好好好,這樣寫是吧
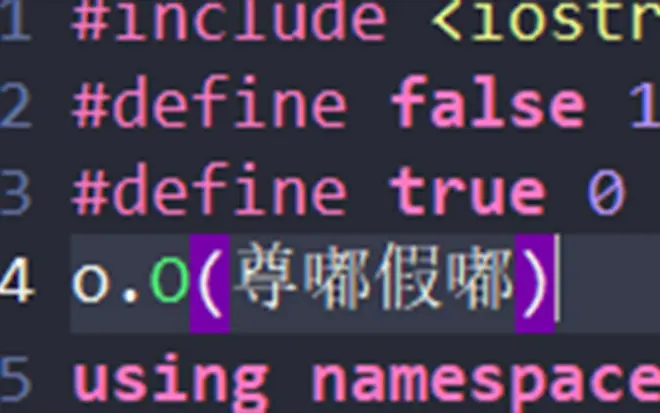
來點(diǎn)硬核抽象——線段樹的正確用法:
#include<bits/stdc++.h>
using namespace std;
namespace IO
{
bool isdigit(const char x)
{
return x>='0'&&x<='9';
}
struct reader
{
template<typename T> reader& operator >> (T &x)
{
x=0;
bool f=0;
char c=getchar();
while (!isdigit(c)) f=c=='-'?1:f,c=getchar();
while (isdigit(c)) x=(x<<1)+(x<<3)+(c^'0'),c=getchar();
x=f?-x:x;
return *this;
}
reader& operator >> (double &x)
{
x=0;
bool f=0;
int t=0;
char c=getchar();
while (!isdigit(c)&&c!='.') f=c=='-'?1:f,c=getchar();
while (isdigit(c)&&c!='.') x=x*10+(c^'0'),c=getchar();
if (c!='.')
{
x=f?-x:x;
return *this;
}
c=getchar();
double y=0;
while (isdigit(c)) y=y*10+(c^48),t++,c=getchar();
while (t--) y/=10.0;
x+=y;
x=f?-x:x;
return *this;
}
reader& operator >> (char &x)
{
x=getchar();
while (x=='\n'||x==' '||x=='\r') x=getchar();
return *this;
}
reader& operator >> (char *x)
{
int l=0;
char c=getchar();
while (c==' '||c=='\n'||c=='\r') c=getchar();
while (c!=' '&&c!='\n'&&c!='\r') x[++l]=c,c=getchar();
return *this;
}
void getline(char *x)
{
int l=0;
char c=getchar();
while (c=='\n'||c=='\r') c=getchar();
while (c!='\n'&&c!='\r') x[++l]=c,c=getchar();
}
reader& operator >> (string &x)
{
x="";
char c=getchar();
while (c==' '||c=='\n'||c=='\r') c=getchar();
while (c!=' '&&c!='\n'&&c!='\r') x=x+c,c=getchar();
return *this;
}
void getline(string &x)
{
x="";
char c=getchar();
while (c=='\n'||c=='\r') c=getchar();
while (c!='\n'&&c!='\r') x=x+c,c=getchar();
}
}cin;
const char endl='\n',tab='\t',null='\0';
struct writer
{
template<typename T>writer& operator << (T x)
{
if (x<0) putchar('-'),x=-x;
int s[40],t=0;
while (x) s[++t]=x%10,x/=10;
if (!t) putchar('0');
while (t) putchar(s[t--]+'0');
return *this;
}
writer& operator << (double x)
{
if (x<0) putchar('-'),x=-x;
int y=x;
x-=y*1.0;
int s[40],t=0;
while (y) s[++t]=y%10,y/=10;
if (!t) putchar('0');
while (t) putchar(s[t--]+'0');
putchar('.');
for (int i=0;i<6;i++) x*=10;
y=x;
while (y) s[++t]=y%10,y/=10;
for (int i=0;i<6-t;i++) putchar('0');
while (t) putchar(s[t--]+'0');
return *this;
}
writer& operator << (const char x)
{
putchar(x);
return *this;
}
writer& operator << (char *x)
{
int t=0;
while (x[t]) putchar(x[t++]);
return *this;
}
writer& operator << (const char *x)
{
int t=0;
while (x[t]) putchar(x[t++]);
return *this;
}
writer& operator << (string x)
{
int t=0,l=x.size();
while (t<l) putchar(x[t++]);
return *this;
}
}cout;
}
namespace main_code
{
const int N=1e5+10;
int a[N],sum[N<<2],ad[N<<2];
int tot=0;
void build(int k,int l,int r)
{
if (l==r)
{
sum[k]=a[++tot];
return;
}
int mid=l+r>>1;
build(k<<1,l,mid);
build(k<<1|1,mid+1,r);
sum[k]=sum[k<<1]+sum[k<<1|1];
}
void add(int k,int l,int r,int z)
{
ad[k]+=z;
sum[k]+=z*(r-l+1);
}
void pushdown(int k,int l,int r,int mid)
{
add(k<<1,l,mid,ad[k]);
add(k<<1|1,mid+1,r,ad[k]);
ad[k]=0;
}
void modify(int k,int l,int r,int x,int y,int z)
{
if (r<x||l>y) return;
if (l>=x&&r<=y)
{
add(k,l,r,z);
return;
}
int mid=l+r>>1;
if (ad[k]) pushdown(k,l,r,mid);
if (x<=mid) modify(k<<1,l,mid,x,y,z);
if (mid<y) modify(k<<1|1,mid+1,r,x,y,z);
sum[k]=sum[k<<1]+sum[k<<1|1];
}
int query(int k,int l,int r,int x,int y)
{
if (r<x||l>y) return 0;
if (l>=x&&r<=y) return sum[k];
int mid=l+r>>1;
if (ad[k]) pushdown(k,l,r,mid);
int ans=0;
if (x<=mid) ans+=query(k<<1,l,mid,x,y);
if (mid<y) ans+=query(k<<1|1,mid+1,r,x,y);
return ans;
}
void main()
{
IO::cin>>a[1]>>a[2];
build(1,1,2);
IO::cout<<query(1,1,2,1,2)<<IO::endl;
}
}
signed main()
{
main_code::main();
return 0;
}
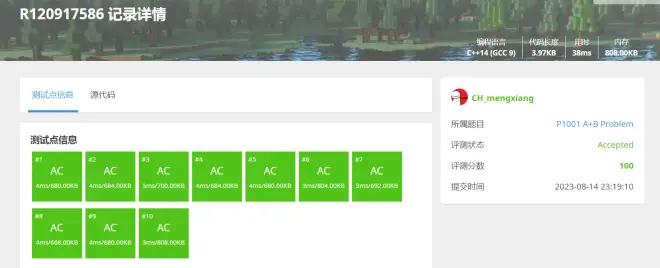