Java :查找次數和連接(兩種方法),String方法圖,替換,相等,提取,輸入,翻轉
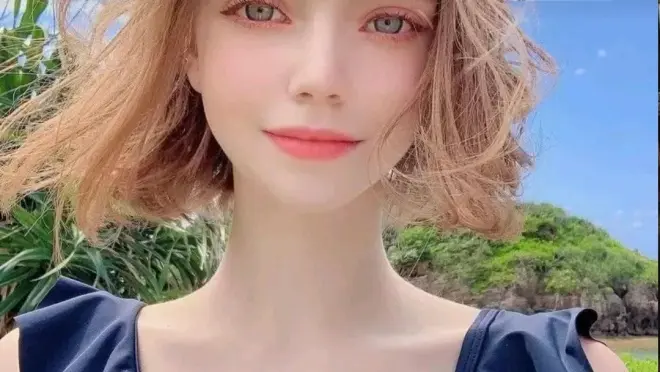
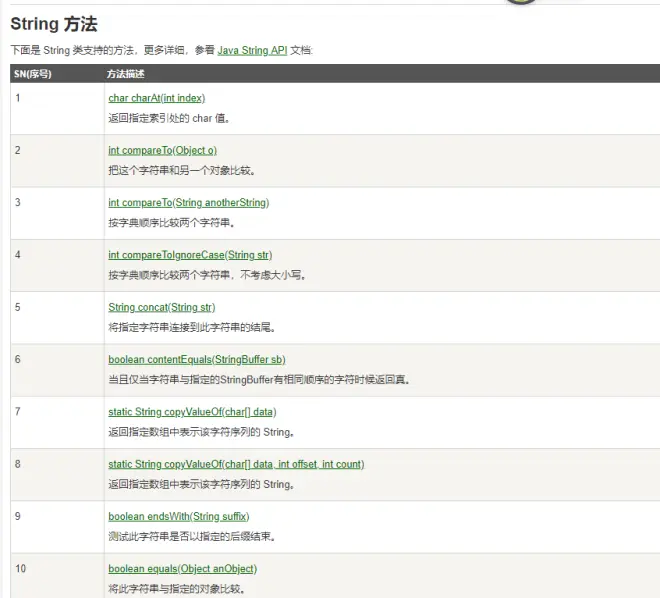
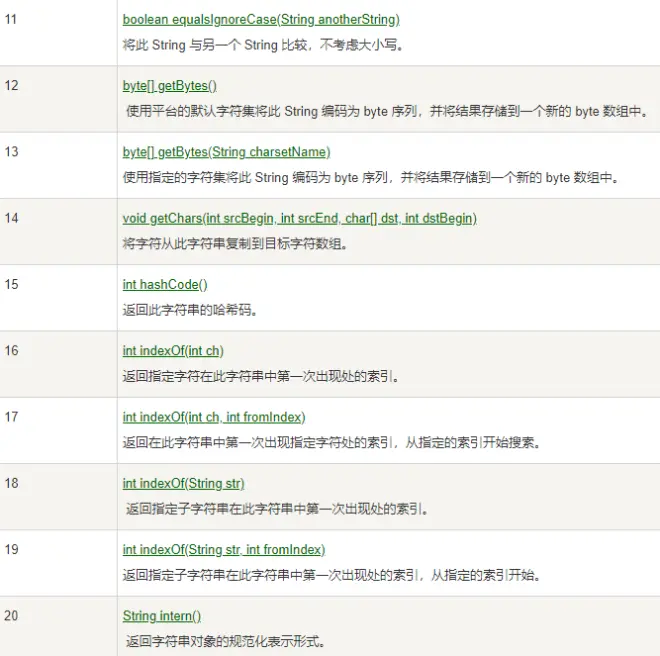
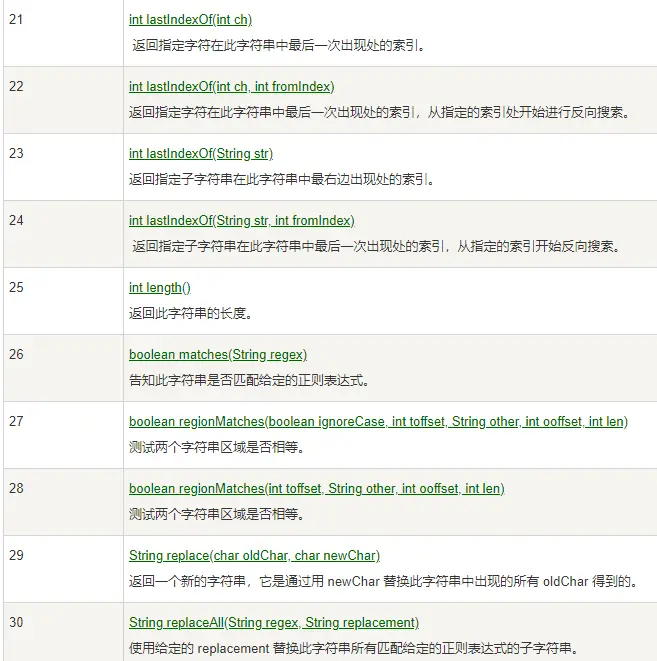
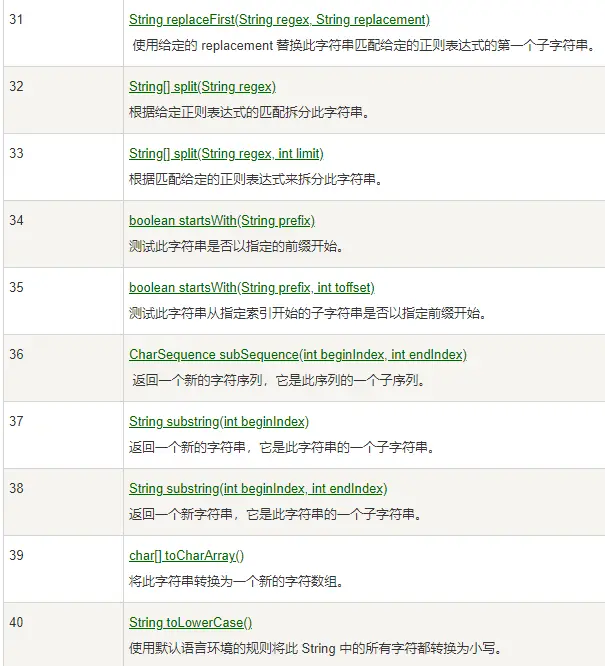
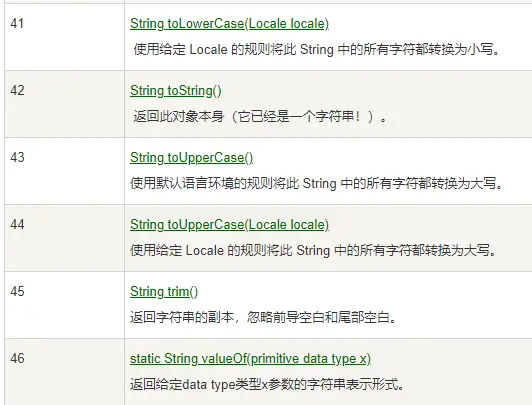
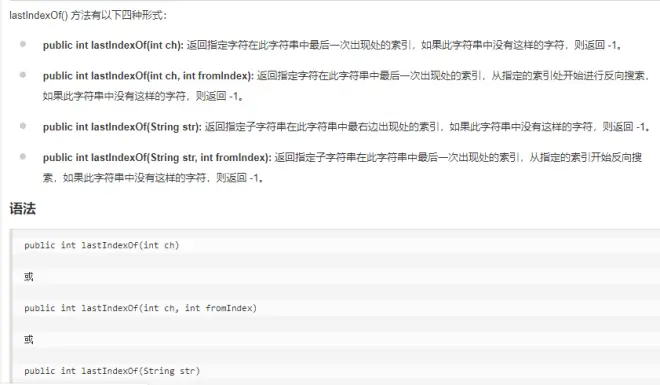
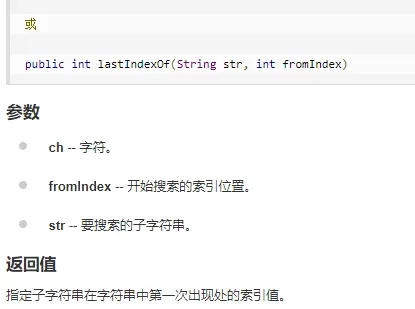
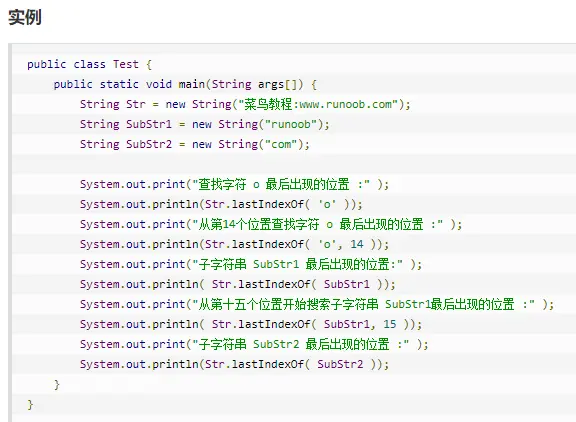
//練習內容一:編寫一個Java程序,完成以下功能:
//? ? 聲明一個名為s的String對象,并使它的內容是"Call me Accp";
//? ? 打印整個字符串。
//? ? 使用length()方法打印字符串的長度
//? ? 打印字符串的第一個字符
//? ? 打印字符串的最后一個字符
//? ? 打印字符串的第一個單詞。
//? ? ? ? ? 打印字符串的最后一個單詞。
package a;
public class fuxi {
public static void main(String[] args) {
String s = "Call me Accp";
int len = s.length();
char charAt = s.charAt(0);
char charAt2 = s.charAt(len - 1);
System.out.println("打印整個字符串: " + s);
System.out.println("Call me Accp的長度 : " + len);
