無監(jiān)督學(xué)習(xí)Kmeans聚類(代碼重傳
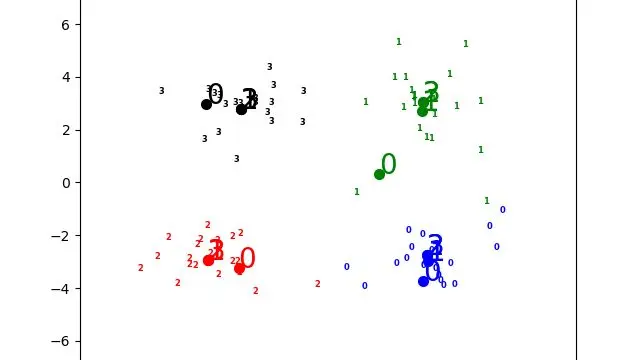
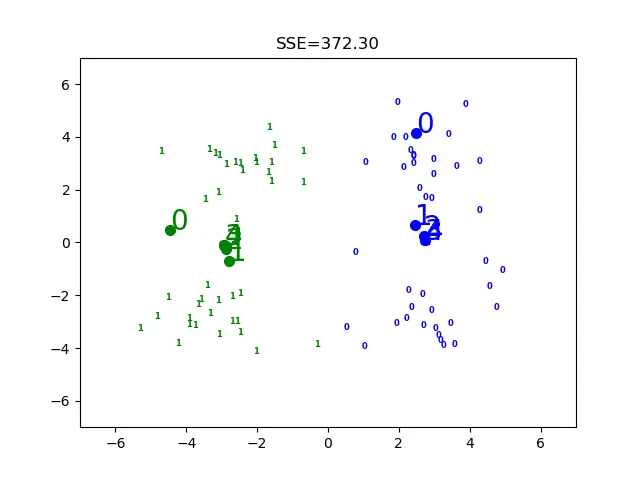
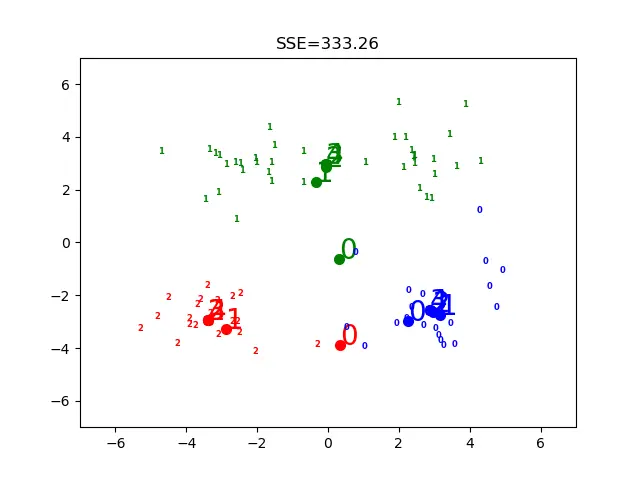
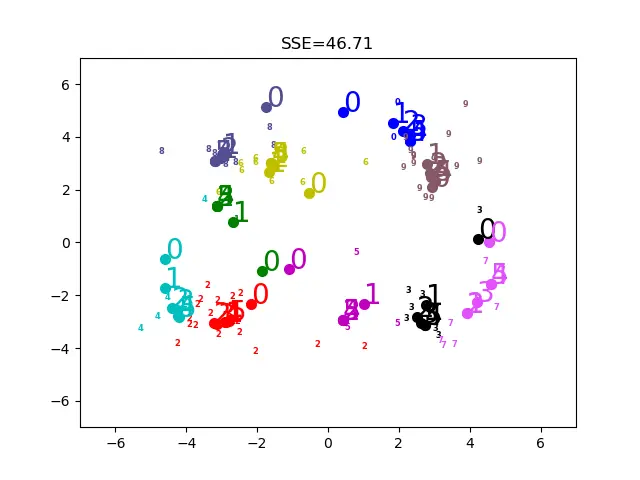
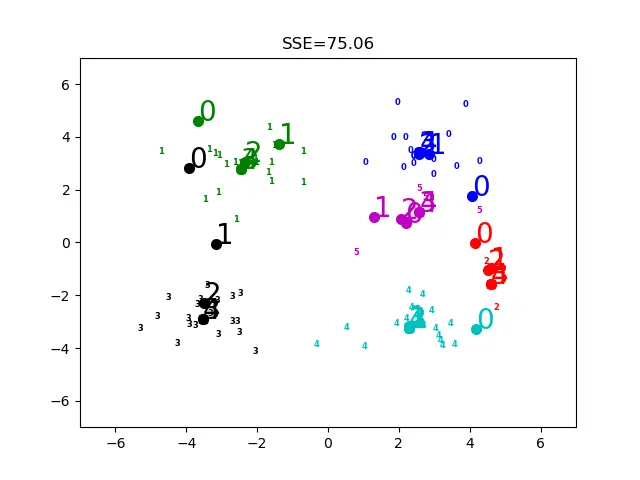
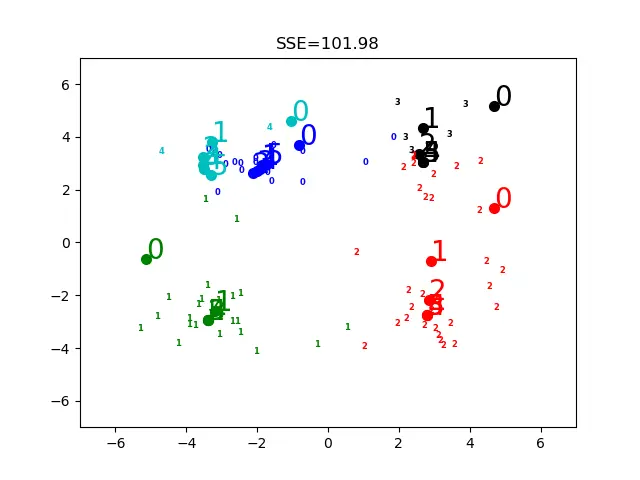
上面是k值不同時(shí)候的情況
共兩個(gè)代碼文件:
kmeans.py
# -*- coding: utf-8 -*-
import numpy as np
import matplotlib.pyplot as plt
class KMeansClassifier():
"""
這是一個(gè)kmean分類器
"""
# k initcent='random'初始化聚類中心的方法 max_iter=20訓(xùn)練的次數(shù)
def __init__(self, k=3, initcent='random', max_iter=20):
self._k = k # 接外面?zhèn)鬟M(jìn)來的
self._initCent = initcent
self._max_iter = max_iter
self._clusterAssment = None
self._labels = None
self._sse = None
def draw_pic(self, step):
colors = ['b', 'g', 'r', 'k', 'c', 'm', 'y', '#e24fff', '#524C90', '#845868']
for i in range(self._k):
plt.scatter(self._centroids[i, 0], self._centroids[i, 1], marker='o', color=colors[i], linewidths=2)
plt.text(s=step.__str__(), x=self._centroids[i, 0], y=self._centroids[i, 1], color=colors[i], size=20)
# print(arrA, arrB) # 前一個(gè)是聚類中心的x y坐標(biāo),后一個(gè)散點(diǎn)的x y坐標(biāo) 歐拉距離計(jì)算
def _cal_e_dist(self, arr_a, arr_b):
"""
功能:歐拉距離計(jì)算
輸入:兩個(gè)一維數(shù)組
"""
return np.math.sqrt(sum(np.power(arr_a - arr_b, 2)))
def _calMDist(self, arr_a, arr_b):
"""
功能:曼哈頓距離距離計(jì)算
輸入:兩個(gè)一維數(shù)組
arrA是聚類中心的x y坐標(biāo),arrB是散點(diǎn)的x y坐標(biāo)
"""
return sum(np.abs(arr_a - arr_b))
# centroids[0][0]保存的是第一個(gè)聚類中心的x值 centroids[0][1]保存的是第一個(gè)聚類中心的y值
def _randCent(self, data_x, k):
"""
功能:隨機(jī)選取k個(gè)質(zhì)心
輸出:centroids #返回一個(gè)k*n的質(zhì)心矩陣
"""
n = data_x.shape[1] # 獲取特征的維數(shù)
# print(n) # 這里是2,因?yàn)樯Ⅻc(diǎn)是二維的
centroids = np.empty((k, n)) # 使用numpy生成一個(gè)k*n的矩陣,用于存儲(chǔ)質(zhì)心坐標(biāo)
for j in range(n):
min_j = min(data_x[:, j]) # 取到第j維最小的數(shù)
range_j = float(max(data_x[:, j] - min_j)) # 取到第j維的坐標(biāo)變化范圍
# 使用flatten拉平嵌套列表(nested list)
centroids[:, j] = (min_j + range_j * np.random.rand(k, 1)).flatten() # 隨機(jī)選取第j維的坐標(biāo)(保證坐標(biāo)不超過數(shù)據(jù))
# print(centroids)
"""
# 指定初始坐標(biāo)
centroids = np.empty((k, data_x.shape[1]))
for i in range(k):
centroids[i][0] = i
centroids[i][1] = i
# print(centroids)"""
return centroids
