面向所有人的chatGPT 專屬程序員入門教程
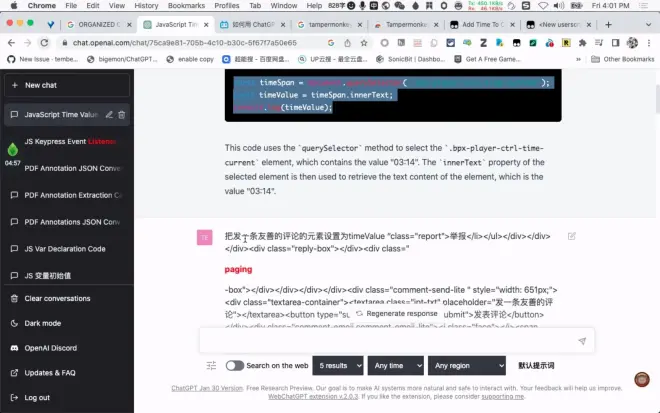
> 獲取03:14 這種數(shù)值的JavaScript 代碼“ <input class="bpx-player-ctrl-time-seek" value="00:00" style="display: none;"> <div class="bpx-player-ctrl-time-label"> <span class="bpx-player-ctrl-time-current">03:14</span> <span class="bpx-player-ctrl-time-divide">/</span> <span class="bpx-player-ctrl-time-duration">18:17</span> </div> </div></div>”
---
You can use the following JavaScript code to extract the value "03:14":
javascript
```javascript
const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
const timeValue = timeSpan.innerText;
console.log(timeValue);
```
This code uses the `querySelector` method to select the `.bpx-player-ctrl-time-current` element, which contains the value "03:14". The `innerText` property of the selected element is then used to retrieve the text content of the element, which is the value "03:14".
---
> 把發(fā)一條友善的評(píng)論的元素設(shè)置為timeValue “class="report">舉報(bào)</li></ul></div></div></div><div class="reply-box"></div><div class="paging-box"></div></div></div></div><div class="comment-send-lite " style="width: 651px;"><div class="textarea-container"><textarea class="ipt-txt" placeholder="發(fā)一條友善的評(píng)論"></textarea><button type="submit" class="comment-submit">發(fā)表評(píng)論</button></div><div class="comment-emoji comment-emoji-lite"><i class="face"></i><span class="text">表情</span></div></div><div class="loading-state"></div><div class="bottom-page paging-box-big"></”
---
You can use the following JavaScript code to set the value of `timeValue` as the content of the textarea element with class `ipt-txt`:
javascript
```javascript
const textarea = document.querySelector('.ipt-txt');
textarea.value = timeValue;
```
This code uses the `querySelector` method to select the `.ipt-txt` element, which is the textarea where you want to set the value of `timeValue`. The `value` property of the selected element is then set to the value of `timeValue`.
---
> 合并上面的代碼
---
Here's the combined code for extracting the value "03:14" and setting it as the content of the textarea element with class `ipt-txt`:
javascript
```javascript
const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
const timeValue = timeSpan.innerText;
const textarea = document.querySelector('.ipt-txt');
textarea.value = timeValue;
```
---
> 按t 時(shí)執(zhí)行這些代碼
---
You can use the following code to execute the previous combined code when the "t" key is pressed:
javascript
```javascript
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT") {
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText;
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
?}
});
```
This code uses the `addEventListener` method to listen for a "keydown" event. When the "keydown" event occurs, the code checks if the `event.code` property is equal to "KeyT". If it is, the code for extracting the value "03:14" and setting it as the content of the textarea element with class `ipt-txt` is executed.
---
> 執(zhí)行代碼后激活內(nèi)容輸入?yún)^(qū)域
---
You can use the following code to activate the textarea element after the code is executed:
javascript
```javascript
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT") {
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText;
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but with the addition of a call to the `focus` method on the selected textarea element. The `focus` method activates the textarea and places the cursor inside it, allowing the user to immediately start typing.
---
> 取消網(wǎng)頁(yè)默認(rèn)行為
---
You can use the following code to cancel the default behavior of the "t" key in the web page:
javascript
```javascript
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT") {
??event.preventDefault();
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText;
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but with the addition of a call to the `preventDefault` method on the event object. The `preventDefault` method cancels the default behavior of the "t" key in the web page. This is useful if you want to prevent the key from triggering any other events or actions that it would normally trigger.