System.out.println("Call me Accp的第一個字符 : " + charAt);
System.out.println("Call me Accp的最后一個字符 : " + charAt2);
String a = s.substring(s.lastIndexOf(" ") + 1);
String a1 = s.substring(s.lastIndexOf(" "));
String a2 = s.substring(s.lastIndexOf(" ") + 2);
String a3 = s.substring(s.lastIndexOf(" ") - 1);
String b = s.substring(0, 5);
System.out.println("字符串的第一個單詞:" + b);
System.out.println("字符串的最后一個單詞" + a);
}
}
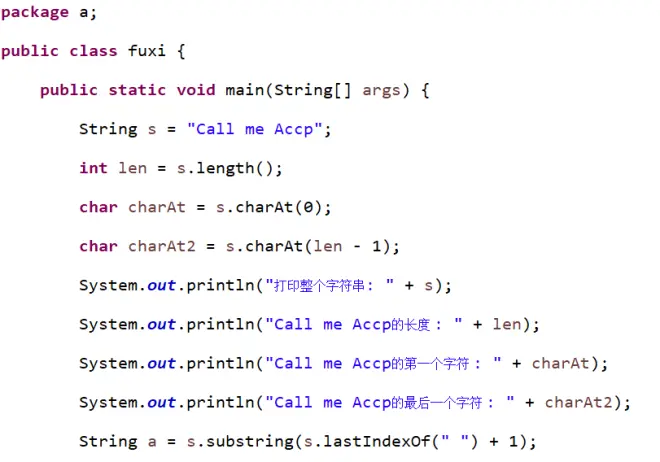
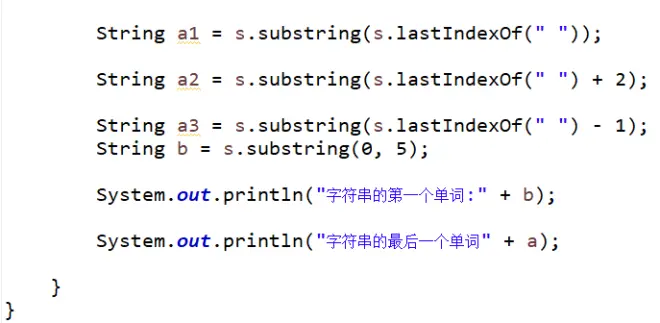
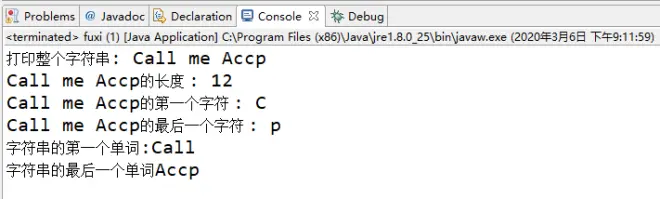
// 練習內容二:編寫一個Java程序,用于
// 測試兩個字符串String firstString="This is"和String secondString="This is";是否相等。
// 將"his father"與其中的firstString字符串連接。
// 用z替換新字符串中的i。
方法一:
package a;
public class fuxi {
public static void main(String[] args) {
String S1 = "This is";
String S2 = "This is";
if (S1.equals(S2)) {
System.out.println("字符串S1和S2相等");
}
else {