# self._centroids[i, :] # 計(jì)算取出的第i類樣本點(diǎn)的各個(gè)坐標(biāo)均值
# self._labels # 樣本點(diǎn)所屬的類的索引值minIndex
# self._sse # 該點(diǎn)與其聚類中心的平方誤差minDist**2
def fit(self, data_x):
"""
輸入:一個(gè)m*n維的矩陣
本例是m*2
"""
if not isinstance(data_x, np.ndarray) or isinstance(data_x, np.matrixlib.defmatrix.matrix):
try:
data_x = np.asarray(data_x)
except:
raise TypeError("numpy.ndarray resuired for data_X")
m = data_x.shape[0] # 獲取樣本的個(gè)數(shù)m(79) data_X.shape[1]是維數(shù)n=2
# 準(zhǔn)備一個(gè)m*2的二維矩陣,矩陣第一列存儲(chǔ)樣本點(diǎn)所屬的類的索引值 第二列存儲(chǔ)該點(diǎn)與其聚類中心的平方誤差
self._clusterAssment = np.zeros((m, 2))
# 返回centroids[0][0]第0中心的x centroids[0][1]第0中心的y
if self._initCent == 'random':
self._centroids = self._randCent(data_x, self._k)
self.draw_pic(0) # =====================================================================================
# self._max_iter是最多訓(xùn)練的次數(shù)
for _iter in range(self._max_iter): # ============================對(duì)每次訓(xùn)練
shou_lian = True # 這個(gè)散點(diǎn)所跟隨的聚類中心是不是變了
# 將每個(gè)樣本點(diǎn)分配到離它最近的質(zhì)心所屬的族
for i in range(m): # ========================================對(duì)每個(gè)散點(diǎn)
min_dist = np.inf # 首先將minDist置為一個(gè)無窮大的數(shù)
min_index = -1 # 將最近質(zhì)心的下標(biāo)置為-1
# 次迭代用于尋找最近的質(zhì)心
for j in range(self._k): # ===============================對(duì)每個(gè)聚類中心
# 第j個(gè)聚類中心的x y坐標(biāo) # ab第i個(gè)散點(diǎn)的x y坐標(biāo)
arr_a = self._centroids[j, :]
arr_b = data_x[i, :]
dist_ab = self._cal_e_dist(arr_a, arr_b)
if dist_ab < min_dist:
min_dist = dist_ab # 更新
min_index = j # 記錄
# 第一列索引值 第二列平方誤差 共m行
if self._clusterAssment[i, 0] != min_index or self._clusterAssment[i, 1] > min_dist**2:
self._clusterAssment[i, :] = min_index, min_dist**2
shou_lian = False
if shou_lian: # 收斂,結(jié)束迭代
break
self.old_temp = []
# 更新 將每個(gè)類中的點(diǎn)的均值作為新的聚類中心的坐標(biāo)
for i in range(self._k): # =====================================對(duì)于聚類中心數(shù)量
value = np.nonzero(self._clusterAssment[:, 0] == i) # 取出散點(diǎn)的索引值
self.old_temp[:] = self._centroids[i, :]
self._centroids[i, :] = np.mean(data_x[value[0]], axis=0) # 計(jì)算第i中心所有樣本點(diǎn)的各個(gè)坐標(biāo)均值
if self._centroids[i, 0] != self._centroids[i, 0]: # 是空值nan,沒有人追隨著個(gè)中心點(diǎn)
self._centroids[i, :] = self.old_temp[:]
# 這里打印出每步的聚類中心的位置
colors = ['b', 'g', 'r', 'k', 'c', 'm', 'y', '#e24fff', '#524C90', '#845868']