---
> 時(shí)間后面加個(gè)空格
---
You can modify the code to add a space after the time value like this:
javascript
```javascript
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT") {
??event.preventDefault();
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText + " ";
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but with the addition of a space character at the end of the `timeValue` string. The modified `timeValue` string will now have a space after the time value when it is set as the value of the textarea element.
---
> 按t 改成按shift 加 t
---
You can modify the code to trigger the event when the "Shift" key and the "t" key are pressed together like this:
javascript
```javascript
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT" && event.shiftKey) {
??event.preventDefault();
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText + " ";
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but with the addition of a check for the `shiftKey` property of the event object. The `shiftKey` property is `true` if the "Shift" key is pressed when the event is triggered. The event will only be triggered if both the "Shift" key and the "t" key are pressed together.
---
> 改成tampermonkey 代碼
---
Here's the code for Tampermonkey:
javascript
```javascript
// ==UserScript==
// @name????Add Time To Comment
// @namespace??http://www.example.com/
// @include???http://www.example.com/
// @version???1.0
// @grant????none
// ==/UserScript==
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT" && event.shiftKey) {
??event.preventDefault();
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText + " ";
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but with the addition of the Tampermonkey header information at the top. The header information is used by Tampermonkey to identify the script and control how it operates. The `@include` directive specifies the URL pattern that the script should be run on. In this case, the script will be run on the `http://www.example.com/` URL.
---
> 只在https://www.bilibili.com/ 加載
---
Here's the updated code for Tampermonkey to run only on `https://www.bilibili.com/`:
javascript
```javascript
// ==UserScript==
// @name????Add Time To Comment
// @namespace??http://www.example.com/
// @include???https://www.bilibili.com/
// @version???1.0
// @grant????none
// ==/UserScript==
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT" && event.shiftKey) {
??event.preventDefault();
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText + " ";
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but the `@include` directive has been updated to `https://www.bilibili.com/`, so the script will only run on that specific URL.
---
> 在在https://www.bilibili.com/ 所有頁(yè)面加載
---
Here's the updated code for Tampermonkey to run on all pages of `https://www.bilibili.com/`:
javascript
```javascript
// ==UserScript==
// @name????Add Time To Comment
// @namespace??http://www.example.com/
// @include???https://www.bilibili.com/*
// @version???1.0
// @grant????none
// ==/UserScript==
document.addEventListener("keydown", function(event) {
?if (event.code === "KeyT" && event.shiftKey) {
??event.preventDefault();
??const timeSpan = document.querySelector('.bpx-player-ctrl-time-current');
??const timeValue = timeSpan.innerText + " ";
??const textarea = document.querySelector('.ipt-txt');
??textarea.value = timeValue;
??textarea.focus();
?}
});
```
This code is the same as the previous code, but the `@include` directive has been updated to `https://www.bilibili.com/*`, so the script will run on all pages of `https://www.bilibili.com/`.
---
> summarize a prompt for the current code
---
The current code is a script for Tampermonkey that adds the current time to a comment section on the website `https://www.bilibili.com/`. The script activates when the user presses the `Shift` key and the `T` key, which adds the time displayed on the page (in the format `hh:mm`) followed by a space to the textarea for the comment. The script is designed to run on all pages of `https://www.bilibili.com/` and cancels the default behavior of the web page when the keys are pressed.
