2023-05-26:golang關(guān)于垃圾回收和析構(gòu)函數(shù)的選擇題,多數(shù)人會選錯。
2023-05-26:golang關(guān)于垃圾回收和析構(gòu)的選擇題,代碼如下:
package?main
import?(
????"fmt"
????"runtime"
????"time"
)
type?ListNode?struct?{
????Val??int
????Next?*ListNode
}
func?main0()?{
????a?:=?&ListNode{Val:?1}
????b?:=?&ListNode{Val:?2}
????runtime.SetFinalizer(a,?func(obj?*ListNode)?{
????????fmt.Printf("a被回收--")
????})
????runtime.SetFinalizer(b,?func(obj?*ListNode)?{
????????fmt.Printf("b被回收--")
????})
????a.Next?=?b
????b.Next?=?a
}
func?main()?{
????main0()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????fmt.Print("結(jié)束")
}
代碼的運行結(jié)果是什么?并說明原因。注意析構(gòu)是無序的。
A. 結(jié)束
B. a被回收--b被回收--結(jié)束
C. b被回收--a被回收--結(jié)束
D. B和C都有可能
答案2023-05-26:
golang的垃圾回收算法跟java一樣,都是根可達算法。代碼中main0函數(shù)里a和b是互相引用,但是a和b沒有外部引用。因此a和b會被當成垃圾被回收掉。而析構(gòu)函數(shù)的調(diào)用不是有序的,所以B和C都有可能,答案選D。讓我們看看答案是什么,如下:
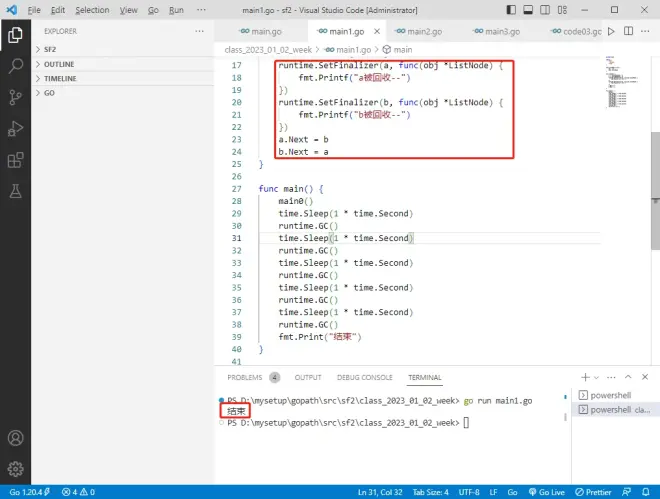
看運行結(jié)果,答案不是選D,而是選A。這肯定會出乎很多人意料,golang的垃圾回收算法是根可達算法難不成是假的,大家公認的八股文難道是錯的?有這個疑問是好事,但不能全盤否定。讓我們看看析構(gòu)函數(shù)的源碼吧。代碼在?src/runtime/mfinal.go
?中,如下:
//?SetFinalizer?sets?the?finalizer?associated?with?obj?to?the?provided
//?finalizer?function.?When?the?garbage?collector?finds?an?unreachable?block
//?with?an?associated?finalizer,?it?clears?the?association?and?runs
//?finalizer(obj)?in?a?separate?goroutine.?This?makes?obj?reachable?again,
//?but?now?without?an?associated?finalizer.?Assuming?that?SetFinalizer
//?is?not?called?again,?the?next?time?the?garbage?collector?sees
//?that?obj?is?unreachable,?it?will?free?obj.
//
//?SetFinalizer(obj,?nil)?clears?any?finalizer?associated?with?obj.
//
//?The?argument?obj?must?be?a?pointer?to?an?object?allocated?by?calling
//?new,?by?taking?the?address?of?a?composite?literal,?or?by?taking?the
//?address?of?a?local?variable.
//?The?argument?finalizer?must?be?a?function?that?takes?a?single?argument
//?to?which?obj's?type?can?be?assigned,?and?can?have?arbitrary?ignored?return
//?values.?If?either?of?these?is?not?true,?SetFinalizer?may?abort?the
//?program.
