修改密碼(react+ts+antd)
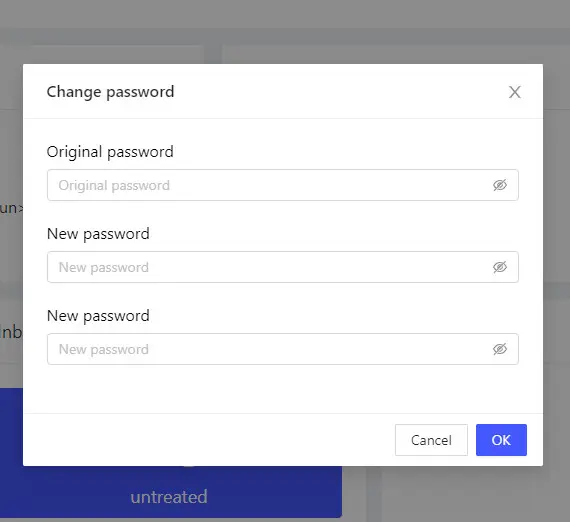



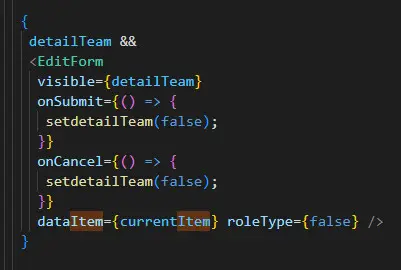
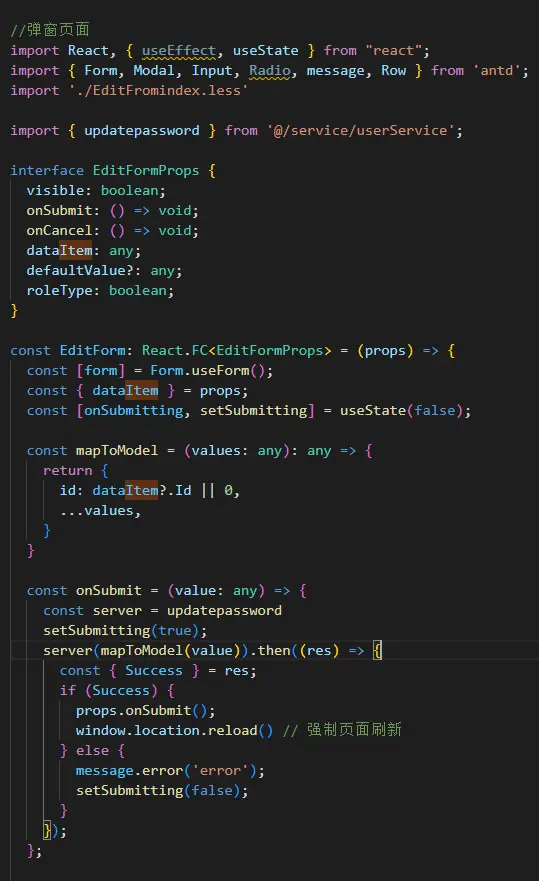
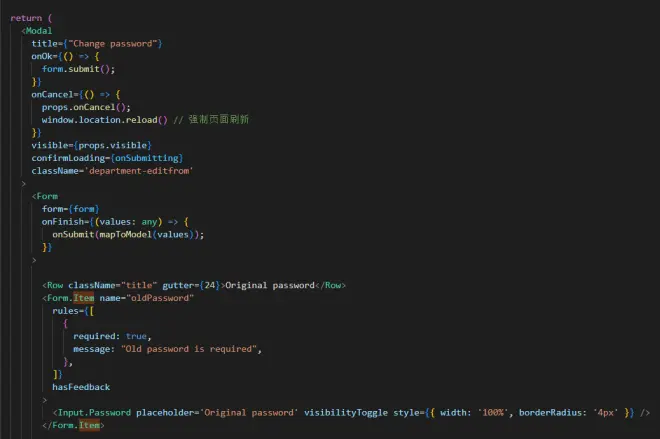
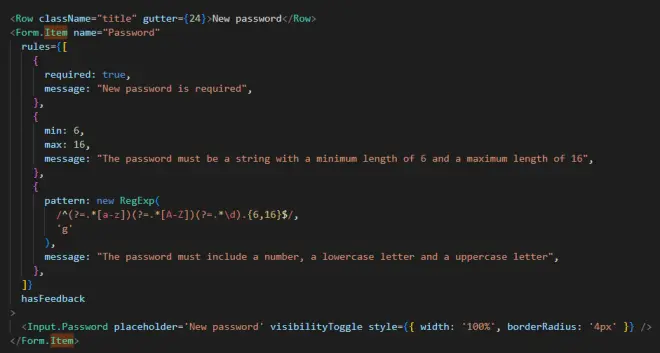
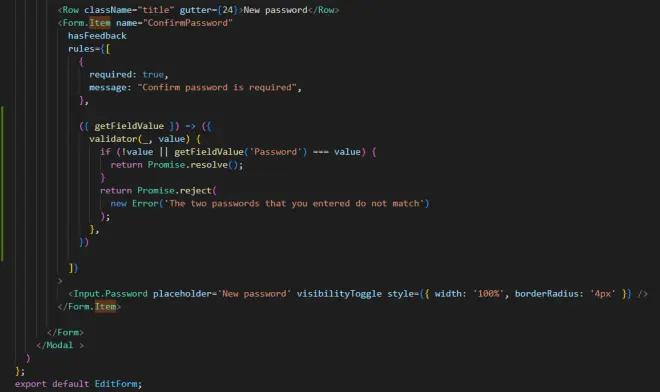
彈窗頁(yè)面代碼
//彈窗頁(yè)面
import React, { useEffect, useState } from "react";
import { Form, Modal, Input, Radio, message, Row } from 'antd';
import './EditFromindex.less'
import { updatepassword } from '@/service/userService';
interface EditFormProps {
? visible: boolean;
? onSubmit: () => void;
? onCancel: () => void;
? dataItem: any;
? defaultValue?: any;
? roleType: boolean;
}
const EditForm: React.FC<EditFormProps> = (props) => {
? const [form] = Form.useForm();
? const { dataItem } = props;
? const [onSubmitting, setSubmitting] = useState(false);
? const mapToModel = (values: any): any => {
? ? return {
? ? ? id: dataItem?.Id || 0,
? ? ? ...values,
? ? }
? }
? const onSubmit = (value: any) => {
? ? const server = updatepassword
? ? setSubmitting(true);
? ? server(mapToModel(value)).then((res) => {
? ? ? const { Success } = res;
? ? ? if (Success) {
? ? ? ? props.onSubmit();
? ? ? ? window.location.reload() // 強(qiáng)制頁(yè)面刷新
? ? ? } else {
? ? ? ? message.error('error');
? ? ? ? setSubmitting(false);
? ? ? }
? ? });
? };
? return (
? ? <Modal
? ? ? title={"Change password"}
? ? ? onOk={() => {
? ? ? ? form.submit();
? ? ? }}
? ? ? onCancel={() => {
? ? ? ? props.onCancel();
? ? ? ? window.location.reload() // 強(qiáng)制頁(yè)面刷新
? ? ? }}
? ? ? visible={props.visible}
? ? ? confirmLoading={onSubmitting}
? ? ? className='department-editfrom'
? ? >
? ? ? <Form
? ? ? ? form={form}
? ? ? ? onFinish={(values: any) => {
? ? ? ? ? onSubmit(mapToModel(values));
? ? ? ? }}
? ? ? >
? ? ? ? <Row className="title" gutter={24}>Original password</Row>
? ? ? ? <Form.Item name="oldPassword"
? ? ? ? ? rules={[
? ? ? ? ? ? {
? ? ? ? ? ? ? required: true,
? ? ? ? ? ? ? message: "Old password is required",
? ? ? ? ? ? },
? ? ? ? ? ]}
? ? ? ? ? hasFeedback
? ? ? ? >
