基于ssm的數(shù)據(jù)學院教務(wù)管理系統(tǒng)設(shè)計與實現(xiàn)-計算機畢業(yè)設(shè)計源碼+LW文檔
開發(fā)語言:Java
框架:ssm
JDK版本:JDK1.8
服務(wù)器:tomcat7
數(shù)據(jù)庫:mysql 5.7(一定要5.7版本)
數(shù)據(jù)庫工具:Navicat11
開發(fā)軟件:eclipse/myeclipse/idea
Maven包:Maven3.3.9
瀏覽器:谷歌瀏覽器
關(guān)鍵代碼:
package com.dao;
import com.entity.KechengzuoyeEntity;
import com.baomidou.mybatisplus.mapper.BaseMapper;
import java.util.List;
import java.util.Map;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.baomidou.mybatisplus.plugins.pagination.Pagination;
import org.apache.ibatis.annotations.Param;
import com.entity.vo.KechengzuoyeVO;
import com.entity.view.KechengzuoyeView;
/**
?* 課程作業(yè)
?*?
?* @author?
?* @email?
?* @date 2022-03-31 09:46:16
?*/
public interface KechengzuoyeDao extends BaseMapper<KechengzuoyeEntity> {
List<KechengzuoyeVO> selectListVO(@Param("ew") Wrapper<KechengzuoyeEntity> wrapper);
KechengzuoyeVO selectVO(@Param("ew") Wrapper<KechengzuoyeEntity> wrapper);
List<KechengzuoyeView> selectListView(@Param("ew") Wrapper<KechengzuoyeEntity> wrapper);
List<KechengzuoyeView> selectListView(Pagination page,@Param("ew") Wrapper<KechengzuoyeEntity> wrapper);
KechengzuoyeView selectView(@Param("ew") Wrapper<KechengzuoyeEntity> wrapper);
}
? ? /**
? ? ?* 后端列表
? ? ?*/
? ? @RequestMapping("/page")
? ? public R page(@RequestParam Map<String, Object> params,XuankexinxiEntity xuankexinxi,?
HttpServletRequest request){
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("jiaoshi")) {
xuankexinxi.setJiaoshigonghao((String)request.getSession().getAttribute("username"));
}
if(tableName.equals("xuesheng")) {
xuankexinxi.setXuehao((String)request.getSession().getAttribute("username"));
}
? ? ? ? EntityWrapper<XuankexinxiEntity> ew = new EntityWrapper<XuankexinxiEntity>();
PageUtils page = xuankexinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xuankexinxi), params), params));
? ? ? ? return R.ok().put("data", page);
? ? }
? ??
? ? /**
? ? ?* 前端列表
? ? ?*/
@IgnoreAuth
? ? @RequestMapping("/list")
? ? public R list(@RequestParam Map<String, Object> params,XuankexinxiEntity xuankexinxi,?
HttpServletRequest request){
? ? ? ? EntityWrapper<XuankexinxiEntity> ew = new EntityWrapper<XuankexinxiEntity>();
PageUtils page = xuankexinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xuankexinxi), params), params));
? ? ? ? return R.ok().put("data", page);
? ? }
/**
? ? ?* 列表
? ? ?*/
? ? @RequestMapping("/lists")
? ? public R list( XuankexinxiEntity xuankexinxi){
? ? ? ? EntityWrapper<XuankexinxiEntity> ew = new EntityWrapper<XuankexinxiEntity>();
? ? ? ew.allEq(MPUtil.allEQMapPre( xuankexinxi, "xuankexinxi"));?
? ? ? ? return R.ok().put("data", xuankexinxiService.selectListView(ew));
? ? }
/**
? ? ?* 查詢
? ? ?*/
? ? @RequestMapping("/query")
? ? public R query(XuankexinxiEntity xuankexinxi){
? ? ? ? EntityWrapper< XuankexinxiEntity> ew = new EntityWrapper< XuankexinxiEntity>();
? ew.allEq(MPUtil.allEQMapPre( xuankexinxi, "xuankexinxi"));?