if _iter==0:
for i in range(self._k): # 對(duì)每個(gè)聚類
index = np.nonzero(self._clusterAssment[:, 0] == i)[0]
x0 = data_x[index, 0] # x坐標(biāo)
x1 = data_x[index, 1] # y坐標(biāo)
for j in range(len(x0)): # 對(duì)所有這些點(diǎn)
plt.text(x0[j], x1[j], str(i), color=colors[i], fontdict={'weight': 'bold', 'size': 6})
self.draw_pic(_iter+1)
self._labels = self._clusterAssment[:, 0] # 第一列存樣本點(diǎn)所屬的類的索引值minIndex
self._sse = sum(self._clusterAssment[:, 1]) # 第二列存該點(diǎn)與其聚類中心的平方誤差minDist**2
# print('step', _iter, '\n', self._centroids, '\n', self._labels, '\n', self._sse)
# preds[:] 各個(gè)點(diǎn)依次的預(yù)測(cè)聚類中心
def predict(self, x): # 根據(jù)聚類結(jié)果,預(yù)測(cè)新輸入數(shù)據(jù)所屬的族
# 類型檢查
if not isinstance(x, np.ndarray):
try:
x = np.asarray(x)
except:
raise TypeError("numpy.ndarray required for X")
m = x.shape[0] # m代表樣本數(shù)量
preds = np.empty((m,))
for i in range(m): # 將每個(gè)樣本點(diǎn)分配到離它最近的質(zhì)心所屬的族
min_dist = np.inf
for j in range(self._k):
dist_j_i = self._cal_e_dist(self._centroids[j, :], x[i, :])
if dist_j_i < min_dist:
min_dist = dist_j_i
preds[i] = j
return preds[:]
class biKMeansClassifier():
"""
這是一個(gè)二分 k-means
"""
def __init__(self, k=3):
self._k = k
self._centroids = None
self._clusterAssment = None
self._labels = None
self._sse = None
def _calEDist(self, arr_a, arr_b):
"""
功能:歐拉距離距離計(jì)算
輸入:兩個(gè)一維數(shù)組
"""
# print(arrA, arrB) # 前一個(gè)是聚類中心的x y坐標(biāo),后一個(gè)散點(diǎn)的x y坐標(biāo)
return np.math.sqrt(sum(np.power(arr_a - arr_b, 2)))
def fit(self, X):
m = X.shape[0]
self._clusterAssment = np.zeros((m, 2))
centroid0 = np.mean(X, axis=0).tolist()
cent_list = [centroid0]
for j in range(m): # 計(jì)算每個(gè)樣本點(diǎn)與質(zhì)心之間初始的平方誤差
self._clusterAssment[j, 1] = self._calEDist(np.asarray(centroid0), X[j, :]) ** 2
while(len(cent_list) < self._k):
lowest_sse = np.inf
# 嘗試劃分每一族,選取使得誤差最小的那個(gè)族進(jìn)行劃分
for i in range(len(cent_list)):
index_all = self._clusterAssment[:, 0] # 取出樣本所屬簇的索引值
value = np.nonzero(index_all == i) # 取出所有屬于第i個(gè)簇的索引值
pts_in_curr_cluster = X[value[0], :] # 取出屬于第i個(gè)簇的所有樣本點(diǎn)
clf = KMeansClassifier(k=2)
clf.fit(pts_in_curr_cluster)
# 劃分該族后,所得到的質(zhì)心、分配結(jié)果及誤差矩陣
centroid_mat, split_clust_ass = clf._centroids, clf._clusterAssment