//
//?Finalizers?are?run?in?dependency?order:?if?A?points?at?B,?both?have
//?finalizers,?and?they?are?otherwise?unreachable,?only?the?finalizer
//?for?A?runs;?once?A?is?freed,?the?finalizer?for?B?can?run.
//?If?a?cyclic?structure?includes?a?block?with?a?finalizer,?that
//?cycle?is?not?guaranteed?to?be?garbage?collected?and?the?finalizer
//?is?not?guaranteed?to?run,?because?there?is?no?ordering?that
//?respects?the?dependencies.
//
//?The?finalizer?is?scheduled?to?run?at?some?arbitrary?time?after?the
//?program?can?no?longer?reach?the?object?to?which?obj?points.
//?There?is?no?guarantee?that?finalizers?will?run?before?a?program?exits,
//?so?typically?they?are?useful?only?for?releasing?non-memory?resources
//?associated?with?an?object?during?a?long-running?program.
//?For?example,?an?os.File?object?could?use?a?finalizer?to?close?the
//?associated?operating?system?file?descriptor?when?a?program?discards
//?an?os.File?without?calling?Close,?but?it?would?be?a?mistake
//?to?depend?on?a?finalizer?to?flush?an?in-memory?I/O?buffer?such?as?a
//?bufio.Writer,?because?the?buffer?would?not?be?flushed?at?program?exit.
//
//?It?is?not?guaranteed?that?a?finalizer?will?run?if?the?size?of?*obj?is
//?zero?bytes,?because?it?may?share?same?address?with?other?zero-size
//?objects?in?memory.?See?https://go.dev/ref/spec#Size_and_alignment_guarantees.
//
//?It?is?not?guaranteed?that?a?finalizer?will?run?for?objects?allocated
//?in?initializers?for?package-level?variables.?Such?objects?may?be
//?linker-allocated,?not?heap-allocated.
//
//?Note?that?because?finalizers?may?execute?arbitrarily?far?into?the?future
//?after?an?object?is?no?longer?referenced,?the?runtime?is?allowed?to?perform
//?a?space-saving?optimization?that?batches?objects?together?in?a?single
//?allocation?slot.?The?finalizer?for?an?unreferenced?object?in?such?an
//?allocation?may?never?run?if?it?always?exists?in?the?same?batch?as?a
//?referenced?object.?Typically,?this?batching?only?happens?for?tiny
//?(on?the?order?of?16?bytes?or?less)?and?pointer-free?objects.
//
//?A?finalizer?may?run?as?soon?as?an?object?becomes?unreachable.
//?In?order?to?use?finalizers?correctly,?the?program?must?ensure?that
//?the?object?is?reachable?until?it?is?no?longer?required.
//?Objects?stored?in?global?variables,?or?that?can?be?found?by?tracing
//?pointers?from?a?global?variable,?are?reachable.?For?other?objects,
//?pass?the?object?to?a?call?of?the?KeepAlive?function?to?mark?the
//?last?point?in?the?function?where?the?object?must?be?reachable.
//
//?For?example,?if?p?points?to?a?struct,?such?as?os.File,?that?contains
//?a?file?descriptor?d,?and?p?has?a?finalizer?that?closes?that?file
//?descriptor,?and?if?the?last?use?of?p?in?a?function?is?a?call?to
//?syscall.Write(p.d,?buf,?size),?then?p?may?be?unreachable?as?soon?as
//?the?program?enters?syscall.Write.?The?finalizer?may?run?at?that?moment,
//?closing?p.d,?causing?syscall.Write?to?fail?because?it?is?writing?to
//?a?closed?file?descriptor?(or,?worse,?to?an?entirely?different
//?file?descriptor?opened?by?a?different?goroutine).?To?avoid?this?problem,
//?call?KeepAlive(p)?after?the?call?to?syscall.Write.
//
//?A?single?goroutine?runs?all?finalizers?for?a?program,?sequentially.
//?If?a?finalizer?must?run?for?a?long?time,?it?should?do?so?by?starting
//?a?new?goroutine.
