Android+servlet+navicat:登錄、注冊頁面 02(Java+navicat部分)
來源:我的學(xué)習(xí)筆記(這是Java和數(shù)據(jù)庫模塊)

項(xiàng)目結(jié)構(gòu):
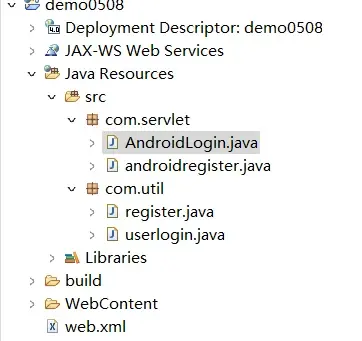

第一個(gè)servlet:
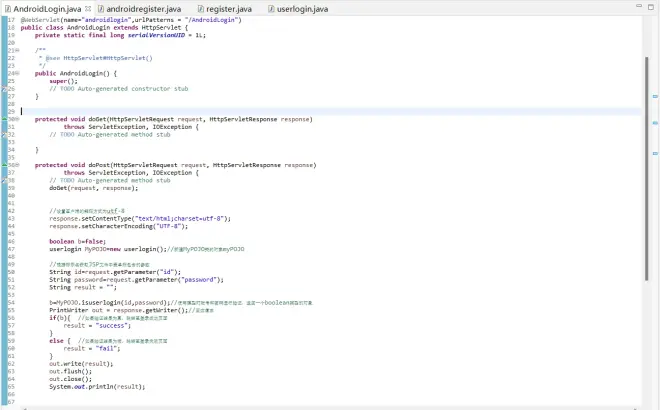
代碼:
package com.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.util.userlogin;
/**
?* Servlet implementation class AndroidLogin
?*/
@WebServlet(name="androidlogin",urlPatterns = "/AndroidLogin")
public class AndroidLogin extends HttpServlet {
private static final long serialVersionUID = 1L;
? ? ? ?
? ? /**
? ? ?* @see HttpServlet#HttpServlet()
? ? ?*/
? ? public AndroidLogin() {
? ? ? ? super();
? ? ? ? // TODO Auto-generated constructor stub
? ? }
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)?
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
?
? ? ? ? //設(shè)置客戶端的解碼方式為utf-8
? ? ? ? response.setContentType("text/html;charset=utf-8");
? ? ? ? response.setCharacterEncoding("UTF-8");
?
? ? ? ? boolean b=false;
? ? ? ? userlogin MyPOJO=new userlogin();//新建MyPOJO類的對象myPOJO
?
? ? ? ? //根據(jù)標(biāo)示名獲取JSP文件中表單所包含的參數(shù)
? ? ? ? String id=request.getParameter("id");
? ? ? ? String password=request.getParameter("password");
? ? ? ? String result = "";
?
? ? ? ? b=MyPOJO.isuserlogin(id,password);//使用模型對賬號和密碼進(jìn)行驗(yàn)證,返回一個(gè)boolean類型的對象
? ? ? ? PrintWriter out = response.getWriter();//回應(yīng)請求
? ? ? ? if(b){? //如果驗(yàn)證結(jié)果為真,跳轉(zhuǎn)至登錄成功頁面
? ? ? ? ? ? result = "success";
? ? ? ? }
? ? ? ? else {? //如果驗(yàn)證結(jié)果為假,跳轉(zhuǎn)至登錄失敗頁面
? ? ? ? ? ? result = "fail";
? ? ? ? }
? ? ? ? out.write(result);
? ? ? ? out.flush();
? ? ? ? out.close();
? ? ? ? System.out.println(result);
}
}

