使用unity制作一個寶可夢——5.使用腳本對象創(chuàng)建寶可夢
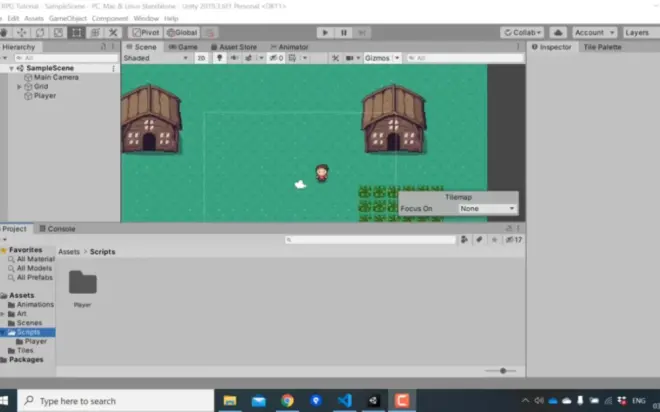
《PonkemonBase.cs》
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu(fileName = "Pokemon",menuName ="Pokemon/Create new pokemon")]
public class PokemonBase : ScriptableObject
{
??[SerializeField] string name;?//寶可夢的名字
??[TextArea]?
??[SerializeField] string description;?//描述
??[SerializeField] Sprite frontSprite;??//寶可夢的正面
??[SerializeField] Sprite backSprite;??//寶可夢的反面
??[SerializeField] PokemonType Type1;??//寶可夢的主系別
??[SerializeField] PokemonType Type2;??//寶可夢的副系別
???
??[SerializeField] int maxHp;?????//最大生命值
??[SerializeField] int attack;?????//攻擊力
??[SerializeField] int defense;?????//防御力
??[SerializeField]int spAttack;?????//魔法攻擊
??[SerializeField]int spDefense;?????//魔法防御
??[SerializeField]int speed;????????//速度值
??public string GetName()
??{
????return name;
??}
??public string Description
??{
????get { return description; }
??}
??public int MaxHp
??{
????get { return maxHp; }?
??}
??public int Attack?
??{
????get { return attack; }
??}
??public int SpAttack?
??{
????get { return spAttack; }
??}
??public int Defense?
??{
????get { return defense; }
??}
??public int SpDefense
??{
????get { return spDefense; }
??}
??public int Speed
??{
????get { return speed; }
??}
??public string Name
??{
????get { return name; }
??}
}
public enum PokemonType
{
??None,??????????//沒有屬性
??Normal,?????????//正常的
??Fire,??????????//火屬性
??Water,??????????//水屬性
??Electric,???????//電氣
??Grass,?????????//草系
??Ice,??????????//冰系
??Fighting,???????//戰(zhàn)斗系
??Poison,????????//毒系
??Ground,????????//土系
??Flying,????????//飛行系
??Psychic,???????//精神系
??Bug,?????????//蟲系
??Rock,??????????//石系
??Ghost,???????//鬼系
??Dragon????????//龍系
}
《Pokemon.cs》
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Pokemon
{
??PokemonBase _base;??//原始數(shù)據(jù)(父類)
??int level;??????//等級
??public Pokemon(PokemonBase pbase,int plevel)
??{
????_base = pbase;
????level = plevel;
??}
??public int Attack?//攻擊力隨等級成長的公式
??{
????get { return Mathf.FloorToInt((_base.Attack * level) / 100f) + 5; }
??}
??public int Defense?//防御力隨等級成長的公式
??{
????get { return Mathf.FloorToInt((_base.Defense * level) / 100f) + 5; }
??}
??public int SpAttack?//魔攻隨等級成長的公式
??{
????get { return Mathf.FloorToInt((_base.SpAttack * level) / 100f) + 5; }
??}
??public int SpDefense?//魔抗隨等級成長的公式
??{
????get { return Mathf.FloorToInt((_base.SpDefense * level) / 100f) + 5; }
??}
??public int Speed?//速度隨等級成長的公式
??{
????get { return Mathf.FloorToInt((_base.Speed * level) / 100f) + 5; }
??}
??public int MaxHp?//防御力隨等級成長的公式
??{
????get { return Mathf.FloorToInt((_base.MaxHp * level) / 100f) + 10; }
??}
}