新手使用mongodb
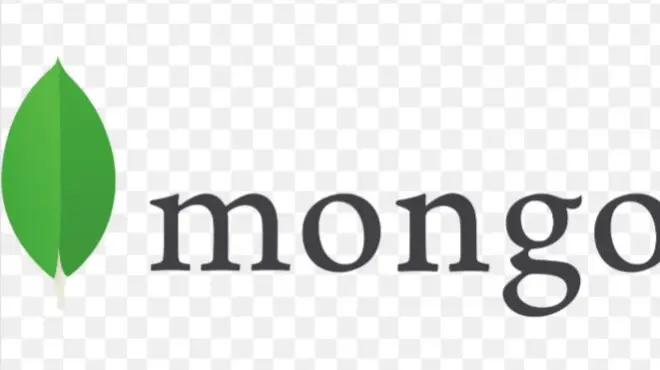
# mongo
mongo適用于大數(shù)據(jù)量,高并發(fā),輕量事物型系統(tǒng)
## devops
### 創(chuàng)建管理員賬戶
1. 以非授權(quán)模式啟動(dòng)mongod
```sh
mongod --dbpath /usr/local/var/mongodb --logpath /usr/local/var/log/mongodb/mongo.log --fork
```
2. 創(chuàng)建管理用戶admin
`使用mongosh連接進(jìn)去`
```bash
test> use admin
switched to db admin
admin> db.createUser({
... ? ? user:"admin",
... ? ? pwd:"admin123456",
... ? ? roles:[{role:"userAdminAnyDatabase",db:"admin"}]
... });
{ ok: 1 }
#查看用戶,另外還可以使用db.system.users.find()
admin> show users
[
{
_id: 'admin.admin',
userId: UUID("554560b1-e47b-44d9-8d3a-5f7ae5c933eb"),
user: 'admin',
db: 'admin',
roles: [ { role: 'userAdminAnyDatabase', db: 'admin' } ],
mechanisms: [ 'SCRAM-SHA-1', 'SCRAM-SHA-256' ]
}
]
#退出來(lái)
admin> quit
```
3. 停止數(shù)據(jù)庫(kù)
```sh
killall `pidof mongod`
```
### 創(chuàng)建業(yè)務(wù)賬戶
1. 以授權(quán)模式啟動(dòng)
```bash
```sh
mongod --auth --dbpath /usr/local/var/mongodb --logpath /usr/local/var/log/mongodb/mongo.log --fork
```
```
2. 使用admin登錄
```sh
# 可直接使用mongosh進(jìn)去后使用db.auth('admin','admin123456')再認(rèn)證
mongosh -u admin -p
```
3. 創(chuàng)建業(yè)務(wù)用戶
```sh
test> use biz
biz> db.createUser({user:"webopr",pwd:"123456",roles:[{role:"readWrite",db:"biz"}]})
{ ok: 1 }
```
4. 使用webopr登錄
```sh
test> use biz
switched to db biz
biz> db.auth('webopr','123456')
{ ok: 1 }
biz>
biz> show tables;
# 插入數(shù)據(jù)
biz> db.users.insertOne({uid:0,age:19,name:"EdgeCross",uin:"skyhawk"})
{
acknowledged: true,
insertedId: ObjectId("6438fa7dda4faacf8d683e85")
}
# 查詢剛插入的數(shù)據(jù)
biz> db.users.find();
[
{
_id: ObjectId("6438fa7dda4faacf8d683e85"),
uid: 0,
age: 19,
name: 'EdgeCross',
uin: 'skyhawk'
}
]
```
## 客戶端編碼
### options包
在options包, 可用來(lái)設(shè)置客戶端選項(xiàng)(ClientOptions)。
```go
// 新建客戶端選項(xiàng)
func Client()*ClientOptions
// 連接字符串,DSN
func (c *ClientOptions) GetURI() string
func (c *ClientOptions) ApplyURI(uri string) *ClientOptions
// 參數(shù)設(shè)置... 一些參數(shù)可通過(guò)uri參數(shù)設(shè)置
```
#### 連接字符串
```go
"mongodb://user:password@host:port/db?param1=value1¶m2=value2"
```
### mongo包
#### Client
一個(gè)Client對(duì)象是一個(gè)連接池,它是并發(fā)安全的。它可以自動(dòng)地打開和關(guān)閉連接,并維護(hù)一個(gè)空閑的連接池。
連接池的配置選項(xiàng)可通過(guò)options.ClientOptions配置。
```go
// 調(diào)用NewClient創(chuàng)建Client對(duì)象,并調(diào)用Connect方法初始化它。
func Connect(ctx context.Context, opts ...*options.ClientOptions) (*Client, error)
// 創(chuàng)建client對(duì)象
func NewClient(opts ...*options.ClientOptions) (*Client, error)
// 通過(guò)啟動(dòng)后臺(tái)監(jiān)聽協(xié)程來(lái)初始化Client,如果c通過(guò)NewClient函數(shù)創(chuàng)建,該方法必須在client使用之前被調(diào)用。