? ? ? ? ? <Input.Password placeholder='Original password' visibilityToggle style={{ width: '100%', borderRadius: '4px' }} />
? ? ? ? </Form.Item>
? ? ? ? <Row className="title" gutter={24}>New password</Row>
? ? ? ? <Form.Item name="Password"
? ? ? ? ? rules={[
? ? ? ? ? ? {
? ? ? ? ? ? ? required: true,
? ? ? ? ? ? ? message: "New password is required",
? ? ? ? ? ? },
? ? ? ? ? ? {
? ? ? ? ? ? ? min: 6,
? ? ? ? ? ? ? max: 16,
? ? ? ? ? ? ? message: "The password must be a string with a minimum length of 6 and a maximum length of 16",
? ? ? ? ? ? },
? ? ? ? ? ? {
? ? ? ? ? ? ? pattern: new RegExp(
? ? ? ? ? ? ? ? /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d).{6,16}$/,
? ? ? ? ? ? ? ? 'g'
? ? ? ? ? ? ? ),
? ? ? ? ? ? ? message: "The password must include a number, a lowercase letter and a uppercase letter",
? ? ? ? ? ? },
? ? ? ? ? ]}
? ? ? ? ? hasFeedback
? ? ? ? >
? ? ? ? ? <Input.Password placeholder='New password' visibilityToggle style={{ width: '100%', borderRadius: '4px' }} />
? ? ? ? </Form.Item>
? ? ? ? <Row className="title" gutter={24}>New password</Row>
? ? ? ? <Form.Item name="ConfirmPassword"
? ? ? ? ? hasFeedback
? ? ? ? ? rules={[
? ? ? ? ? ? {
? ? ? ? ? ? ? required: true,
? ? ? ? ? ? ? message: "Confirm password is required",
? ? ? ? ? ? },
? ? ? ? ? ? ({ getFieldValue }) => ({
? ? ? ? ? ? ? validator(_, value) {
? ? ? ? ? ? ? ? if (!value || getFieldValue('Password') === value) {
? ? ? ? ? ? ? ? ? return Promise.resolve();
? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? return Promise.reject(
? ? ? ? ? ? ? ? ? new Error('The two passwords that you entered do not match')
? ? ? ? ? ? ? ? );
? ? ? ? ? ? ? },
? ? ? ? ? ? })
? ? ? ? ? ]}
? ? ? ? >
? ? ? ? ? <Input.Password placeholder='New password' visibilityToggle style={{ width: '100%', borderRadius: '4px' }} />
? ? ? ? </Form.Item>
? ? ? </Form>
? ? </Modal >
? )
};
export default EditForm;
主頁(yè)面代碼:
import React, { useEffect, useState } from 'react';
import { StateType } from '@/models/login';
import { Menu, Dropdown } from 'antd';