第二個(gè)servlet:
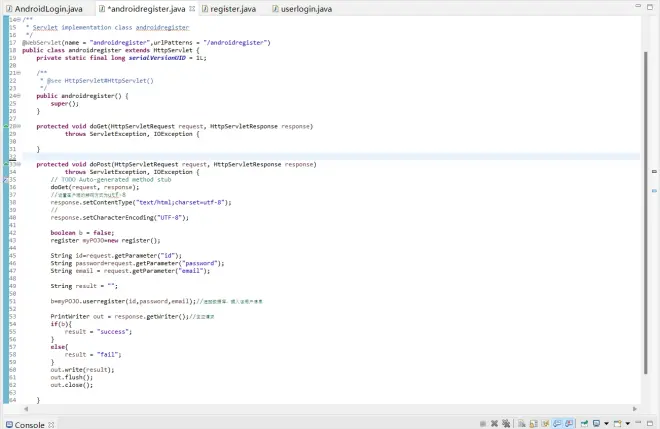
代碼:
package com.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.util.register;
/**
?* Servlet implementation class androidregister
?*/
@WebServlet(name = "androidregister",urlPatterns = "/androidregister")
public class androidregister extends HttpServlet {
private static final long serialVersionUID = 1L;
? ? ? ?
? ? /**
? ? ?* @see HttpServlet#HttpServlet()
? ? ?*/
? ? public androidregister() {
? ? ? ? super();
? ? }
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
//設(shè)置客戶端的解碼方式為utf-8
? ? ? ? response.setContentType("text/html;charset=utf-8");
? ? ? ? //
? ? ? ? response.setCharacterEncoding("UTF-8");
?
? ? ? ? boolean b = false;
? ? ? ? register myPOJO=new register();
?
? ? ? ? String id=request.getParameter("id");
? ? ? ? String password=request.getParameter("password");
? ? ? ? String email = request.getParameter("email");
?
? ? ? ? String result = "";
?
? ? ? ? b=myPOJO.userregister(id,password,email);//連接數(shù)據(jù)庫,插入該用戶信息
?
? ? ? ? PrintWriter out = response.getWriter();//回應(yīng)請求
? ? ? ? if(b){
? ? ? ? ? ? result = "success";
? ? ? ? }
? ? ? ? else{
? ? ? ? ? ? result = "fail";
? ? ? ? }
? ? ? ? out.write(result);
? ? ? ? out.flush();
? ? ? ? out.close();
}
}

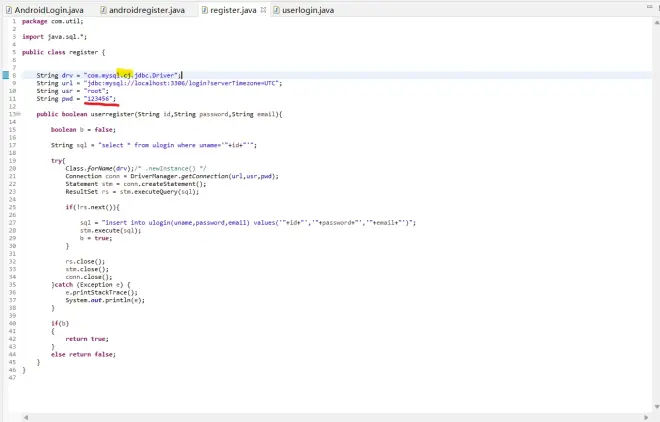
注意:我的MySQL是8.0以上的版本。這里的用戶名和密碼是自己的MySQL的用戶名和密碼
代碼:
package com.util;
import java.sql.*;
public class register {
?
? ? String drv = "com.mysql.cj.jdbc.Driver";
? ? String url = "jdbc:mysql://localhost:3306/login?serverTimezone=UTC";
? ? String usr = "root";
? ? String pwd = "123456";
?
? ? public boolean userregister(String id,String password,String email){
?
? ? ? ? boolean b = false;
?
? ? ? ? String sql = "select * from ulogin where uname='"+id+"'";
?
? ? ? ? try{
Class.forName(drv);/* .newInstance() */
? ? ? ? ? ? Connection conn = DriverManager.getConnection(url,usr,pwd);
? ? ? ? ? ? Statement stm = conn.createStatement();
? ? ? ? ? ? ResultSet rs = stm.executeQuery(sql);
?
? ? ? ? ? ? if(!rs.next()){
?
? ? ? ? ? ? ? ? sql = "insert into ulogin(uname,password,email) values('"+id+"','"+password+"','"+email+"')";
? ? ? ? ? ? ? ? stm.execute(sql);
? ? ? ? ? ? ? ? b = true;
? ? ? ? ? ? }
?
? ? ? ? ? ? rs.close();
? ? ? ? ? ? stm.close();
? ? ? ? ? ? conn.close();
? ? ? ? }catch (Exception e) {
? ? ? ? ? ? e.printStackTrace();
? ? ? ? ? ? System.out.println(e);
? ? ? ? }
?
? ? ? ? if(b)
? ? ? ? {
? ? ? ? ? ? return true;
? ? ? ? }
? ? ? ? else return false;
? ? }
}