---
2023-02-03 17:07:54 CST|23m 48s
Keywords:
程序、插件、時(shí)間戳、網(wǎng)站、自定義、規(guī)則、編輯器、輸入框、自然語(yǔ)言、頁(yè)面加載
Transcript:
看了這個(gè)視頻之后,你可能就能夠用掐的 GBT 打造成你專屬的程序員。比如現(xiàn)在網(wǎng)頁(yè),你可以用按 shift t 把它的時(shí)間戳插入在評(píng)論里,這個(gè)是 b 站默認(rèn)沒有的功能?,F(xiàn)在實(shí)際上是程序發(fā)揮的作用。但是你怎么樣自己來(lái)做到這件事情,只是通過(guò)這個(gè)例子來(lái)說(shuō)明這么一種方式。你有自己的自定義需求,也可以通過(guò)類似的方式來(lái)實(shí)現(xiàn)。比如現(xiàn)在這個(gè)東西,把這里的時(shí)間插入到輸入框里面,你現(xiàn)在來(lái)做。先把頁(yè)面給保存下來(lái)。
對(duì),因?yàn)楝F(xiàn)在剛才改了一下,因?yàn)橐褷顟B(tài)改成默認(rèn)的,這樣他才他的初始的狀態(tài)才比較正確?,F(xiàn)在把這個(gè)頁(yè)面保存下來(lái),而這里保存頁(yè)面之后,你隨便用一個(gè)什么編輯器打開,比如最簡(jiǎn)單的這種,他好像他還給加,還給解析了一下。
make plantest。先不管了,我之前我因?yàn)槲易约毫?xí)慣用別的方式,我剛才沒用這種。反正你隨便找一個(gè)編輯器,按這種方式打開,隨便找個(gè)編輯器,現(xiàn)在你想先做的,你就想得到值。171748,你就在里邊搜一期, T8 對(duì)吧。你就把它附近的,你不用管它什么規(guī)則,你大概是把附近的只要包含它內(nèi)容給復(fù)制出來(lái),把它輸入到洽的 APP 里。這種獲取。因?yàn)橹拔視r(shí)間和這是不一樣的,你就替換成這個(gè)時(shí)間就行了。把代碼粘貼到這里,他就會(huì)就會(huì)把這個(gè)給寫出來(lái),對(duì)吧。這是這一步。下面得到了值之后,我們可以試一試代碼,看 1748 剛才這個(gè)值對(duì)吧,它其實(shí)已經(jīng)得到了這個(gè)值。得到了值之后,我就要去設(shè)置到輸入的區(qū)域里面,這里你可以找到標(biāo)志性的內(nèi)容,發(fā)一條友善的評(píng)論,你還是去這里面搜,跟剛才類似,E。一條發(fā)一條友商的評(píng)論,也是在它附近把這個(gè)東西給復(fù)制出來(lái)。和剛才做法是類似的,把描述的內(nèi)容給改一下,把發(fā)一條友善的評(píng)論的元素設(shè)置為咳,因?yàn)槟憧赡懿皇悄敲醋匀弧N覜]試過(guò)設(shè)置成時(shí)間,什么可不可以,我估計(jì)可能也可以。這里剛開始試的時(shí)候,我直接把它寫成了它提供的值,你是需要理解的。如果你純從語(yǔ)義上可能也是可以的,把它設(shè)置,它就得到了這么一個(gè)東西。實(shí)際上它只是完成了這第二個(gè)步驟。第一個(gè)步驟沒有包含在這里面,所以合并一下上面的代碼,這里你可以看到和剛才代碼,加上這個(gè)代碼,有一句去掉了,是因?yàn)樗且粋€(gè)就是輸出,讓你在里面,沒有讓你在里面再輸出到看到值的一個(gè)語(yǔ)句,不是影響它的功能。下面這里就做到了。獲取到時(shí)間戳,設(shè)置到輸入框里面。但是一般的時(shí)候我不可能每次都這樣執(zhí)行一遍嗎?我牛哥可以給他設(shè)個(gè)快捷鍵,按 t 的時(shí)候執(zhí)行那些代碼,他又給他改了一下,這里和上面是一樣的。這里你其實(shí)不用管他怎么寫,反正寫出來(lái)了,這樣之后你按 t 就行了。執(zhí)行之后有一個(gè)問(wèn)題是,因?yàn)樗喈?dāng)于,我按了 t 之后,它可以把它設(shè)置到這里我想做的事情。比如現(xiàn)在這里他并沒有包含程序這個(gè)東西,是你講什么他就做什么,他不會(huì)去多余的去做一些事情。