;
? ? ? ? ? ?System.out.println(this);
? ? ? ? ? ?System.out.println(Outer.this);
? ? ? ? ? ?System.out.println(index);
? ? ? ? ? ?System.out.println(this.index);
? ? ? ? ? ?System.out.println(Outer.this.index);
? ? ? ?}
? ?}
? ?void print(){
? ? ? ?Inner inner = new Inner();
? ? ? ?inner.print();
? ?}
}
/*
抽象類給所有由它派生出來的類創(chuàng)建一個(gè)公共的接口。
抽象類和接口,引入是為了解決沒有多繼承的問題。
抽象類不能被實(shí)例化。
一個(gè)類通過繼承接口,以繼承接口的抽象方法。
接口像一個(gè)插銷,寫在以接口名命名的.java文件里,然后,插到我的main類里面。
接口的變量,默認(rèn)為public static final的。接口的方法都是public abstract的,只有說明沒有定義。
不過,從java 8開始,允許給接口添加一個(gè)非抽象的方法實(shí)現(xiàn)。不過要使用default關(guān)鍵字。
Java 9可以:常量、抽象方法、默認(rèn)方法、靜態(tài)方法、私有方法、私有靜態(tài)方法。
為了把接口插進(jìn)類里面,類必須聲明實(shí)現(xiàn)接口(implements),按指定參數(shù)個(gè)數(shù)類型返回。
接口的實(shí)現(xiàn):
1. 靜態(tài)方法,只能使用接口名稱調(diào)用;
2. default方法屬于實(shí)現(xiàn)接口的類的對(duì)象方法;
……
一般的應(yīng)用里,最頂層的是接口,然后是抽象類實(shí)現(xiàn)接口,最后才到具體類實(shí)現(xiàn)。
接口--implements-->抽象類--extends-->具體類
final修飾符的作用:防止方法的重寫或者類的繼承。
內(nèi)部類。
匿名類(創(chuàng)建一個(gè)類的對(duì)象,無需用到名字)直接new classname(){};。
可以用匿名類實(shí)現(xiàn)接口,new interfacename(){};
匿名內(nèi)部類的編譯后命名規(guī)則:outerclass.$n.class,n從1開始。
用一次就丟棄的類,所以不需要名字(哭)。
Java API提供了很多類和方法,直接調(diào)用(e. g. ……)
·Math類
包(需要import)
·java.lang
·java.io
·java.util
*/

calrect.java
public class Main {
? ?public static void main(String[] args) {
? ? ? ?demo sample1 = new demo();
? ? ? ?sample1.list();
? ? ? ?sample1.print();
? ? ? ?testDemo sample2 = new testDemo();
? ? ? ?sample2.method1();
? ? ? ?sample2.method2();
? ? ? ?System.out.println(sample2.sqrt(3.14)+sample2.calcircle()+sample2.calarea());
? ? ? ?Crocodile sample3 = new Crocodile();
? ? ? ?sample3.waterDo();
? ? ? ?Outer outer = new Outer();
? ? ? ?outer.print();
? ?}
}
abstract class Demoo implements calrect{
? ?String name;
? ?abstract void method1();
? ?abstract void method2();
}
class testDemo extends Demoo{
? ?@Override
? ?void method1() {
? ? ? ?System.out.println("method 1");
? ?}
? ?@Override
? ?void method2() {
? ? ? ?System.out.println("method 2");
? ?}
? ?@Override
? ?public int calarea() {
? ? ? ?return 0;
? ?}
? ?@Override
? ?public double calcircle() {
? ? ? ?return 0;
? ?}
}
interface OnEarthFunc{
? ?void earthDo();
}
interface InWaterFunc{
? ?void waterDo();
}
interface OnAirFunc{
? ?void airDo();
}
class Vulture implements OnEarthFunc, OnAirFunc{
? ?public void earthDo(){}
? ?public void airDo(){}
}