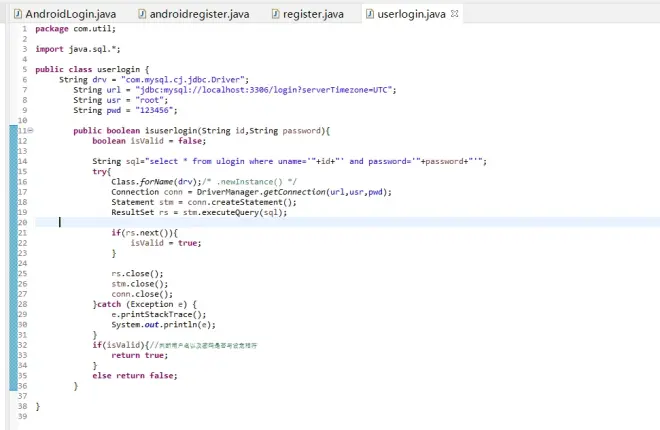
代碼:
package com.util;
import java.sql.*;
public class userlogin {
String drv = "com.mysql.cj.jdbc.Driver";
? ? String url = "jdbc:mysql://localhost:3306/login?serverTimezone=UTC";
? ? String usr = "root";
? ? String pwd = "123456";
?
? ? public boolean isuserlogin(String id,String password){
? ? ? ? boolean isValid = false;
?
? ? ? ? String sql="select * from ulogin where uname='"+id+"' and password='"+password+"'";
? ? ? ? try{
Class.forName(drv);/* .newInstance() */
? ? ? ? ? ? Connection conn = DriverManager.getConnection(url,usr,pwd);
? ? ? ? ? ? Statement stm = conn.createStatement();
? ? ? ? ? ? ResultSet rs = stm.executeQuery(sql);
?
? ? ? ? ? ? if(rs.next()){
? ? ? ? ? ? ? ? isValid = true;
? ? ? ? ? ? }
?
? ? ? ? ? ? rs.close();
? ? ? ? ? ? stm.close();
? ? ? ? ? ? conn.close();
? ? ? ? }catch (Exception e) {
? ? ? ? ? ? e.printStackTrace();
? ? ? ? ? ? System.out.println(e);
? ? ? ? }
? ? ? ? if(isValid){//判斷用戶名以及密碼是否與設(shè)定相符
? ? ? ? ? ? return true;
? ? ? ? }
? ? ? ? else return false;
? ? }
}
《《《《《《《《《《《《數(shù)據(jù)庫設(shè)計(jì)》》》》》》》》》》》》》》》
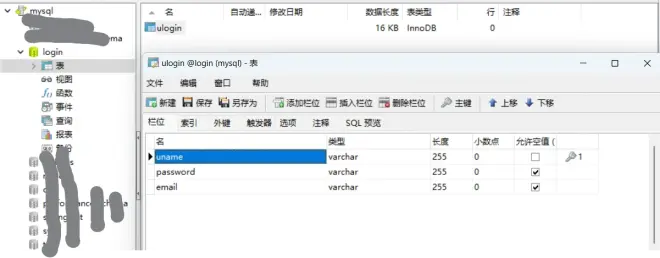