≈T6
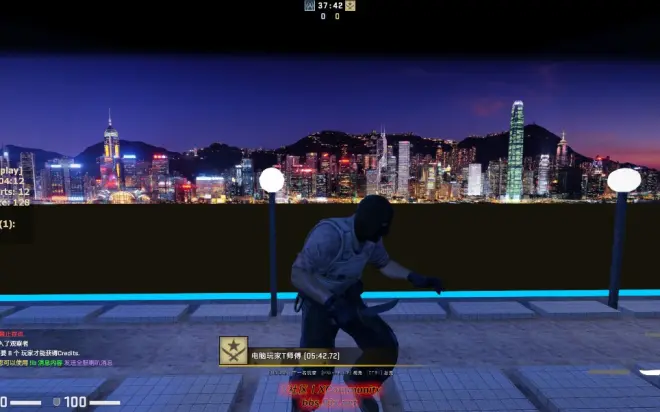
function float[] matrix_multiplication(vector4 mat_1[]; float mat_2[])
{
float result[];
vector4 temp_matrix[];
temp_matrix[0] = set( 0, 0, 0, 0 );
temp_matrix[1] = set( 0, 0, 0, 0 );
temp_matrix[2] = set( 0, 0, 0, 0 );
temp_matrix[3] = set( 0, 0, 0, 0 );
if (4 == len(mat_2))
{
for (int i = 0; i < len(mat_1); i++)
{
for (int j = 0; j < 1; j++)
{
float temp = 0;
for (int k = 0; k <4; k++)
{
temp += mat_1[i][k] * mat_2[k];
}
temp_matrix[i][j] = temp;
}
}
}
result[0] = temp_matrix[0][0];
result[1] = temp_matrix[1][0];
result[2] = temp_matrix[2][0];
result[3] = temp_matrix[3][0];
return result;
};
void DrawLine(int offset;int i;int j;vector4 final_coordinates[])
{
vector start = set(final_coordinates[i + offset][0], final_coordinates[i + offset][1], final_coordinates[i + offset][2]);
vector end = set(final_coordinates[j + offset][0], final_coordinates[j + offset][1], final_coordinates[j + offset][2]);
int a = addpoint(0,start);
int b = addpoint(0,end);
int aa = addprim(0,"polyline",a,b);
}
float rot_speed=1.0f;
float theta = 0;
float cam_dist = 3;
int side_length = 5;
vector4 final_coordinates[];
vector4 coordinates[] ={{ -1, -1, -1, 1 },{ 1, -1, -1, 1 },{ 1, 1, -1, 1 },{ -1, 1, -1, 1 },
{ -1, -1, 1, 1 },{ 1, -1, 1, 1 },{ 1, 1, 1, 1 },{ -1, 1, 1, 1 },
{ -1, -1, -1, -1 },{ 1, -1, -1, -1 },{ 1, 1, -1, -1 },{ -1, 1, -1, -1 },
{ -1, -1, 1, -1 },{ 1, -1, 1, -1 },{ 1, 1, 1, -1 },{ -1, 1, 1, -1 }};
theta += rot_speed*$F/50;
vector4 rotation_matrix[] ;
rotation_matrix[0] = set( 1, 0, 0, 0 );
rotation_matrix[1] = set( 0, 1, 0, 0 );
rotation_matrix[2] = set( 0, 0, cos(theta), -sin(theta) );
rotation_matrix[3] = set( 0, 0, sin(theta), cos(theta) );
for (int i = 0; i < 16; i++)
{
float temp_coordinate_matrix[];
for (int k = 0; k < 4; k++)
{
temp_coordinate_matrix[k] = coordinates[i][k];
}
float rot_temp[] = matrix_multiplication(rotation_matrix, temp_coordinate_matrix);
float perspective_dist = 1 / (cam_dist - rot_temp[len(rot_temp) - 1]);
float proj_temp[];
proj_temp[0] = perspective_dist * rot_temp[0] * side_length / 2;
proj_temp[1] = perspective_dist * rot_temp[1] * side_length / 2;
proj_temp[2] = perspective_dist * rot_temp[2] * side_length / 2;
proj_temp[3] = perspective_dist * rot_temp[3] * side_length / 2;
final_coordinates[i] = set(proj_temp[0], proj_temp[1], proj_temp[2], proj_temp[3]);
}
for (int i = 0; i < 8; i++)
{
if (i < 4)
{
DrawLine(0, i, i + 4,final_coordinates);
DrawLine(0, i, i + 8,final_coordinates);
DrawLine(0, i, (i + 1) % 4,final_coordinates);
DrawLine(0, i + 4, ((i + 1) % 4) + 4,final_coordinates);
DrawLine(8, i, i + 4,final_coordinates);
DrawLine(8, i, (i + 1) % 4,final_coordinates);
DrawLine(8, i + 4, ((i + 1) % 4) + 4,final_coordinates);
}
DrawLine(0, i, i + 8,final_coordinates);
}