import { history, useIntl, connect, ConnectProps, Dispatch, Link, FormattedMessage } from 'umi';
import { User } from '@/typings';
import { getCurrentUser } from '@/utils/authority';
import EditForm from './components/EditForm'
import { SettingOutlined } from '@ant-design/icons';
// import Profile from '../../pages/Profiles/index'
interface ProfileProps extends ConnectProps {
?login: StateType;
?ResData: User;
?dispatch: Dispatch;
?detailTeam: boolean;
}
const Profileheader: React.FC<ProfileProps> = (props: any) => {
?useEffect(() => {
? dispatch({
? ?type: 'newProfileModle/getByUserId'
? })
?}, [])
?const [profileVisible, setprofileVisible] = useState(false)
?const [detailTeam, setdetailTeam] = useState(false)
?const { currentUser, dispatch, currentItem } = props;
?const menu = (
? <Menu>
? ?<Menu.Item onClick={() => {
? ? history.push('/settings/profile')
? ? // setprofileVisible(true)
? ?}}><FormattedMessage
? ? ?id={'page.profile.profile'}
? ? ?defaultMessage="Profile"
? ? /></Menu.Item>
? ?<Menu.Item>
? ? <span onClick={() => { setdetailTeam(true); }}>Change password</span>
? ?</Menu.Item>
? ?<Menu.Item>
? ? <Link to="/Settings/Agent">Agent</Link>
? ?</Menu.Item>
? ?<Menu.Item>
? ? <Link to="/logout"><FormattedMessage
? ? ?id={'page.profile.logout'}
? ? ?defaultMessage="Logout"
? ? /></Link>
? ?</Menu.Item>
? </Menu>
?);
?return (
? <div>
? ?<Dropdown overlay={menu}>
? ? <div className="currentUser_name">{currentUser?.Member?.Name || currentUser?.Name} <em></em></div>
? ?</Dropdown>
? ?{/* {
? ? profileVisible ?
? ? <Profile defaultValue={currentItem} /> :
? ? null
? ?} */}
? ?{
? ? detailTeam &&
? ? <EditForm
? ? ?visible={detailTeam}
? ? ?onSubmit={() => {
? ? ? setdetailTeam(false);
? ? ?}}
? ? ?onCancel={() => {
? ? ? setdetailTeam(false);
? ? ?}}
? ? ?dataItem={currentItem} roleType={false} />
? ?}
? </div >
?);
};
export default connect(({ login, newProfileModle }: { login: StateType, newProfileModle: any }) => ({
?...login,
?...newProfileModle,
?currentUser: getCurrentUser(),
}))(Profileheader);