class Crocodile implements OnEarthFunc, InWaterFunc{
? ?public void earthDo(){System.out.println("Crocodile on earth!");}
? ?public void waterDo(){System.out.println("Crocodile in water!");}
}
interface func{
? ?void print();
}
interface show extends func{
? ?void list();
}
class demo implements show{
? ?public void print(){
? ? ? ?System.out.println("print");
? ?}
? ?public void list(){
? ? ? ?System.out.println("list");
? ?}
}
class Outer{
? ?private int index=10;
? ?class Inner{
? ? ? ?private int index=20;
? ? ? ?void print() {
? ? ? ? ? ?int index = 30;
? ? ? ? ? ?System.out.println(this);
? ? ? ? ? ?System.out.println(Outer.this);
? ? ? ? ? ?System.out.println(index);
? ? ? ? ? ?System.out.println(this.index);
? ? ? ? ? ?System.out.println(Outer.this.index);
? ? ? ?}
? ?}
? ?void print(){
? ? ? ?Inner inner = new Inner();
? ? ? ?inner.print();
? ?}
}
/*
抽象類給所有由它派生出來的類創(chuàng)建一個(gè)公共的接口。
抽象類和接口,引入是為了解決沒有多繼承的問題。
抽象類不能被實(shí)例化。
一個(gè)類通過繼承接口,以繼承接口的抽象方法。
接口像一個(gè)插銷,寫在以接口名命名的.java文件里,然后,插到我的main類里面。
接口的變量,默認(rèn)為public static final的。接口的方法都是public abstract的,只有說明沒有定義。
不過,從java 8開始,允許給接口添加一個(gè)非抽象的方法實(shí)現(xiàn)。不過要使用default關(guān)鍵字。
Java 9可以:常量、抽象方法、默認(rèn)方法、靜態(tài)方法、私有方法、私有靜態(tài)方法。
為了把接口插進(jìn)類里面,類必須聲明實(shí)現(xiàn)接口(implements),按指定參數(shù)個(gè)數(shù)類型返回。
接口的實(shí)現(xiàn):
1. 靜態(tài)方法,只能使用接口名稱調(diào)用;
2. default方法屬于實(shí)現(xiàn)接口的類的對(duì)象方法;
……
一般的應(yīng)用里,最頂層的是接口,然后是抽象類實(shí)現(xiàn)接口,最后才到具體類實(shí)現(xiàn)。
接口--implements-->抽象類--extends-->具體類
final修飾符的作用:防止方法的重寫或者類的繼承。
內(nèi)部類。
匿名類(創(chuàng)建一個(gè)類的對(duì)象,無需用到名字)直接new classname(){};。
可以用匿名類實(shí)現(xiàn)接口,new interfacename(){};
匿名內(nèi)部類的編譯后命名規(guī)則:outerclass.$n.class,n從1開始。
用一次就丟棄的類,所以不需要名字(哭)。
Java API提供了很多類和方法,直接調(diào)用(e. g. ……)
·Math類
包(需要import)
·java.lang
·java.io
·java.util
*/

終端輸出
D:\SoftwareSet\Java\JDK19\jdk-19.0.2\bin\java.exe "-javaagent:D:\SoftwareSet\Java\javaIDE(jetbrains)\installPath\IntelliJ IDEA Community Edition 2022.3.2\lib\idea_rt.jar=40628:D:\SoftwareSet\Java\javaIDE(jetbrains)\installPath\IntelliJ IDEA Community Edition 2022.3.2\bin" -Dfile.encoding=UTF-8 -Dsun.stdout.encoding=UTF-8 -Dsun.stderr.encoding=UTF-8 -classpath D:\AllData\mess\2023_Java_lecture\上課抄代碼\2023年3月30日\untitled\out\production\untitled Main
list
method 1
method 2
1.772004514666935
Crocodile in water!
Outer$Inner@10f87f48
Outer@b4c966a
30
20
10
Process finished with exit code 0