sse_split = sum(split_clust_ass[:, 1])
index_all = self._clusterAssment[:, 0]
value = np.nonzero(index_all == i)
sse_not_split = sum(self._clusterAssment[value[0],1])
if (sse_split + sse_not_split) < lowest_sse:
best_cent_to_split = i
best_new_cents = centroid_mat
best_clust_ass = split_clust_ass.copy()
lowest_sse = sse_split + sse_not_split
# 該族被劃分成兩個(gè)子族后,其中一個(gè)子族的索引變?yōu)樵宓乃饕?/p>
# 另一個(gè)子族的索引變?yōu)閘en(cent_list),然后存入centList
best_clust_ass[np.nonzero(best_clust_ass[:, 0] == 1)[0], 0] = len(cent_list)
best_clust_ass[np.nonzero(best_clust_ass[:, 0] == 0)[0], 0] = best_cent_to_split
cent_list[best_cent_to_split] = best_new_cents[0, :].tolist()
cent_list.append(best_new_cents[1, :].tolist())
self._clusterAssment[np.nonzero(self._clusterAssment[:,0] == best_cent_to_split)[0], :] = best_clust_ass
self._labels = self._clusterAssment[:, 0]
self._sse = sum(self._clusterAssment[:, 1])
self._centroids = np.asarray(cent_list)
def predict(self, x): # 根據(jù)聚類結(jié)果,預(yù)測(cè)新輸入數(shù)據(jù)所屬的族
# 類型檢查
if not isinstance(x, np.ndarray):
try:
x = np.asarray(x)
except:
raise TypeError("numpy.ndarray required for X")
m = x.shape[0] # m代表樣本數(shù)量
preds = np.empty((m,))
for i in range(m): # 將每個(gè)樣本點(diǎn)分配到離它最近的質(zhì)心所屬的族
min_dist = np.inf
for j in range(self._k):
dist_j_i = self._calEDist(self._centroids[j, :], x[i, :])
if dist_j_i < min_dist:
min_dist = dist_j_i
preds[i] = j
return preds
run.py
# -*- coding: utf-8 -*-
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from kmeans import KMeansClassifier
# 加載數(shù)據(jù)集,DataFrame格式,最后將返回為一個(gè)matrix格式
def loadDataset(infile):
df = pd.read_csv(infile, sep='\t', header=0, dtype=str, na_filter=False)
# print(df)
return np.array(df).astype(np.float)
if __name__ == "__main__":
data_X = loadDataset(r"data/testSet.txt")
# print(data_X[0][0]) # 是一個(gè)list,data_X[0][0]是第一個(gè)點(diǎn)的x坐標(biāo) data_X[0][1]是第一個(gè)點(diǎn)的y坐標(biāo)
k = 4 # kmean的k
max_iter = 5 # kmean最大訓(xùn)練次數(shù)(100可以滿足大多數(shù)要求)
clf = KMeansClassifier(k, max_iter=max_iter)
clf.fit(data_X)
labels = clf._labels # 由上一行計(jì)算得出
sse = clf._sse # 由上上一行計(jì)算得出
cents = clf._centroids # 由上上上一行計(jì)算得出
colors = ['b', 'g', 'r', 'k', 'c', 'm', 'y', '#e24fff', '#524C90', '#845868']
