OC最實(shí)用的runtime總結(jié),面試、工作你看我就足夠了!
引言
Objective-C是一門面向?qū)ο蟮木幊陶Z言,在iOS開發(fā)中被廣泛使用。而在Objective-C中,runtime是其核心之一,為開發(fā)者提供了許多強(qiáng)大的特性。本文將總結(jié)OC中最實(shí)用的runtime特性,包括動(dòng)態(tài)添加方法、動(dòng)態(tài)交換方法、獲取類的屬性和方法列表、動(dòng)態(tài)創(chuàng)建類和對(duì)象等,并結(jié)合實(shí)際案例演示其用法,幫助讀者更好地理解和應(yīng)用runtime。
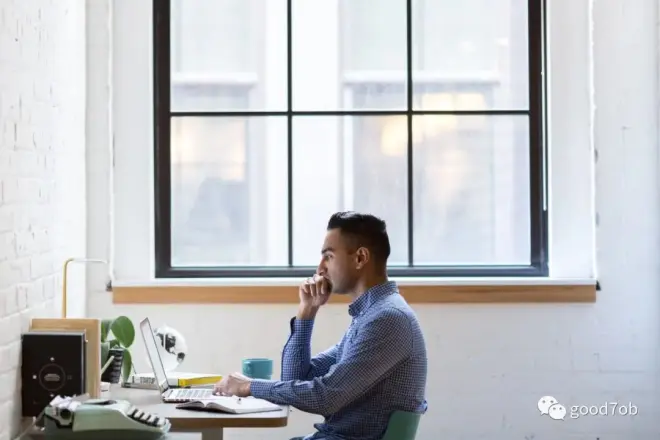
1. 動(dòng)態(tài)添加方法
在OC中,我們可以使用runtime動(dòng)態(tài)地為一個(gè)類添加方法。這在某些情況下非常有用,比如在運(yùn)行時(shí)根據(jù)條件來決定是否添加某個(gè)方法,或者在運(yùn)行時(shí)根據(jù)用戶的操作來動(dòng)態(tài)添加方法。
步驟
導(dǎo)入
<objc/runtime.h>
頭文件。使用
class_addMethod
函數(shù)為類添加方法。
代碼示例
假設(shè)我們有一個(gè)Person類,但是它沒有一個(gè)sayHello
方法。我們可以使用runtime在運(yùn)行時(shí)動(dòng)態(tài)為Person類添加一個(gè)sayHello
方法。
\#import?<objc/runtime.h>
@interface?Person?:?NSObject
@end
@implementation?Person
@end
void?sayHello(id?self,?SEL?_cmd) {
?NSLog(@"Hello!");
}
int?main(int?argc,?const?char?*?argv[]) {
?@autoreleasepool?{
??? ?Person?*person?=?[[Person?alloc]?init];
??? ?// 動(dòng)態(tài)添加sayHello方法
??? ?class_addMethod([Person?class],?@selector(sayHello), (IMP)sayHello,?"v@:");
??? ?// 調(diào)用動(dòng)態(tài)添加的sayHello方法
??? [person?performSelector:@selector(sayHello)];
}
?return?0;
}
運(yùn)行這段代碼,我們會(huì)發(fā)現(xiàn)Person類成功地動(dòng)態(tài)添加了sayHello
方法,并成功調(diào)用了它。
2. 動(dòng)態(tài)交換方法
使用runtime,我們還可以動(dòng)態(tài)交換兩個(gè)方法的實(shí)現(xiàn)。這在某些情況下非常有用,比如在調(diào)試或者實(shí)現(xiàn)A/B測(cè)試時(shí),我們可能需要替換某個(gè)方法的實(shí)現(xiàn)。
步驟
導(dǎo)入
<objc/runtime.h>
頭文件。使用
method_exchangeImplementations
函數(shù)交換兩個(gè)方法的實(shí)現(xiàn)。
代碼示例
假設(shè)我們有一個(gè)Calculator類,它有兩個(gè)方法add:(NSInteger)a to:(NSInteger)b
和subtract:(NSInteger)a from:(NSInteger)b
,我們可以使用runtime在運(yùn)行時(shí)交換這兩個(gè)方法的實(shí)現(xiàn)。
#import <objc/runtime.h>
@interface?Calculator?:?NSObject
-?(NSInteger)add:(NSInteger)a?to:(NSInteger)b;
-?(NSInteger)subtract:(NSInteger)a?from:(NSInteger)b;
@end
@implementation?Calculator
-?(NSInteger)add:(NSInteger)a?to:(NSInteger)b?{
?return?a?+?b;
}
-?(NSInteger)subtract:(NSInteger)a?from:(NSInteger)b?{