System.out.println("字符串S1和S2不相等");
}
String[] arr = { S1, "his father" };
String d = arr[0] + arr[1];/* 下標一定要對 */
System.out.println(d);
System.out.println("用z替換新字符串d中的i后的結果:" + d.replace('i', 'z'));
}
}
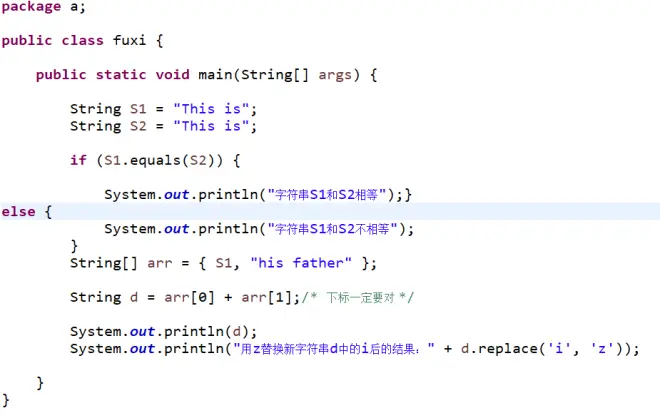
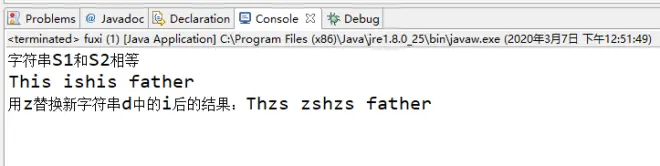
方法二:
package a;
public class fuxi {
public static void main(String[] args) {
String S1 = "This is";
String S2 = "This is";
if (S1.equals(S2)) {
System.out.println("字符串S1和S2相等");
}
else {
System.out.println("字符串S1和S2不相等");
}
String[] arr = { S1, "his father" };
String d = arr[0].concat(arr[1]);/* 下標一定要對 */
System.out.println("S1和結果his father連接的結果:" + d);
System.out.println("用z替換新字符串d中的i后的結果:" + d.replace('i', 'z'));
}
}
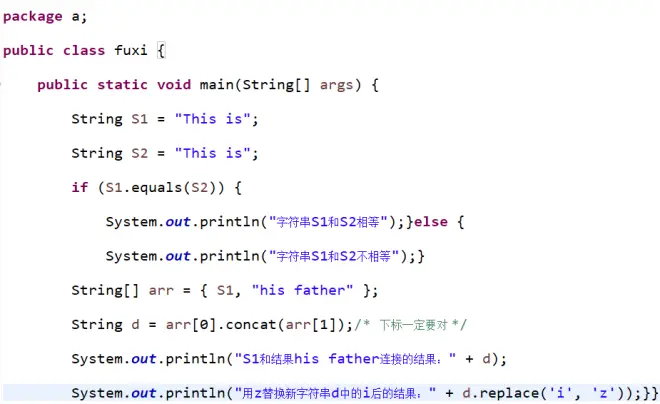

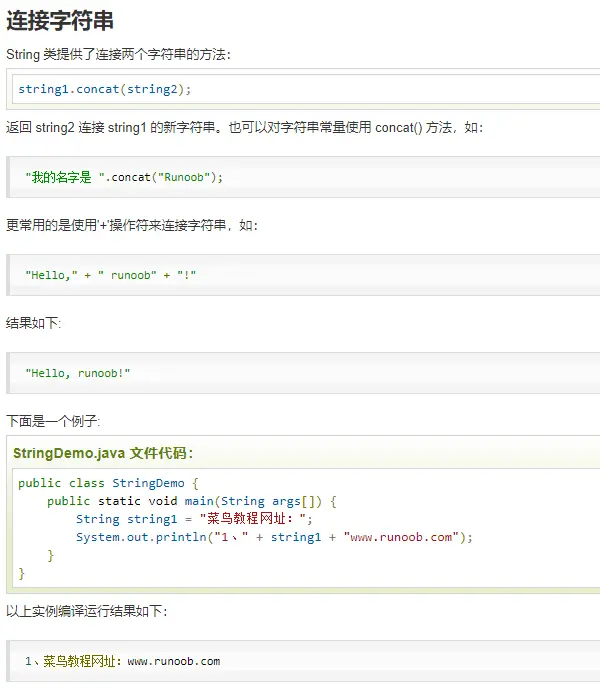
// 練習三:編寫一個java程序,從字符串"wellcom to Beijing"中提取單詞"well"和"Beijing".(使用subString()來做)
package a;
public class fuxi {