plt.title("SSE={:.2f}".format(sse))
plt.axis([-7, 7, -7, 7])
outname = "./result/k_clusters" + str(k) + ".png"
plt.savefig(outname)
# plt.show()
fig1 = plt.figure()
ax1 = fig1.add_subplot(111)
for i in range(k): # 對(duì)每個(gè)聚類
index = np.nonzero(labels == i)[0]
x0 = data_X[index, 0] # x坐標(biāo)
x1 = data_X[index, 1] # y坐標(biāo)
for j in range(len(x0)): # 對(duì)所有這些點(diǎn)
ax1.text(x0[j], x1[j], str(i), color=colors[i], fontdict={'weight': 'bold', 'size': 6})
ax1.scatter(cents[i, 0], cents[i, 1], marker='o', color=colors[i], linewidths=5)
plt.show()
# print('[[2, 2], [-4, -4]]兩個(gè)點(diǎn)的預(yù)測(cè)結(jié)果=', clf.predict([[2, 2], [-4, -4]])) # 預(yù)測(cè)的輸出
測(cè)試用的數(shù)據(jù)集
1.658985 4.285136
-3.453687 3.424321
4.838138 -1.151539
-5.379713 -3.362104
0.972564 2.924086
-3.567919 1.531611
0.450614 -3.302219
-3.487105 -1.724432
2.668759 1.594842
-3.156485 3.191137
3.165506 -3.999838
-2.786837 -3.099354
4.208187 2.984927
-2.123337 2.943366
0.704199 -0.479481
-0.392370 -3.963704
2.831667 1.574018
-0.790153 3.343144
2.943496 -3.357075
-3.195883 -2.283926
2.336445 2.875106
-1.786345 2.554248
2.190101 -1.906020
-3.403367 -2.778288
1.778124 3.880832
-1.688346 2.230267
2.592976 -2.054368
-4.007257 -3.207066
2.257734 3.387564
-2.679011 0.785119
0.939512 -4.023563
-3.674424 -2.261084
2.046259 2.735279
-3.189470 1.780269
4.372646 -0.822248
-2.579316 -3.497576
1.889034 5.190400
-0.798747 2.185588
2.836520 -2.658556
-3.837877 -3.253815
2.096701 3.886007
-2.709034 2.923887
3.367037 -3.184789
-2.121479 -4.232586
2.329546 3.179764
-3.284816 3.273099
3.091414 -3.815232
-3.762093 -2.432191
3.542056 2.778832
-1.736822 4.241041
2.127073 -2.983680
-4.323818 -3.938116
3.792121 5.135768
-4.786473 3.358547
2.624081 -3.260715
-4.009299 -2.978115
2.493525 1.963710
-2.513661 2.642162
1.864375 -3.176309
-3.171184 -3.572452
2.894220 2.489128
-2.562539 2.884438
3.491078 -3.947487
-2.565729 -2.012114
3.332948 3.983102
-1.616805 3.573188
2.280615 -2.559444
-2.651229 -3.103198
2.321395 3.154987
-1.685703 2.939697
3.031012 -3.620252
-4.599622 -2.185829
4.196223 1.126677
-2.133863 3.093686
4.668892 -2.562705
-2.793241 -2.149706
2.884105 3.043438
-2.967647 2.848696
4.479332 -1.764772
-4.905566 -2.911070