func (c *Client) Connect(ctx context.Context) error
// 關(guān)閉網(wǎng)絡(luò)連接
func (c *Client) Disconnect(ctx context.Context) error
// Ping 用于驗(yàn)證連接是否建立起來(lái)了(與數(shù)據(jù)庫(kù)的網(wǎng)絡(luò)連接)
func (c *Client) Ping(ctx context.Context, rp *readpref.ReadPref) error
// 根據(jù)給定的選項(xiàng)創(chuàng)建一個(gè)新的會(huì)話,調(diào)用StartSession是線程安全的,但是返回的session不是線程安全的。
func (c *Client) StartSession(opts ...*options.SessionOptions) (Session, error)
// 獲取數(shù)據(jù)庫(kù)句柄
func (c *Client) Database(name string, opts ...*options.DatabaseOptions) *Database
func (c *Client) ListDatabases(ctx context.Context, filter interface{}, opts ...*options.ListDatabasesOptions)
func (c *Client) ListDatabaseNames(ctx context.Context, filter interface{}, opts ...*options.ListDatabasesOptions) ([]string, error)
// session
func WithSession(ctx context.Context, sess Session, fn func(SessionContext) error) error
func (c *Client) UseSession(ctx context.Context, fn func(SessionContext) error) error
func (c *Client) UseSessionWithOptions(ctx context.Context, opts *options.SessionOptions, fn func(SessionContext) error) error
func (c *Client) NumberSessionsInProgress() int
// changestream
func (c *Client) Watch(ctx context.Context, pipeline interface{},
opts ...*options.ChangeStreamOptions) (*ChangeStream, error)
```
#### Database
描述一個(gè)mongoDB數(shù)據(jù)庫(kù),是線程安全的。
```go
// 獲得一個(gè)集合句柄
func (db *Database) Collection(name string, opts ...*options.CollectionOptions) *Collection
// 在數(shù)據(jù)庫(kù)上執(zhí)行一個(gè)聚合命令
func (db *Database) Aggregate(ctx context.Context, pipeline interface{},
opts ...*options.AggregateOptions) (*Cursor, error)
// 執(zhí)行命令
func (db *Database) RunCommand(ctx context.Context, runCommand interface{}, opts ...*options.RunCmdOptions) *SingleResult
func (db *Database) RunCommandCursor(ctx context.Context, runCommand interface{}, opts ...*options.RunCmdOptions) (*Cursor, error)
// 在數(shù)據(jù)庫(kù)上刪除
func (db *Database) Drop(ctx context.Context) error
// 列出集合
func (db *Database) ListCollectionSpecifications(ctx context.Context, filter interface{},
opts ...*options.ListCollectionsOptions) ([]*CollectionSpecification, error)
func (db *Database) ListCollections(ctx context.Context, filter interface{}, opts ...*options.ListCollectionsOptions) (*Cursor, error)
// 數(shù)據(jù)庫(kù)ChangeStream
func (db *Database) Watch(ctx context.Context, pipeline interface{},
opts ...*options.ChangeStreamOptions) (*ChangeStream, error)
// 顯示的創(chuàng)建集合,如果集合存在,返回錯(cuò)誤
func (db *Database) CreateCollection(ctx context.Context, name string, opts ...*options.CreateCollectionOptions) error
// 創(chuàng)建視圖,視圖是干什么的?