public static void main(String[] args) {
String a = "wellcom to Beijing";
String d = a.substring(0, 7);/* 下標一定要對 */
String c = a.substring(a.lastIndexOf(" ")
);/* 下標一定要對,a.lastIndexOf(" ")表明空格后面的字符,substring表明截取 */
System.out.println("提取單詞well:" + d);
System.out.println("提取單詞Beijing:" + c);
}
}
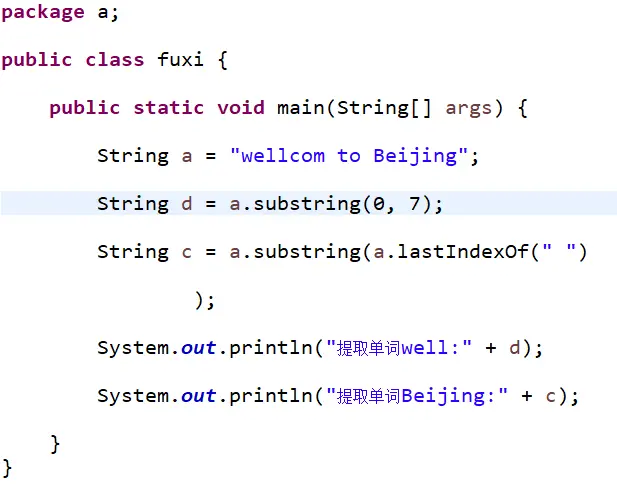

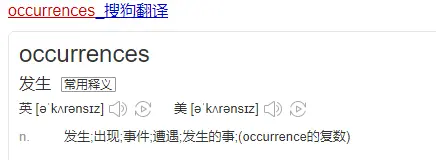
練習內容四:構建一個Occurrences程序,查找字符"e"在字符串"Seize the day"中出現的次數。
方法一:
package a;
public class fuxi {
public static void main(String[] args) {
String str = "Seize the day";
int count = 0;
// 統(tǒng)計出現了多少次的變量
int start = 0;// 每次查找開始的位置
while ((start = str.indexOf("e", start)) != -1) {
count++;// 如果循環(huán)一次出現 的次數就+1
start++;// 并且讓其開始的位置+1,如果不+1就會出現死循環(huán)
}
System.out.println("e在Seize the day中出現了" + count + "次");
}
}
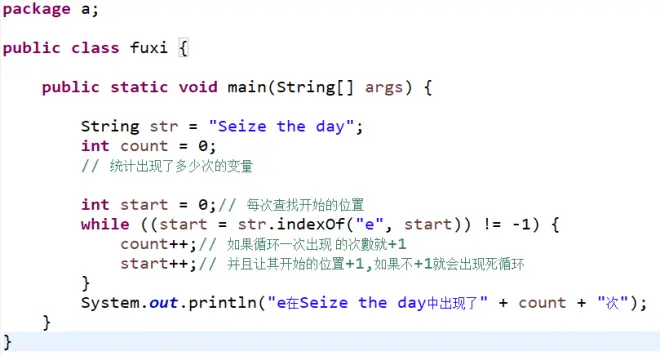

方法二:
package a;
public class fuxi {