所以他只設(shè)置之后,你需要去把你的光標(biāo)或者鼠標(biāo)激活在這個(gè)位置。所以你再告訴他說(shuō)執(zhí)行代碼后激活內(nèi)容輸入的區(qū)域,就相當(dāng)于它會(huì)它會(huì)把讓你可以直接在這里輸入了,而不用你自己再手動(dòng)來(lái)激活這個(gè)地方。這里還有一個(gè)問(wèn)題是,這里說(shuō)的是 t 按 t 默認(rèn),它其實(shí)會(huì)把 t 也輸入到這里,這是一個(gè)網(wǎng)頁(yè)的默認(rèn)的行為,因?yàn)閷?shí)際上他這個(gè)事情是你在改寫網(wǎng)頁(yè)默認(rèn)的一些反應(yīng)行為,你其實(shí)不想讓他不想要默認(rèn)的行為,就按 t 的時(shí)候不是去輸入t,而是做一些事情。你就要寫一下取消網(wǎng)頁(yè)默認(rèn)的行為。我你其實(shí)不太需要去管它里面是怎么個(gè)意思,我這里就是為了說(shuō)明。我來(lái)講一下。其實(shí)加了這么這一句,就對(duì)應(yīng)了剛才這句話。加了默認(rèn)的行為。這個(gè)時(shí)候它生成的是這種,就在這里輸入的時(shí)候,直接在這個(gè)時(shí)間戳里輸入的,你可以再優(yōu)化一下時(shí)間,后面加個(gè)空格,這時(shí)候它就會(huì)變成空格,加了一個(gè)空格讓你再輸入。他也是改了一下,其實(shí)是這里加了一個(gè)這里的空格。按t。我其實(shí)我之前自己寫的代碼就是按 t 來(lái)加的,我都沒有,因?yàn)榉凑詈鬀]有改?,F(xiàn)在我可以再優(yōu)化一個(gè)代碼,就是按 shift t,這里他又加了一個(gè) shift key,就是按 t 的時(shí)候也按shift。讓執(zhí)行代碼。剛才激活的是 for focus,這是這一句大概能對(duì)應(yīng)出來(lái)。你看一下它的每次變化的東西,大概能對(duì)應(yīng)出來(lái)。這個(gè)功能還是比較簡(jiǎn)單的,就是這個(gè)代碼,其實(shí)你這樣這樣粘貼的,讓它執(zhí)行,你就以在這個(gè)頁(yè)面里就生效了。但一般情況下,這種很不方便。你可以安裝這么一個(gè)程序,它其實(shí)幫你做,讓你做這件事更加的方便。把這個(gè)東西安裝之后,就像剛才這里會(huì)出現(xiàn),你可以自己寫一個(gè),創(chuàng)建新的,把粘進(jìn)去,因?yàn)檫@個(gè)插件它本身有一些東西,有一些規(guī)則什么之類的,所以你這里要再改一下它。寫。改成這個(gè)東西叫什么 time monkey,他的名字就是插件名字的代碼,他就加了一些說(shuō)明的東西。這里有一個(gè)問(wèn)題,可能是稍微要明確的一點(diǎn),就了解一下它的功能。代碼其實(shí)不用你寫,但是你要知道它有哪些約定好的一些規(guī)則規(guī)范,他有哪些能力。實(shí)際上是對(duì)應(yīng)的說(shuō)明。這個(gè)意思是代碼在哪個(gè)頁(yè)面加載,我們實(shí)際上是想讓他在嗶哩嗶哩加載。這里要改一下,你不用改代碼,你我剛開始是只在加載它,其實(shí)最后生成的不太對(duì),因?yàn)樗皇沁@一個(gè)網(wǎng)頁(yè),就是首頁(yè),只是首頁(yè)。實(shí)際上你不想,你是想在這種我視頻播放頁(yè)讓它加載,你就再稍微改一下,在這是多輸了一個(gè),在他所有頁(yè)面加載這里他又改了,他又加了一個(gè)星號(hào)。這其實(shí)是一種匹配的一個(gè)所謂匹配的模式。這個(gè)也可以不用了解,你之后用的多的時(shí)候你就知道怎么樣來(lái)做這種說(shuō)明。主要是這種說(shuō)明這里的其實(shí)不用,我是為了說(shuō)明去解釋它,其實(shí)你平時(shí)也可以不解釋它,這里就生成了代碼。