func (db *Database) CreateView(ctx context.Context, viewName, viewOn string, pipeline interface{},
opts ...*options.CreateViewOptions) error
```
#### Collection
描述一個(gè)mongoDB集合,是線程安全的。
```go
// 執(zhí)行一個(gè)批量寫操作
func (coll *Collection) BulkWrite(ctx context.Context, models []WriteModel,
opts ...*options.BulkWriteOptions) (*BulkWriteResult, error)
// 插入
func (coll *Collection) InsertOne(ctx context.Context, document interface{},
opts ...*options.InsertOneOptions) (*InsertOneResult, error)
func (coll *Collection) InsertMany(ctx context.Context, documents []interface{},
opts ...*options.InsertManyOptions) (*InsertManyResult, error)
// 刪除
func (coll *Collection) DeleteOne(ctx context.Context, filter interface{},
opts ...*options.DeleteOptions) (*DeleteResult, error)
func (coll *Collection) DeleteMany(ctx context.Context, filter interface{},
opts ...*options.DeleteOptions) (*DeleteResult, error)
// 更新
func (coll *Collection) UpdateByID(ctx context.Context, id interface{}, update interface{},
opts ...*options.UpdateOptions) (*UpdateResult, error)
func (coll *Collection) UpdateOne(ctx context.Context, filter interface{}, update interface{},
opts ...*options.UpdateOptions) (*UpdateResult, error)
func (coll *Collection) UpdateMany(ctx context.Context, filter interface{}, update interface{},
opts ...*options.UpdateOptions) (*UpdateResult, error)
func (coll *Collection) ReplaceOne(ctx context.Context, filter interface{},
replacement interface{}, opts ...*options.ReplaceOptions) (*UpdateResult, error)
// 執(zhí)行聚合命令
func (coll *Collection) Aggregate(ctx context.Context, pipeline interface{},
opts ...*options.AggregateOptions) (*Cursor, error)
// 統(tǒng)計(jì)文檔數(shù)量
func (coll *Collection) CountDocuments(ctx context.Context, filter interface{},
opts ...*options.CountOptions) (int64, error)
func (coll *Collection) EstimatedDocumentCount(ctx context.Context,
opts ...*options.EstimatedDocumentCountOptions) (int64, error)
func (coll *Collection) Distinct(ctx context.Context, fieldName string, filter interface{},
opts ...*options.DistinctOptions) ([]interface{}, error)
// 查詢
func (coll *Collection) Find(ctx context.Context, filter interface{},
opts ...*options.FindOptions) (cur *Cursor, err error)
func (coll *Collection) FindOne(ctx context.Context, filter interface{},
opts ...*options.FindOneOptions) *SingleResult
func (coll *Collection) FindOneAndDelete(ctx context.Context, filter interface{},
opts ...*options.FindOneAndDeleteOptions) *SingleResult
func (coll *Collection) FindOneAndReplace(ctx context.Context, filter interface{},
replacement interface{}, opts ...*options.FindOneAndReplaceOptions) *SingleResult
func (coll *Collection) FindOneAndUpdate(ctx context.Context, filter interface{},
update interface{}, opts ...*options.FindOneAndUpdateOptions) *SingleResult
// 監(jiān)聽
func (coll *Collection) Watch(ctx context.Context, pipeline interface{},
opts ...*options.ChangeStreamOptions) (*ChangeStream, error)
// 索引視圖
func (coll *Collection) Indexes() IndexView
// 銷毀
func (coll *Collection) Drop(ctx context.Context) error
```
#### ___Cursor___
用于迭代文檔流。通過(guò)Decode或者通過(guò)Current字典訪問(wèn)原始的BSON都可以將一個(gè)數(shù)據(jù)庫(kù)文檔解碼為一個(gè)Go類型。不是線程安全的哦
```go
func (c *Cursor) Next(ctx context.Context) bool
func (c *Cursor) TryNext(ctx context.Context) bool
// 將bson反序列化到val中
func (c *Cursor) Decode(val interface{})
func (c *Cursor) Err() error
func (c *Cursor) Close(ctx context.Context) error
func (c *Cursor) All(ctx context.Context, results interface{}) error
```