?return?b?-?a;
}
@end
int?main(int?argc,?const?char?*?argv[]) {
?@autoreleasepool?{
??? ?Calculator?*calculator?=?[[Calculator?alloc]?init];
??? ?NSInteger?result1?=?[calculator?add:5?to:10];
??? ?NSLog(@"Result1: %ld", (long)result1);?// Output: Result1: 15
??? ?
??? ?// 動(dòng)態(tài)交換add:to:和subtract:from:方法的實(shí)現(xiàn)
??? ?Method?addMethod?=?class_getInstanceMethod([Calculator?class],?@selector(add:to:));
??? ?Method?subtractMethod?=?class_getInstanceMethod([Calculator?class],?@selector(subtract:from:));
??? ?method_exchangeImplementations(addMethod,?subtractMethod);
??? ?
??? ?NSInteger?result2?=?[calculator?add:5?to:10];
??? ?NSLog(@"Result2: %ld", (long)result2);?// Output: Result2: 5
}
?return?0;
}
在上述代碼中,我們成功地動(dòng)態(tài)交換了add:to:
和subtract:from:
方法的實(shí)現(xiàn),從而在運(yùn)行時(shí)改變了方法的行為。
3. 獲取類的屬性和方法列表
使用runtime,我們可以在運(yùn)行時(shí)獲取一個(gè)類的屬性列表和方法列表,這為我們提供了更多的靈活性和動(dòng)態(tài)性。
步驟
導(dǎo)入
<objc/runtime.h>
頭文件。使用
class_copyPropertyList
函數(shù)獲取類的屬性列表。使用
class_copyMethodList
函數(shù)獲取類的方法列表。
代碼示例
假設(shè)我們有一個(gè)Person類,我們可以使用runtime在運(yùn)行時(shí)獲取它的屬性列表和方法列表。
#import <objc/runtime.h>
@interface?Person?:?NSObject
@property?(nonatomic,?strong)?NSString?*name;
@property?(nonatomic,?assign)?NSInteger?age;
-?(void)sayHello;
@end
@implementation?Person
-?(void)sayHello?{
?NSLog(@"Hello, my name is %@ and I am %ld years old.",?self.name, (long)self.age);
}
@end
int?main(int?argc,?const?char?*?argv[]) {
?@autoreleasepool?{
??? ?Person?*person?=?[[Person?alloc]?init];
??? ?person.name?=?@"John";
??? ?person.age?=?30;
??? ?
??? ?// 獲取屬性列表
??? ?unsigned?int?propertyCount;
??? ?objc_property_t?*properties?=?class_copyPropertyList([Person?class],?&propertyCount);
??? ?for?(unsigned?int?i?=?0;?i?<?propertyCount;?i++) {
??? ? ?objc_property_t?property?=?properties[i];
??? ? ?const?char?*propertyName?=?property_getName(property);
??? ? ?NSString?*name?=?[NSString?stringWithUTF8String:propertyName];
??? ? ?NSLog(@"Property: %@",?name);
??? }
??? ?free(properties);
??? ?