public static void main(String[] args) {
String str = "Seize the day";
int times = searchstr("e", str);
System.out.println("字符“e”在字符串“Seize the day”中出現的次數:" + times);
}
public static int searchstr(String key, String str) {
int index = 0;
int count = 0;
while ((index = str.indexOf(key, index)) != -1) {
index = index + key.length();
count++;
}
return count;
}
}
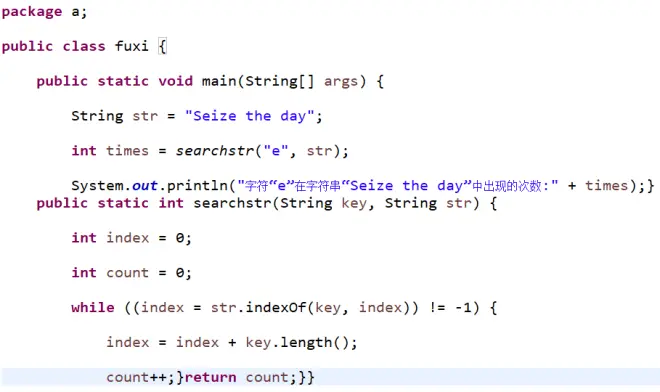

/*練習內容五:編寫一個ExtractString程序,從字符串"Seize the Day"中提取單詞"the"和"Day".*/
package a;
public class fuxi {
public static void main(String[] args) {
String a = "Seize the Day";
String d = a.substring(6, 9);
String c = a.substring(10);
System.out.println("提取單詞the:" + d);
System.out.println("提取單詞Day:" + c);
}
}
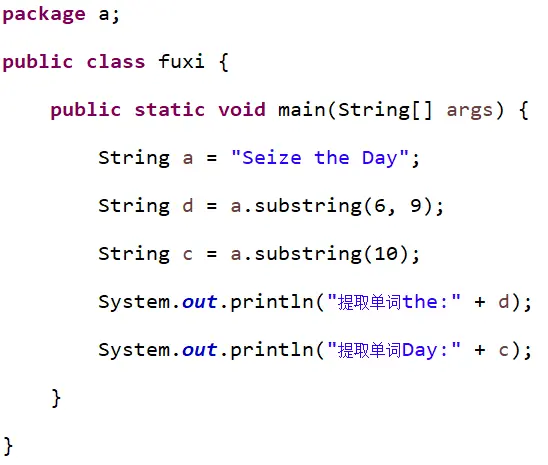

// 練習六:"今天的天氣不錯,雖然天氣不錯,但是我希望天氣能夠更好點",統(tǒng)計"天氣"出現的次數
package a;
public class fuxi {
public static void main(String[] args) {