其實(shí)我直接把它拷貝到這里,這是剛才運(yùn)行的時(shí)候,我直接拷貝一模一樣的東西。在保存之后你就會(huì)出現(xiàn)出現(xiàn)這一個(gè)東西,這里綠色的時(shí)候開啟它就相當(dāng)于生效了,你把它關(guān)掉就是不生效了。這樣就可以做到你在按 shift t 的時(shí)候就可以有這種效果。這實(shí)際上你稍微學(xué)習(xí)一下就可以做到零基礎(chǔ)去真的是接近零基礎(chǔ)去實(shí)現(xiàn)。增強(qiáng)一個(gè)網(wǎng)頁(yè)的功能。在之前你很難做到這件事的,你幾乎不是說(shuō)很難,幾乎不可能。當(dāng)然這里邊你還是要了解一些比如插件的一些規(guī)則,或者是一些基本的東西,這個(gè)東西也是需要一定的學(xué)習(xí)的,不是說(shuō)也不是說(shuō)真的那么自由,因?yàn)樗吘惯€是要遵循一些程序的一些原則的。不管怎么樣,我舉這個(gè)例子也并不完全是功能本身,而是你有了這種能力之后,你可以比如你用哪個(gè)網(wǎng)站的時(shí)候,你發(fā)現(xiàn)這個(gè)地方,我想讓他這樣做。其實(shí)一定程度上你都可以去自己去實(shí)現(xiàn)這個(gè)東西。其他的 g p t 就相當(dāng)于是你專屬的程,給你配備了一個(gè)專屬的程序員。對(duì),剛才有一個(gè)地方我忘說(shuō)了,因?yàn)槲椰F(xiàn)在電腦用新版的代碼比較卡,所以我現(xiàn)在還用的嗶哩嗶哩舊版的代碼。你這個(gè)東西要生效,你還是要要選擇舊版的設(shè)置才能執(zhí)行相應(yīng)的代碼?,F(xiàn)在再回顧一下這個(gè)東西,主要你寫說(shuō)明就行,怎么樣學(xué)寫說(shuō)明里面代碼具體啥意思你也不用太理解。昨今天主要還是看了王淑瑞老師昨天發(fā)了一個(gè)視頻,他講的是一個(gè)用 chart g p t 寫且爬蟲的一個(gè)例子,也挺有啟發(fā)的。我之前是覺著他寫代碼的能力還是還是可能要在有一定的專業(yè)能力的基礎(chǔ)上,可能還要才行,但是他舉的例子相對(duì)來(lái)說(shuō)還是比較能夠讓零基礎(chǔ)的普通人也能做到。這件事,也是有一些限制的。而且我其實(shí)改改也,我說(shuō)修改了他一些描述。他有的地方是你要開這種剛才我打開發(fā)者工具,自己去找元素,實(shí)際上就像像我剛才這種劇,你就隨便復(fù)制,讓他自己去找。這種方式更加 0 基礎(chǔ)。你就不需要了解這些什么開發(fā)者的這些工具,啥東西,唯一一個(gè)個(gè)可能稍微需要了解的插件的工具,以及這個(gè)里面代碼怎么放在什么位置。相對(duì)來(lái)說(shuō)可能專業(yè)化強(qiáng)一點(diǎn),實(shí)際上也不是那么簡(jiǎn),也實(shí)際上也不是那么難。實(shí)際上你看著是代碼,實(shí)際上你把這個(gè)東西粘在某個(gè)地方而已,是和它內(nèi)容本身。你是通過(guò)這種 XGP 對(duì)話的形式實(shí)現(xiàn)的,它只是告訴你放在什么地方。有這么一個(gè)規(guī)則,和代碼本身沒有太大的關(guān)系。所以是真的是挺厲害的。就真的他,有些我還真的挺驚訝的,他有些他真的可以,真的可以。你看起來(lái)他好像真的理解了你在說(shuō)什么,他真的知道哪一步該改到哪一步,而且很準(zhǔn)確,而且跟不需要你去人文的干預(yù)。如果他需要1,就算是需要,因?yàn)槌绦蜻@個(gè)東西需要非常精準(zhǔn)的去寫的。如果你需要一點(diǎn)干預(yù),對(duì)于零基礎(chǔ)的人來(lái)說(shuō)就很困難了,因?yàn)槟憔退愀囊粋€(gè)字母,那個(gè)字母就很難。對(duì)于有基礎(chǔ)的人還好說(shuō),但是他竟然可以做到你不完全不用改代碼本身,只是讓你去增加一些描述,或者改寫一些描述。這個(gè)東西相對(duì)來(lái)說(shuō)還是很自然的一種方式,是挺神奇的。