??? ?// 獲取方法列表
??? ?unsigned?int?methodCount;
??? ?Method?*methods?=?class_copyMethodList([Person?class],?&methodCount);
??? ?for?(unsigned?int?i?=?0;?i?<?methodCount;?i++) {
??? ? ?Method?method?=?methods[i];
??? ? ?SEL?methodName?=?method_getName(method);
??? ? ?NSString?*name?=?NSStringFromSelector(methodName);
??? ? ?NSLog(@"Method: %@",?name);
??? }
??? ?free(methods);
}
?return?0;
}
在上述代碼中,我們成功地獲取了Person類的屬性列表和方法列表,并輸出了它們的名稱。
4. 動(dòng)態(tài)創(chuàng)建類和對(duì)象
在runtime中,我們還可以動(dòng)態(tài)地創(chuàng)建類和對(duì)象。這在一些需要?jiǎng)討B(tài)生成類和對(duì)象的場(chǎng)景下非常有用,比如在運(yùn)行時(shí)根據(jù)用戶的操作動(dòng)態(tài)生成新的類和對(duì)象。
步驟
導(dǎo)1. 導(dǎo)入
<objc/runtime.h>
頭文件。使用
objc_allocateClassPair
函數(shù)創(chuàng)建一個(gè)新類。使用
class_addMethod
函數(shù)為新類添加方法。使用
objc_registerClassPair
函數(shù)注冊(cè)新類。使用
class_createInstance
函數(shù)創(chuàng)建新類的實(shí)例。
代碼示例
假設(shè)我們需要在運(yùn)行時(shí)動(dòng)態(tài)創(chuàng)建一個(gè)新的Person類,并為該類添加一個(gè)sayHello
方法。
#import <objc/runtime.h>
@interface?Person?:?NSObject
@end
@implementation?Person
@end
void?sayHello(id?self,?SEL?_cmd) {
?NSLog(@"Hello, I am a dynamic Person!");
}
int?main(int?argc,?const?char?*?argv[]) {
?@autoreleasepool?{
??? ?// 動(dòng)態(tài)創(chuàng)建一個(gè)新的Person類
??? ?Class?newClass?=?objc_allocateClassPair([NSObject?class],?"DynamicPerson",?0);
??? ?
??? ?// 為新類添加sayHello方法
??? ?class_addMethod(newClass,?@selector(sayHello), (IMP)sayHello,?"v@:");
??? ?
??? ?// 注冊(cè)新類
??? ?objc_registerClassPair(newClass);
??? ?
??? ?// 創(chuàng)建新類的實(shí)例并調(diào)用sayHello方法
??? ?id?dynamicPerson?=?[[newClass?alloc]?init];
??? [dynamicPerson?performSelector:@selector(sayHello)];
}
?return?0;
}
在上述代碼中,我們成功地動(dòng)態(tài)創(chuàng)建了一個(gè)名為DynamicPerson的新類,并為其添加了sayHello
方法。最后,我們創(chuàng)建了DynamicPerson類的實(shí)例,并成功調(diào)用了sayHello
方法。
結(jié)論
OC的runtime是iOS開發(fā)中非常強(qiáng)大且實(shí)用的特性,它為開發(fā)者提供了許多靈活和動(dòng)態(tài)的操作。在本文中,我們總結(jié)了OC中最實(shí)用的runtime特性,包括動(dòng)態(tài)添加方法、動(dòng)態(tài)交換方法、獲取類的屬性和方法列表、動(dòng)態(tài)創(chuàng)建類和對(duì)象等,并通過實(shí)際代碼示例演示了它們的用法。掌握了這些runtime特性,開發(fā)者可以更好地應(yīng)對(duì)各種復(fù)雜的場(chǎng)景,提高開發(fā)效率,使代碼更加靈活和強(qiáng)大。希望本文對(duì)讀者有所幫助,讓你在面試和工作中信心滿滿!