//
//?In?the?terminology?of?the?Go?memory?model,?a?call
//?SetFinalizer(x,?f)?“synchronizes?before”?the?finalization?call?f(x).
//?However,?there?is?no?guarantee?that?KeepAlive(x)?or?any?other?use?of?x
//?“synchronizes?before”?f(x),?so?in?general?a?finalizer?should?use?a?mutex
//?or?other?synchronization?mechanism?if?it?needs?to?access?mutable?state?in?x.
//?For?example,?consider?a?finalizer?that?inspects?a?mutable?field?in?x
//?that?is?modified?from?time?to?time?in?the?main?program?before?x
//?becomes?unreachable?and?the?finalizer?is?invoked.
//?The?modifications?in?the?main?program?and?the?inspection?in?the?finalizer
//?need?to?use?appropriate?synchronization,?such?as?mutexes?or?atomic?updates,
//?to?avoid?read-write?races.
func?SetFinalizer(obj?any,?finalizer?any)?{
????if?debug.sbrk?!=?0?{
????????//?debug.sbrk?never?frees?memory,?so?no?finalizers?run
????????//?(and?we?don't?have?the?data?structures?to?record?them).
????????return
????}
????e?:=?efaceOf(&obj)
????etyp?:=?e._type
????if?etyp?==?nil?{
????????throw("runtime.SetFinalizer:?first?argument?is?nil")
????}
????if?etyp.kind&kindMask?!=?kindPtr?{
????????throw("runtime.SetFinalizer:?first?argument?is?"?+?etyp.string()?+?",?not?pointer")
????}
????ot?:=?(*ptrtype)(unsafe.Pointer(etyp))
????if?ot.elem?==?nil?{
????????throw("nil?elem?type!")
????}
????if?inUserArenaChunk(uintptr(e.data))?{
????????//?Arena-allocated?objects?are?not?eligible?for?finalizers.
????????throw("runtime.SetFinalizer:?first?argument?was?allocated?into?an?arena")
????}
????//?find?the?containing?object
????base,?_,?_?:=?findObject(uintptr(e.data),?0,?0)
????if?base?==?0?{
????????//?0-length?objects?are?okay.
????????if?e.data?==?unsafe.Pointer(&zerobase)?{
????????????return
????????}
????????//?Global?initializers?might?be?linker-allocated.
????????//?var?Foo?=?&Object{}
????????//?func?main()?{
????????//??runtime.SetFinalizer(Foo,?nil)
????????//?}
????????//?The?relevant?segments?are:?noptrdata,?data,?bss,?noptrbss.
????????//?We?cannot?assume?they?are?in?any?order?or?even?contiguous,
????????//?due?to?external?linking.
????????for?datap?:=?&firstmoduledata;?datap?!=?nil;?datap?=?datap.next?{
????????????if?datap.noptrdata?<=?uintptr(e.data)?&&?uintptr(e.data)?<?datap.enoptrdata?||
????????????????datap.data?<=?uintptr(e.data)?&&?uintptr(e.data)?<?datap.edata?||
????????????????datap.bss?<=?uintptr(e.data)?&&?uintptr(e.data)?<?datap.ebss?||
????????????????datap.noptrbss?<=?uintptr(e.data)?&&?uintptr(e.data)?<?datap.enoptrbss?{
????????????????return
????????????}
????????}
????????throw("runtime.SetFinalizer:?pointer?not?in?allocated?block")
????}
????if?uintptr(e.data)?!=?base?{
????????//?As?an?implementation?detail?we?allow?to?set?finalizers?for?an?inner?byte
????????//?of?an?object?if?it?could?come?from?tiny?alloc?(see?mallocgc?for?details).
