Java swing:事件,點(diǎn)即登錄,彈出"點(diǎn)擊了登錄按鈕"的提示窗口
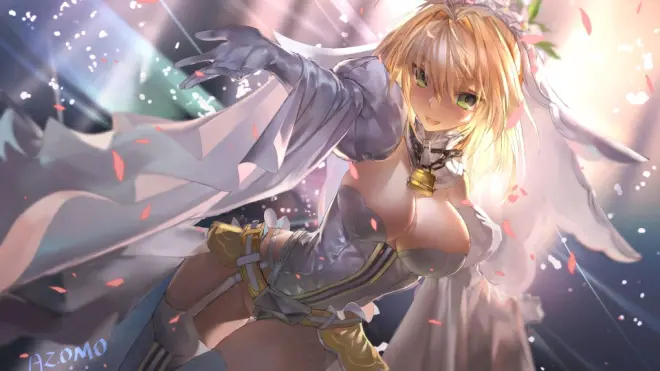
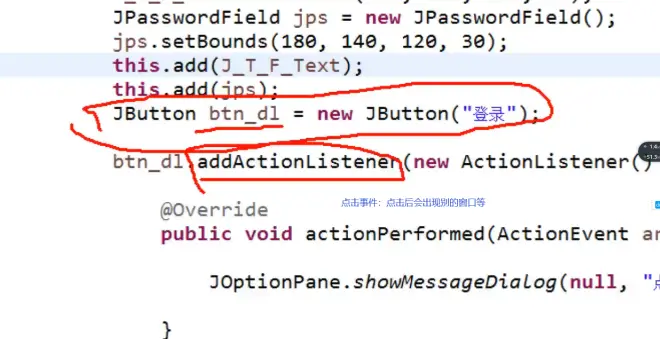
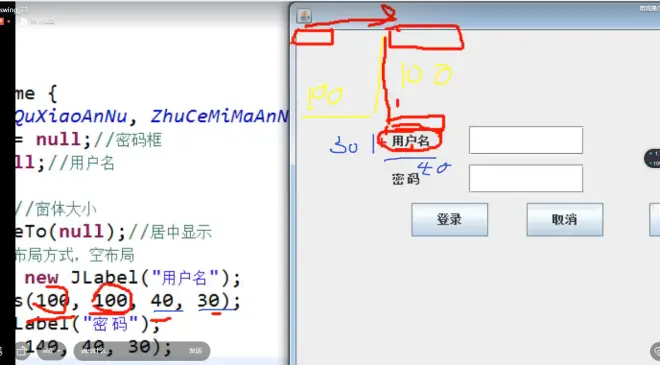
package JDBC;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
/*JFrame:窗口*/
import javax.swing.JFrame;
/*JLabel對象可以顯示文本、圖像或同時(shí)顯示二者。*/
import javax.swing.JLabel;
import javax.swing.JOptionPane;
/*JPanel:面板*/
import javax.swing.JPanel;
/*JPasswordField類是一個(gè)專門處理密碼功能并允許編輯單行文本的組件。*/
import javax.swing.JPasswordField;
/*JTextField類是一個(gè)允許編輯單行文本的組件。*/
import javax.swing.JTextField;
public class mains {
public static void main(String[] args) {
// 創(chuàng)建 JFrame 實(shí)例
JFrame frame = new JFrame("登陸界面");
// Setting the width and height of frame
frame.setSize(350, 200);/* frame.setSize( width,height ) */
/*
* frame.setDefaultCloseOperation()是設(shè)置用戶在此窗體上發(fā)起 "close"
* 時(shí)默認(rèn)執(zhí)行的操作。必須指定以下選項(xiàng)之一:
*
* DO_NOTHING_ON_CLOSE(在 WindowConstants 中定義): 不執(zhí)行任何操作;要求程序在已注冊的
* WindowListener 對象的 windowClosing 方法中處理該操作。
*/
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
/*
* 創(chuàng)建面板,這個(gè)類似于 HTML 的 div 標(biāo)簽 我們可以創(chuàng)建多個(gè)面板并在 JFrame 中指定位置
* 面板中我們可以添加文本字段,按鈕及其他組件。
*/
JPanel panel = new JPanel();
// 添加面板
frame.add(panel);
/*
* 調(diào)用用戶定義的方法并添加組件到面板
*/
placeComponents(panel);
// 設(shè)置界面可見
frame.setVisible(true);
}
private static void placeComponents(JPanel panel) {
/*
* 布局部分我們這邊不多做介紹 這邊設(shè)置布局為 null
*/
panel.setLayout(null);
// 創(chuàng)建 JLabel
JLabel userLabel = new JLabel("用戶名:");
/*
* 這個(gè)方法定義了組件的位置。 setBounds(x, y, width, height) x 和 y 指定左上角的新位置,由 width
* 和 height 指定新的大小。
*/
userLabel.setBounds(10, 20, 80, 25);
panel.add(userLabel);
/*
* 創(chuàng)建文本域用于用戶輸入
*/
JTextField userText = new JTextField(20);
userText.setBounds(100, 20, 165, 25);
panel.add(userText);
// 輸入密碼的文本域
JLabel passwordLabel = new JLabel("密碼:");
passwordLabel.setBounds(10, 50, 80, 25);
panel.add(passwordLabel);
/*
* 這個(gè)類似用于輸入的文本域 但是輸入的信息會(huì)以點(diǎn)號(hào)代替,用于包含密碼的安全性
*/
JPasswordField passwordText = new JPasswordField(20);
passwordText.setBounds(100, 50, 165, 25);
panel.add(passwordText);
// 創(chuàng)建登錄按鈕
JButton loginButton = new JButton("登錄");
loginButton.setBounds(10, 80, 80, 25);
panel.add(loginButton);
loginButton.addActionListener(new ActionListener() {
@Override
/* 下面是ActionListener接口自帶的方法 */
public void actionPerformed(ActionEvent arg0) {
JOptionPane.showMessageDialog(null, "點(diǎn)擊了登錄按鈕'");
}
});
// 創(chuàng)建取消按鈕
JButton quxiaoButton = new JButton("取消");
shijian sj = new shijian();
quxiaoButton.addActionListener(sj);
quxiaoButton.setBounds(110, 80, 80, 25);
panel.add(quxiaoButton);
}
}
/*
?* 下面只是聲明了事件類,并沒有具體去使用,上面的shijian sj=new shijian(); quxiaoButton.
?* addActionListener(sj);是使用方法
?*/
class shijian implements ActionListener {
@Override
public void actionPerformed(ActionEvent arg0) {
JOptionPane.showMessageDialog(null, "點(diǎn)擊了取消按鈕");
}
}