但是另外一個(gè)角度來(lái)講,其實(shí) XGP 這種生成代碼的能力,在我的理解來(lái)說(shuō),它還是一種翻譯。它并不是真的理解了這個(gè)代碼本身的含義,而是有一些人寫了一些描述,比如教程什么之類的,它就有自然語(yǔ)言的這種描述,加上代碼,它實(shí)際上它訓(xùn)練之后,讓自然語(yǔ)言和程序,甚至或者還有數(shù)學(xué)的一些知識(shí)有了這種對(duì)應(yīng)關(guān)系。它只是讓你通過(guò)一種自然語(yǔ)言去翻譯成了一個(gè)代碼的這種語(yǔ)言。所以一個(gè)你,其實(shí)嚴(yán)格來(lái)講,他還是不能做到所有的這種程序的事情,就是那種特別模式化的,比較簡(jiǎn)單的爬蟲,或者這種小小的功能的改動(dòng),它是可以的。另外一個(gè)用的比較廣泛的一些語(yǔ)言,比如這是個(gè)Javascript,網(wǎng)頁(yè)什么東西的用的比較多, Python 各種有各種,它的門檻相對(duì)低一些,用的人也比較多,所以這種它的效果就比較好。如果一些比較冷門的編程或者冷門的語(yǔ)言,它可能都不行,所以它還是受制于語(yǔ)調(diào)這個(gè)東西。回過(guò)頭來(lái),反正網(wǎng)頁(yè)是很大多數(shù)人都會(huì)用到的。這種功能還是挺有意思的。我可以再舉一個(gè)例子,來(lái)說(shuō)明一下這種功能。實(shí)際上,當(dāng)你使用一個(gè)網(wǎng)站的時(shí)候,剛才這個(gè)例子是把它寫在了這個(gè)地方,挺方便的,我其實(shí)用的是挺久的一個(gè)功能。你使用一個(gè)網(wǎng)站的時(shí)候,你有哪些想自定義的一些功能,想增強(qiáng)的一些功能,你就可以自己讓用它來(lái)寫一下。比如,這是飛書的網(wǎng)站,我是經(jīng)常用它來(lái)識(shí)別文稿或者字幕,提取出來(lái)關(guān)鍵詞,看一看這個(gè)東西的大概內(nèi)容。你看這里我經(jīng)常做的是,我想把關(guān)鍵詞粘在它對(duì)應(yīng)的視頻下面,我每次可能要這個(gè)還不能選,因?yàn)樗拇a還是禁止選擇了,我不能選,我就想我想直接讓他能不能我按一個(gè)鍵,讓他把它復(fù)制出來(lái)內(nèi)容?,F(xiàn)在其實(shí)我是做到了。這里也可以看到一個(gè)叫一鍵復(fù)制視頻標(biāo)簽,也是剛才一樣一個(gè),類似的一個(gè)東西,它做到的效果就是我按 c 的時(shí)候就復(fù)制出來(lái)了,你可以看到粘貼剪剪貼板里面就有這個(gè)東西,這就是相當(dāng)于我增強(qiáng)了它。網(wǎng)站功能和剛才那種使用的生成和使用的方式是類似的,也可以嘗試。我舉這個(gè)例子想說(shuō),當(dāng)你看到一個(gè)網(wǎng)站上有你想實(shí)現(xiàn)的一個(gè)小功能的是吧?可能大功能還是有點(diǎn)勉強(qiáng),這種增強(qiáng)性的。這種功能還是挺有挺有價(jià)值的,也比較容易來(lái)做。所以總結(jié)一下,真的有了 XGP 這個(gè)東西,很多一些小的功能,你真的可以自己來(lái)做,也不需要真正的去找一個(gè)董編程的程序員來(lái)幫你來(lái)做了。對(duì),今天視頻就分享到這里,我覺得最大的能力就是它讓零基礎(chǔ)的普通人,所有人都可以來(lái)做這件事情,不再局限于只有專業(yè)的程序員還要學(xué)半天才能去完成這么一件事情。雖然它現(xiàn)在可能還比較只能實(shí)現(xiàn)一些比較簡(jiǎn)單的功能,但是因?yàn)橛謩傆窒裎覄偛耪f(shuō)的,它是因?yàn)檎Z(yǔ)調(diào)的限制,我我我懷疑當(dāng)語(yǔ)料豐富之后,甚至都可以做到非常專業(yè)的這種事情還是挺厲害的一個(gè)功能,也不怪最近那么火,所以就這樣。有什么想法也都?xì)g迎來(lái)交流,看看能用它來(lái)做到一些之前完全想不到的事情。好,今天就這樣。