????????if?ot.elem?==?nil?||?ot.elem.ptrdata?!=?0?||?ot.elem.size?>=?maxTinySize?{
????????????throw("runtime.SetFinalizer:?pointer?not?at?beginning?of?allocated?block")
????????}
????}
????f?:=?efaceOf(&finalizer)
????ftyp?:=?f._type
????if?ftyp?==?nil?{
????????//?switch?to?system?stack?and?remove?finalizer
????????systemstack(func()?{
????????????removefinalizer(e.data)
????????})
????????return
????}
????if?ftyp.kind&kindMask?!=?kindFunc?{
????????throw("runtime.SetFinalizer:?second?argument?is?"?+?ftyp.string()?+?",?not?a?function")
????}
????ft?:=?(*functype)(unsafe.Pointer(ftyp))
????if?ft.dotdotdot()?{
????????throw("runtime.SetFinalizer:?cannot?pass?"?+?etyp.string()?+?"?to?finalizer?"?+?ftyp.string()?+?"?because?dotdotdot")
????}
????if?ft.inCount?!=?1?{
????????throw("runtime.SetFinalizer:?cannot?pass?"?+?etyp.string()?+?"?to?finalizer?"?+?ftyp.string())
????}
????fint?:=?ft.in()[0]
????switch?{
????case?fint?==?etyp:
????????//?ok?-?same?type
????????goto?okarg
????case?fint.kind&kindMask?==?kindPtr:
????????if?(fint.uncommon()?==?nil?||?etyp.uncommon()?==?nil)?&&?(*ptrtype)(unsafe.Pointer(fint)).elem?==?ot.elem?{
????????????//?ok?-?not?same?type,?but?both?pointers,
????????????//?one?or?the?other?is?unnamed,?and?same?element?type,?so?assignable.
????????????goto?okarg
????????}
????case?fint.kind&kindMask?==?kindInterface:
????????ityp?:=?(*interfacetype)(unsafe.Pointer(fint))
????????if?len(ityp.mhdr)?==?0?{
????????????//?ok?-?satisfies?empty?interface
????????????goto?okarg
????????}
????????if?iface?:=?assertE2I2(ityp,?*efaceOf(&obj));?iface.tab?!=?nil?{
????????????goto?okarg
????????}
????}
????throw("runtime.SetFinalizer:?cannot?pass?"?+?etyp.string()?+?"?to?finalizer?"?+?ftyp.string())
okarg:
????//?compute?size?needed?for?return?parameters
????nret?:=?uintptr(0)
????for?_,?t?:=?range?ft.out()?{
????????nret?=?alignUp(nret,?uintptr(t.align))?+?uintptr(t.size)
????}
????nret?=?alignUp(nret,?goarch.PtrSize)
????//?make?sure?we?have?a?finalizer?goroutine
????createfing()
????systemstack(func()?{
????????if?!addfinalizer(e.data,?(*funcval)(f.data),?nret,?fint,?ot)?{
????????????throw("runtime.SetFinalizer:?finalizer?already?set")
????????}
????})
}
看代碼,看不出什么。其端倪在注釋中。注意如下注釋:
// Finalizers are run in dependency order: if A points at B, both have
// finalizers, and they are otherwise unreachable, only the finalizer
// for A runs; once A is freed, the finalizer for B can run.
// If a cyclic structure includes a block with a finalizer, that
// cycle is not guaranteed to be garbage collected and the finalizer
// is not guaranteed to run, because there is no ordering that
// respects the dependencies.