XuankexinxiView xuankexinxiView =? xuankexinxiService.selectView(ew);
return R.ok("查詢選課信息成功").put("data", xuankexinxiView);
? ? }
? ? /**
? ? ?* 后端詳情
? ? ?*/
? ? @RequestMapping("/info/{id}")
? ? public R info(@PathVariable("id") Long id){
? ? ? ? XuankexinxiEntity xuankexinxi = xuankexinxiService.selectById(id);
? ? ? ? return R.ok().put("data", xuankexinxi);
? ? }
? ? /**
? ? ?* 前端詳情
? ? ?*/
@IgnoreAuth
? ? @RequestMapping("/detail/{id}")
? ? public R detail(@PathVariable("id") Long id){
? ? ? ? XuankexinxiEntity xuankexinxi = xuankexinxiService.selectById(id);
? ? ? ? return R.ok().put("data", xuankexinxi);
? ? }
? ??
? ? /**
? ? ?* 后端保存
? ? ?*/
? ? @RequestMapping("/save")
? ? public R save(@RequestBody XuankexinxiEntity xuankexinxi, HttpServletRequest request){
? ? xuankexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
? ? //ValidatorUtils.validateEntity(xuankexinxi);
? ? ? ? xuankexinxiService.insert(xuankexinxi);
? ? ? ? return R.ok();
? ? }
? ??
? ? /**
? ? ?* 前端保存
? ? ?*/
? ? @RequestMapping("/add")
? ? public R add(@RequestBody XuankexinxiEntity xuankexinxi, HttpServletRequest request){
? ? xuankexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
? ? //ValidatorUtils.validateEntity(xuankexinxi);
? ? ? ? xuankexinxiService.insert(xuankexinxi);
? ? ? ? return R.ok();
? ? }
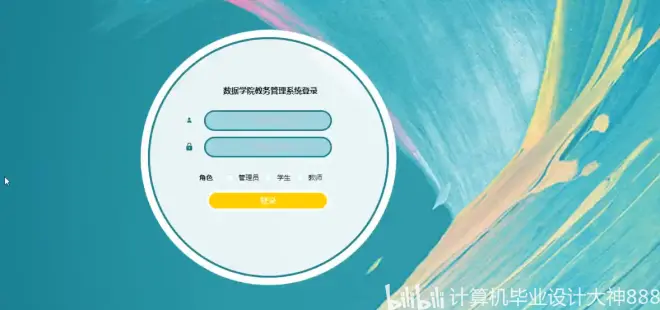
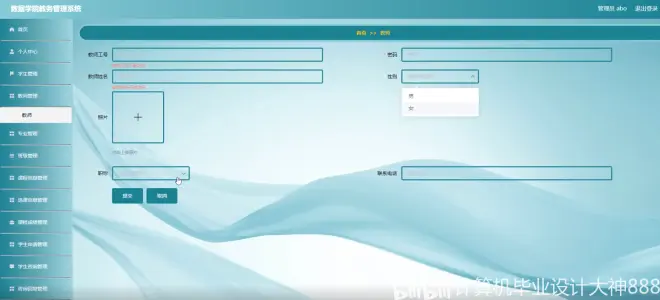
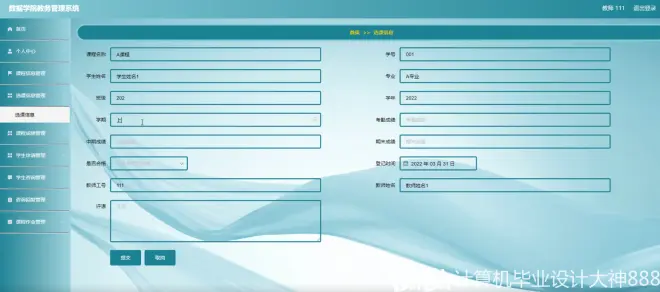
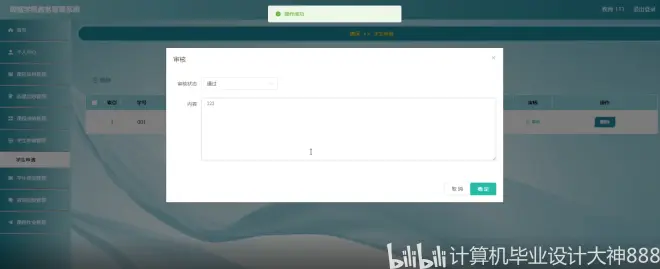