String str = "今天的天氣不錯,雖然天氣不錯,但是我希望天氣能夠更好點";
int times = searchstr("天氣", str);
System.out.println("字符“天氣”在字符串“今天的天氣不錯,雖然天氣不錯,"
+ "但是我希望天氣能夠更好點”中出現的次數:" + times);
}
public static int searchstr(String key, String str) {
int index = 0;
int count = 0;
while ((index = str.indexOf(key, index)) != -1) {
index = index + key.length();
count++;
}
return count;
}
}
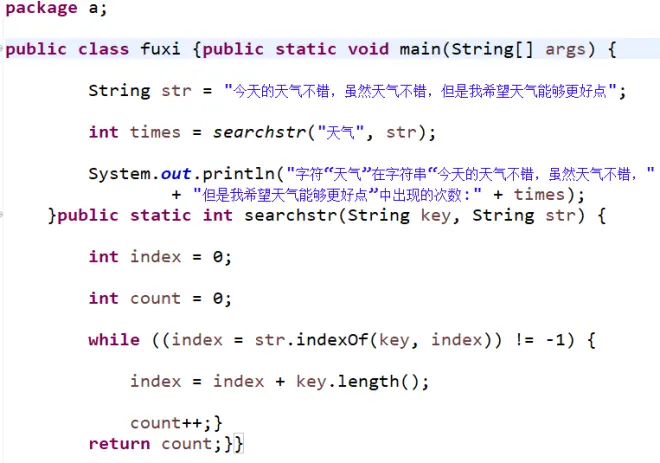

//? 練習七:要求用戶輸入一個字符串,對其進行翻轉
package a;
import java.util.Scanner;
public class fuxi {
public static void main(String[] args) {
System.out.println("請輸入一個字符串");
Scanner shurudeneirong = new Scanner(System.in);
String jieshoudeneirong = shurudeneirong.next();
String reverse = new StringBuffer(jieshoudeneirong).reverse()
.toString();
System.out.println("字符串反轉前:" + jieshoudeneirong);
System.out.println("字符串反轉后:" + reverse);}}
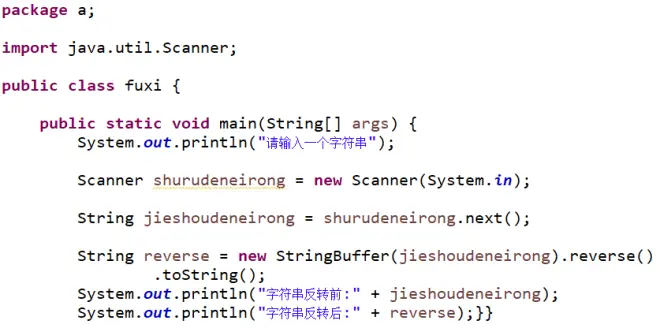
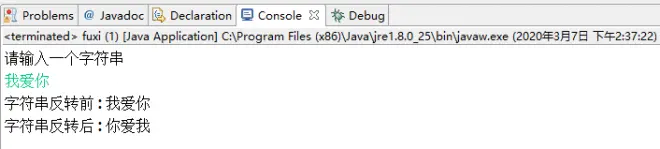