這段英文翻譯成中文如下:
Finalizers(終結(jié)器)按照依賴順序運行:如果 A 指向 B,兩者都有終結(jié)器,并且它們除此之外不可達,則僅運行 A 的終結(jié)器;一旦 A 被釋放,可以運行 B 的終結(jié)器。如果一個循環(huán)結(jié)構(gòu)包含一個具有終結(jié)器的塊,則該循環(huán)體不能保證被垃圾回收并且終結(jié)器不能保證運行,因為沒有符合依賴關(guān)系的排序方式。
這意思很明顯了,析構(gòu)函數(shù)會檢查當前對象A是否有外部對象指向當前對象A。如果有外部對象指向當前對象A時,A的析構(gòu)是無法執(zhí)行的;如果有外部對象指向當前對象A時,A的析構(gòu)才能執(zhí)行。
代碼中的a和b是循環(huán)依賴,當析構(gòu)判斷a和b時,都會有外部對象指向a和b,析構(gòu)函數(shù)無法執(zhí)行。析構(gòu)無法執(zhí)行,內(nèi)存也無法回收。因此答案選A。
去掉析構(gòu)函數(shù)后,a和b肯定會被釋放的。不用析構(gòu)函數(shù)去證明,那如何證明呢?用以下代碼就可以證明,代碼如下:
package?main
import?(
????"fmt"
????"runtime"
????"time"
)
type?ListNode?struct?{
????Val??[1024?*?1024]bool
????Next?*ListNode
}
func?printAlloc()?{
????var?m?runtime.MemStats
????runtime.ReadMemStats(&m)
????fmt.Printf("%d?KB\n",?m.Alloc/1024)
}
func?main0()?{
????printAlloc()
????a?:=?&ListNode{Val:?[1024?*?1024]bool{true}}
????b?:=?&ListNode{Val:?[1024?*?1024]bool{false}}
????a.Next?=?b
????b.Next?=?a
????//?runtime.SetFinalizer(a,?func(obj?*ListNode)?{
????//??fmt.Printf("a被刪除--")
????//?})
????printAlloc()
}
func?main()?{
????fmt.Print("開始")
????main0()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????fmt.Print("結(jié)束")
????printAlloc()
}
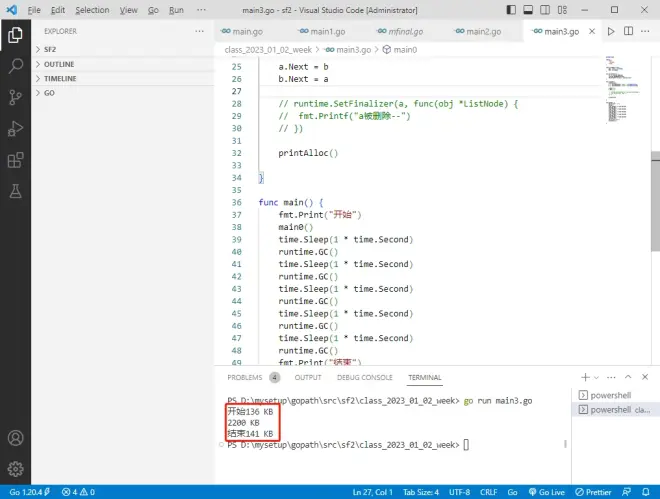
根據(jù)運行結(jié)果,內(nèi)存大小明顯變小,說明a和b已經(jīng)被回收了。
讓我們再看看有析構(gòu)函數(shù)的情況,運行結(jié)果是咋樣的,如下:
package?main
import?(
????"fmt"
????"runtime"
????"time"
)
type?ListNode?struct?{
????Val??[1024?*?1024]bool
????Next?*ListNode
}
func?printAlloc()?{
????var?m?runtime.MemStats
????runtime.ReadMemStats(&m)
????fmt.Printf("%d?KB\n",?m.Alloc/1024)
}
func?main0()?{
????printAlloc()
????a?:=?&ListNode{Val:?[1024?*?1024]bool{true}}
????b?:=?&ListNode{Val:?[1024?*?1024]bool{false}}
????a.Next?=?b
????b.Next?=?a
????runtime.SetFinalizer(a,?func(obj?*ListNode)?{
????????fmt.Printf("a被刪除--")
????})
????printAlloc()
}
func?main()?{
????fmt.Print("開始")
????main0()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????time.Sleep(1?*?time.Second)
????runtime.GC()
????fmt.Print("結(jié)束")
????printAlloc()
}
根據(jù)運行結(jié)果,有析構(gòu)函數(shù)的情況下,a和b確實是無法被回收。
總結(jié)
1.不要懷疑八股文的正確性,golang的垃圾回收確實是根可達算法。
2.不要用析構(gòu)函數(shù)去測試無用對象被回收的情況,上面的例子也看到了,兩對象的循環(huán)引用,析構(gòu)函數(shù)的測試結(jié)果就是錯誤的。只能根據(jù)內(nèi)存變化,看無用對象是否被回收。
3.在寫代碼的時候,能手動設(shè)置引用為nil,最好手動設(shè)置,這樣能更好的避免內(nèi)存泄漏。